Can someone please help correct my errors? This is the assignment: HERE IS MY CODE: Controller: package Controller; import Model.Model; import View.View; public class Controller
Can someone please help correct my errors? This is the assignment:
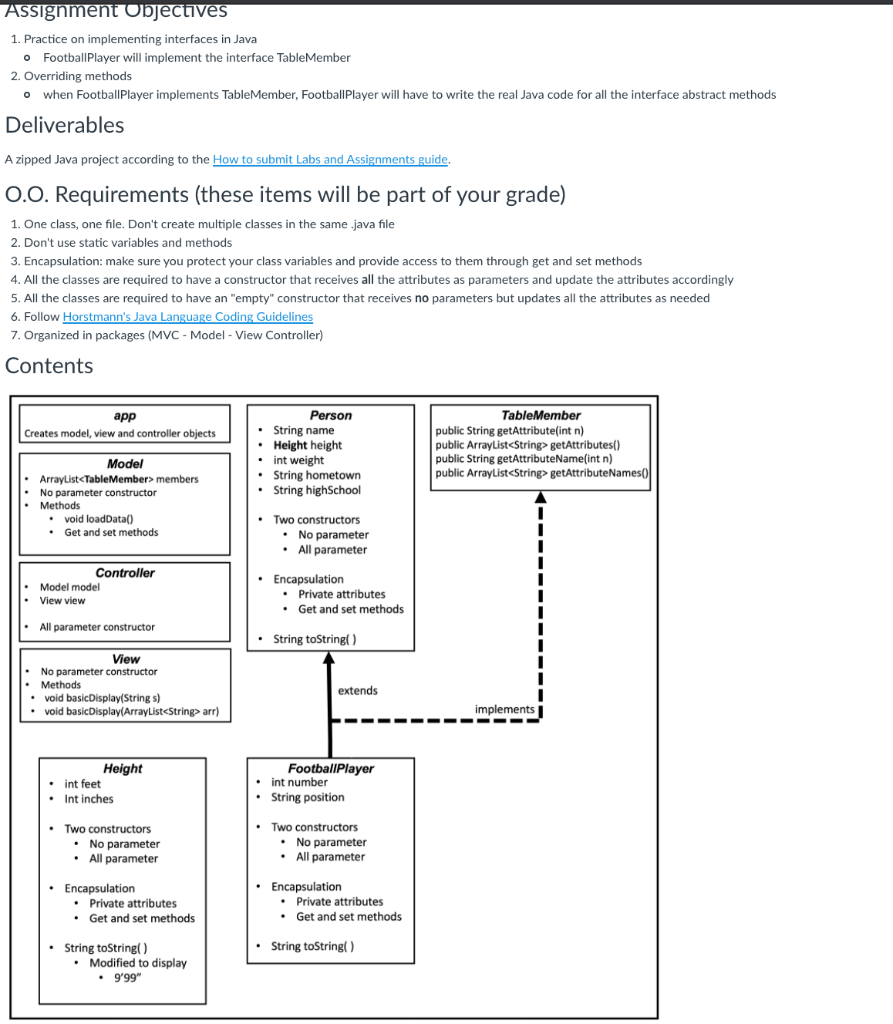
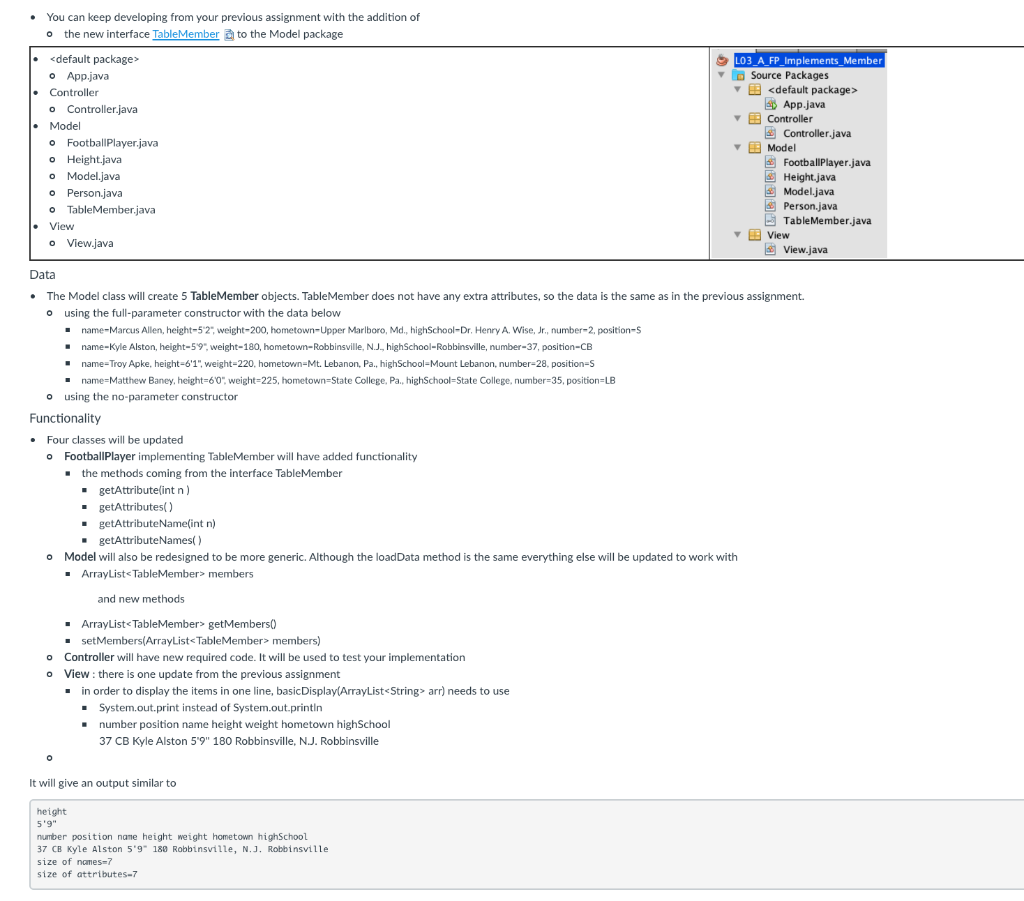
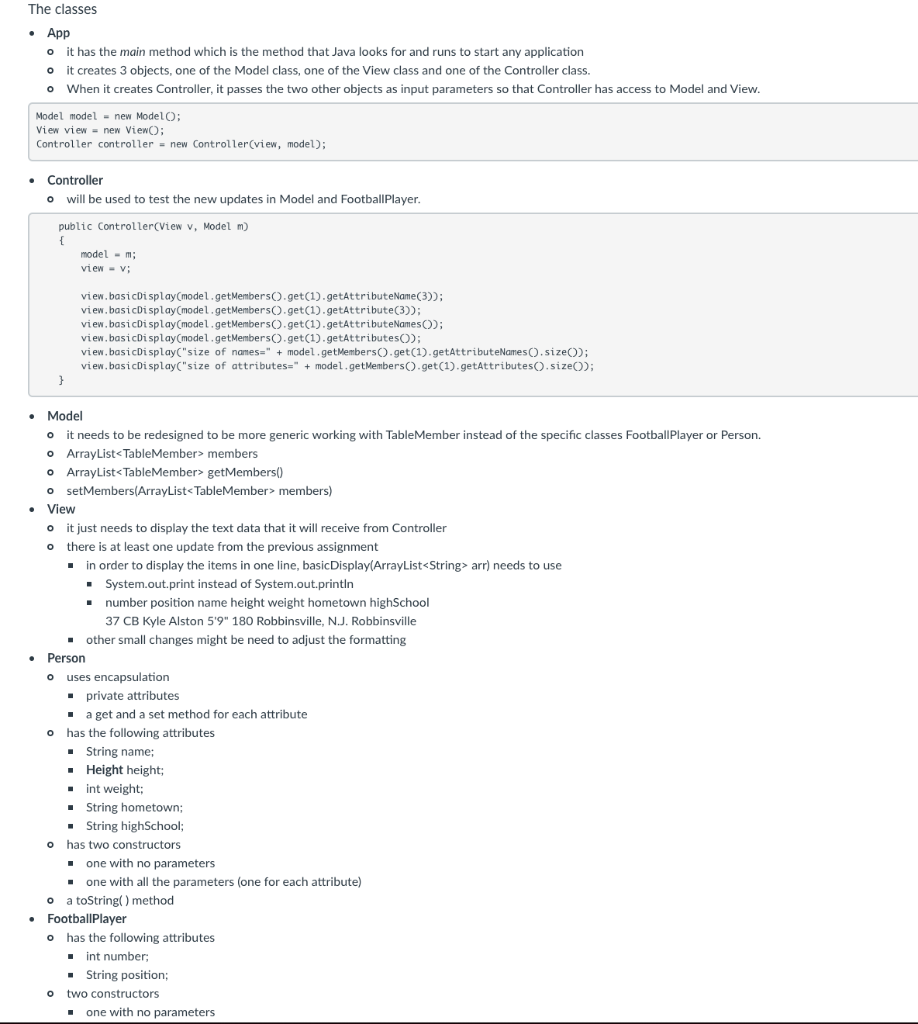
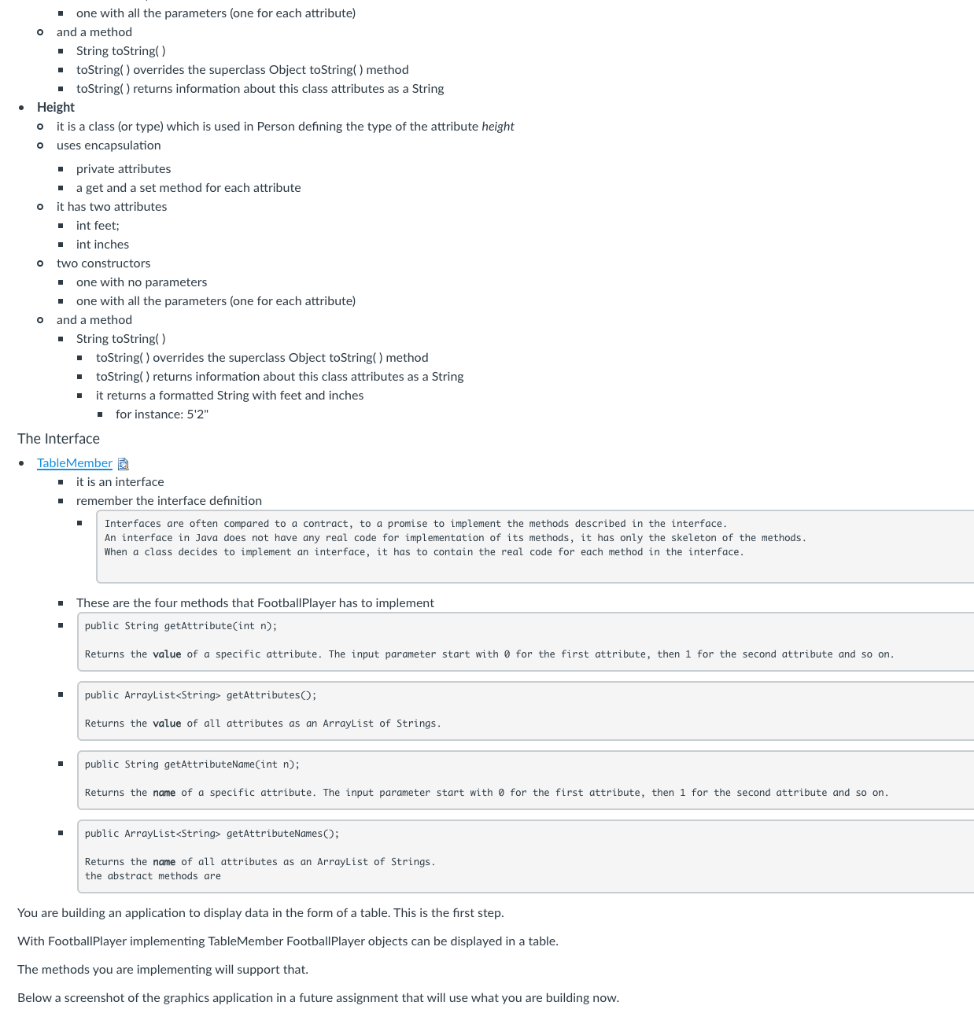
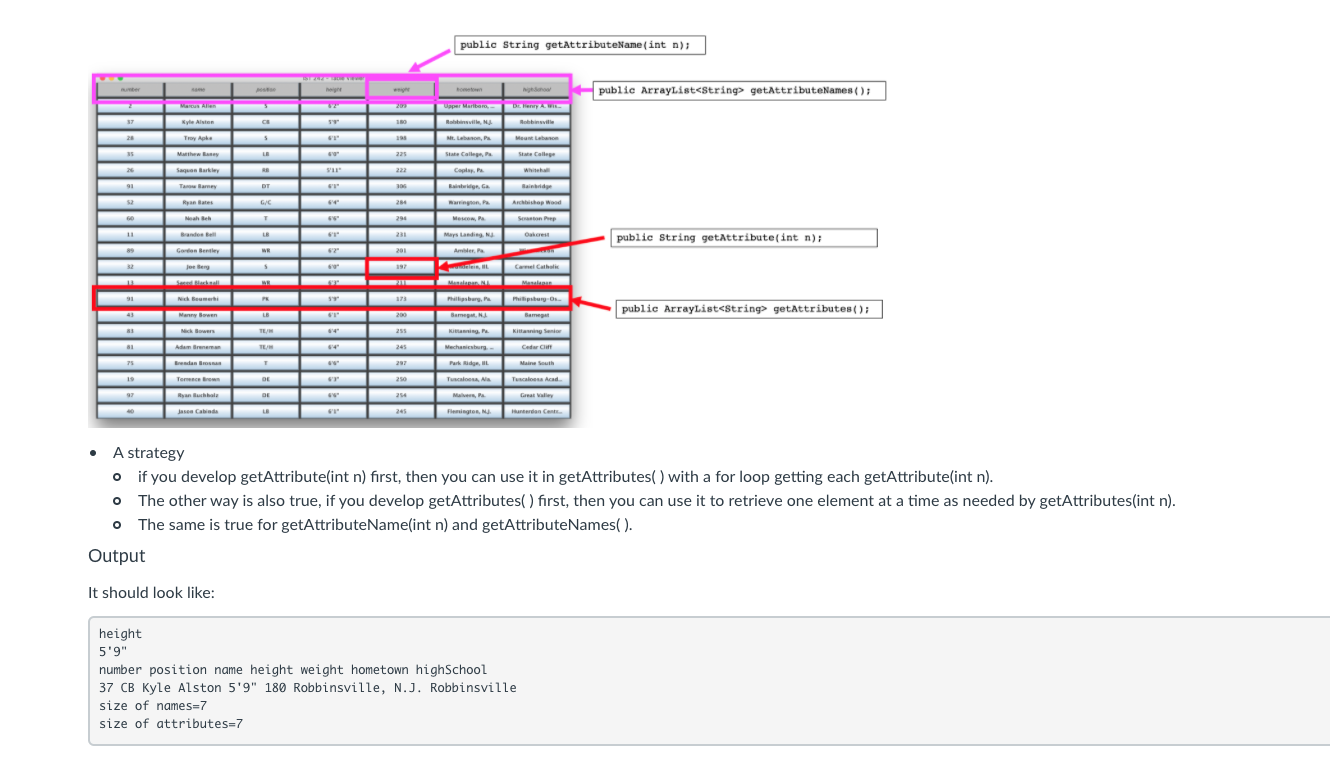
HERE IS MY CODE:
Controller:
package Controller;
import Model.Model; import View.View;
public class Controller { Model model; // Model object View view; // View object
public Controller(View v, Model m) { model = m; view = v;
view.basicDisplay(model.getMembers().get(1).getAttributeName(3)); view.basicDisplay(model.getMembers().get(1).getAttribute(3)); view.basicDisplay(model.getMembers().get(1).getAttributeNames()); view.basicDisplay(model.getMembers().get(1).getAttributes()); view.basicDisplay("size of names=" + model.getMembers().get(1).getAttributeNames().size()); view.basicDisplay("size of attributes=" + model.getMembers().get(1).getAttributes().size()); }
}
Model:
package Model;
import java.util.ArrayList;
public class Model {
private ArrayList members = new ArrayList();
public Model() { loadData(); }
public void loadData() { TableMember p1,p2,p3,p4,p5; p1 = new FootballPlayer ("Marcus Allen",5,2,200,"Upper Marlboro, Md.","Dr. Henry A. Wise, Jr.",2,"S"); p2 = new FootballPlayer ("Kyle Alston",5,9,180,"Robbinsville, N.J.","Robbinsville",37,"CB"); p3 = new FootballPlayer ("Troy Apke",6,1,220,"Mt. Lebanon, Pa.","Mount Lebanon", 28,"S"); p4 = new FootballPlayer ("Matthew Baney",6,0,225,"State College, Pa.","State College",35,"LB"); p5 = new FootballPlayer(); members.add(p1); members.add(p2); members.add(p3); members.add(p4); members.add(p5); } public String getData(int n) { switch (n) { case 0: return members.get(0).toString(); case 1: return members.get(1).toString(); case 2: return members.get(2).toString(); case 3: return members.get(3).toString(); case 4: return members.get(4).toString(); default: return ("invalid input parameter"); } } public ArrayList getData() { ArrayList arr = new ArrayList(); for(int i = 0; i public ArrayList getMembers() // Get method for private players ArrayList. { return members; }
public void setMembers(ArrayList members) // Set method for private players ArrayList. { this.members = members; } } View: package View;
import java.util.ArrayList;
public class View {
public void basicDisplay(String s) // Method that prints String s. { System.out.println(s); } public void basicDisplay(ArrayListarr) // Another basicDisplay method that overrides the first and outputs arr ArrayList. { for (int i = 0; i }
Person:
Package Model;
public class Person {
private String name; private Height height; private int weight; private String homeTown; private String highSchool;
public Person() { name=""; height=new Height(); weight=0; homeTown=""; highSchool=""; }
public Person(String n,Height h,int w,String ht,String hs) { name=n; height=h; weight=w; homeTown=ht; highSchool=hs; }
public String getName() { return name; } public Height getHeight() { return height; } public int getWeight() { return weight; } public String getHomeTown() { return homeTown; } public String getHighSchool() { return highSchool; } //Setters public void setName(String name) { this.name = name; } public void setHeight(Height height) { this.height = height; } public void setWeight(int weight) { this.weight = weight; } public void setHomeTown(String homeTown) { this.homeTown = homeTown; } public void setHighSchool(String highSchool) { this.highSchool = highSchool; } @Override public String toString() {
String str=""; str+=name+"\t"+height.toString()+"\t"+weight+"\t"+homeTown+"\t"+highSchool; return str; } }
Height:
package Model; public class Height {
int feet; int inches;
public Height() { feet=0; inches=0; }
public Height(int f,int in) { feet=f; inches=in; }
public int getFeet() { return feet; } public int getInch() { return inches; }
public void setFeet(int feet) { this.feet = feet; } public void setInch(int inches) { this.inches = inches; } @Override public String toString() {
return feet+"'"+inches+"''"; } } FootballPlayer:
package Model; import java.util.ArrayList;
public class FootballPlayer extends Person implements TableMember { private int number; private String position; //Default constructor public FootballPlayer() { super(); number=0; position=""; } public FootballPlayer(String n,Height h,int w,String ht,String hs,int num,String pos) { super(n,h,w,ht,hs); number=num; position=pos; }
FootballPlayer(String marcus_Allen, int i, int i0, int i1, String upper_Marlboro_Md, String dr_Henry_A_Wise_Jr, int i2, String s) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } //Getter public int getNumber() { return number; } public String getPosition() { return position; } //Setter public void setNumber(int number) { this.number = number; } public void setPosition(String position) { this.position = position; } @Override public String toString() { // TODO Auto-generated method stub return super.toString()+" \t"+number+" \t"+position+" "; } @Override public String getAttribute(int n) { ArrayList lst=getAttributes() ; return lst.get(n); } @Override public ArrayList getAttributes() { Model m=new Model(); m.loadData(); ArrayListtm=m.getMembers(); ArrayList lst=new ArrayList(); for(int i=0;i } @Override public String getAttributeName(int n) { ArrayList lst=getAttributeNames() ; return lst.get(n); } @Override public ArrayList getAttributeNames() { Model m=new Model(); m.loadData(); ArrayListtm=m.getMembers(); ArrayList lst=new ArrayList(); for(int i=0;i TeamMember:
package Model;
import java.util.ArrayList;
public interface TableMember { public String getAttribute(int n); public ArrayList getAttributes(); public String getAttributeName(int n); public ArrayList getAttributeNames(); }
App:
package App;
import Controller.Controller; import Model.Model; import View.View; public class App {
public static void main(String[] args) { Model model=new Model(); View view=new View(); model.loadData(); Controller controller = new Controller(view,model); } }
Here are my professor's comments:
1-FootballPlayer has everything it needs to solve the 4 new methods. It doesn't need "Model". My suggestion is: Start with getAttributeNames() -it needs to return an ArrayList of Strings. By requirement the 7 strings are number position name height weight hometown highSchool See what you did in the last lab for getData(). It is not the same but it is a start. 2-FootballPlayer has a constructor that is not being used and it is also crashing the application.
Can someone please explain? Thank you
Assignment Objectives 1. Practice on implementing interfaces in Java o Football Player will implement the interface Table Member 2. Overriding methods o when Football Player implements TableMember, Football Player will have to write the real Java code for all the interface abstract methods Deliverables A zipped Java project according to the How to submit Labs and Assignments guide, 0.0. Requirements (these items will be part of your grade) 1. One class, one file. Don't create multiple classes in the same.java file 2. Don't use static variables and methods 3. Encapsulation: make sure you protect your class variables and provide access to them through get and set methods 4. All the classes are required to have a constructor that receives all the attributes as parameters and update the attributes accordingly 5. All the classes are required to have an "empty" constructor that receives no parameters but updates all the attributes as needed 6. Follow Horstmann's Java Language Coding Guidelines 7. Organized in packages (MVC - Model - View Controller) Contents app Creates model, view and controller objects Person String name Height height int weight String hometown String highSchool Table Member public String getAttribute(int n) public ArrayListgetAttributes() public String getAttributeName(int n) public ArrayListgetAttributeNames Model ArrayList members No parameter constructor Methods void loadData() Get and set methods Two constructors No parameter All parameter Controller Model model View view Encapsulation Private attributes Get and set methods All parameter constructor String toString() View No parameter constructor Methods void basicDisplay/Strings) void basicDisplay(ArrayList arr) extends implements Height int feet Int inches Football Player int number String position Two constructors No parameter All parameter Two constructors No parameter All parameter Encapsulation Private attributes Get and set methods Encapsulation Private attributes Get and set methods String toString() String toString() Modified to display 999" You can keep developing from your previous assignment with the addition of o the new interface Table Member a to the Model package App.java E Controller Controller.java Model FootballPlayer.java Height.java Model.java Person.java TableMember.java View View.java Data The Model class will create 5 TableMember objects. TableMember does not have any extra attributes, so the data is the same as in the previous assignment. o using the full-parameter constructor with the data below name-Marcus Allen, height-52", weight-200, hometown-Upper Marlboro, Md., highSchool-Dr. Henry A. Wise, Jr., number-2, positions name-Kyle Alston, height-59", weight-180, hometown-Robbinsville, NJ., highSchool-Robbinsville, number-37, position-CB name=Troy Apke, height=6'1", weight-220, hometown Mt. Lebanon, Pa., highSchool-Mount Lebanon, number=28. position=s name=Matthew Baney, height=60", weight=225, hometown=State College, Pa., highSchool=State College, number=35, position=LB o using the no-parameter constructor Functionality Four classes will be updated o FootballPlayer implementing TableMember will have added functionality . the methods coming from the interface TableMember .getAttribute(int n) getAttributes() .getAttributeName(int n) getAttributeNames() Model will also be redesigned to be more generic. Although the loadData method is the same everything else will be updated to work with ArrayList members and new methods ArrayList getMembers() . setMembers(ArrayList members) o Controller will have new required code. It will be used to test your implementation o View : there is one update from the previous assignment in order to display the items in one line, basicDisplay(ArrayList arr) needs to use System.out.print instead of System.out.println number position name height weight hometown highSchool 37 CB Kyle Alston 5'9" 180 Robbinsville, NJ. Robbinsville It will give an output similar to height 5'9" number position name height weight hometown highSchool 37 CB Kyle Alston 5'9" 180 Robbinsville, N.J. Robbinsville size of names=7 size of attributes-7 The classes . App o it has the main method which is the method that Java looks for and runs to start any application it creates 3 objects, one of the Model class, one of the View class and one of the Controller class. o When it creates Controller, it passes the two other objects as input parameters so that Controller has access to Model and View. o Model model = new Model: View view = new ViewO; Controller controller = new Controller(view, model); Controller o will be used to test the new updates in Model and Football Player. public Controller(View v, Model m) model - m; view - V; view.basicDisplay (model.getMembers.get(1).getAttributeName(3)); view.basicDisplay (model.getMembers.get(1).getAttribute(3)); view.basicDisplay(model.getMembers.get(1).getAttributeNames); view.basicDisplay(model.getMembers.get(1).getAttributes(); view.basicDisplay("size of names=" + model.getMembers.get(1).getAttributeNames().size(); view.basicDisplay("size of attributes=" + model.getMembers().get(1).getAttributes().size(); } o Model o it needs to be redesigned to be more generic working with TableMember instead of the specific classes FootballPlayer or Person o ArrayList members o ArrayList getMembers() o setMembers(ArrayList members) View o it just needs to display the text data that it will receive from Controller o there is at least one update from the previous assignment in order to display the items in one line, basicDisplay(ArrayList arr) needs to use System.out.print instead of System.out.println number position name height weight hometown highSchool 37 CB Kyle Alston 5'9" 180 Robbinsville, NJ. Robbinsville other small changes might be need to adjust the formatting Person uses encapsulation private attributes a get and a set method for each attribute O has the following attributes String name: Height height; . int weight: String hometown; String highSchool; o has two constructors one with no parameters one with all the parameters (one for each attribute) o a toString() method Football Player o has the following attributes int number: String position; O two constructors one with no parameters . one with all the parameters (one for each attribute) o and a method . String toString() .toString() overrides the superclass Object toString() method toString() returns information about this class attributes as a String Height o it is a class (or type) which is used in Person defining the type of the attribute height o uses encapsulation . private attributes a get and a set method for each attribute o it has two attributes . int feet; int inches o two constructors one with no parameters one with all the parameters (one for each attribute) o and a method . String toString() toString() overrides the superclass Object toString() method toString() returns information about this class attributes as a String it returns a formatted String with feet and inches for instance: 5'2" The Interface TableMember it is an interface remember the interface definition Interfaces are often compared to a contract, to a promise to implement the methods described in the interface. An interface in Java does not have any real code for implementation of its methods, it has only the skeleton of the methods. When a class decides to implement an interface, it has to contain the real code for each method in the interface. These are the four methods that Football Player has to implement public String getAttribute(int n); Returns the value of a specific attribute. The input parameter start with for the first attribute, then 1 for the second attribute and so on. public ArrayList getAttributes; Returns the value of all attributes as an ArrayList of Strings. public String getAttributeName(int n); Returns the name of a specific attribute. The input parameter start with for the first attribute, then 1 for the second attribute and so on. public ArrayList getAttributeNamesO; Returns the name of all attributes as an ArrayList of Strings. the abstract methods are You are building an application to display data in the form of a table. This is the first step. With Football Player implementing TableMember Football Player objects can be displayed in a table. The methods you are implementing will support that. Below a screenshot of the graphics application in a future assignment that will use what you are building now. public String getAttributeName(int n); public ArrayList getAttributeNames(); ST Kyle Alte Troy Aske Robbinsville, Robinie Nt. Laban, Mount Lan State College State College Copi. Pe 225 Saguen Barkley S'HI Tamame DT Bainbridge Ahop Wood 52 Ryanes GC T Mesto Son Pep Brande Bell Mays Landing, Calcrest public String getAttribute(int n); Gordon Bentley 32 Joe Berg . IRL Carmel Catholic Nicho Phillipsburg, Philipsbury - public ArrayList getAttributes(); Manny Bowen Bamagat 255 Bumage King, Mechanicsburg- Park Ridge, Adarne Cedar CT Torce Brown DE TA Tuscaloosa Acad DE Jasa Cala Mandarden Cente A strategy o if you develop getAttribute(int n) first, then you can use it in getAttributes() with a for loop getting each getAttribute(int n). The other way is also true, if you develop getAttributes() first, then you can use it to retrieve one element at a time as needed by getAttributes(int n). The same is true for getAttributeName(int n) and getAttributeName(). Output o It should look like: height 5'9" number position name height weight hometown highSchool 37 CB Kyle Alston 5'9" 180 Robbinsville, N.J. Robbinsville size of names=7 size of attributes=7 Assignment Objectives 1. Practice on implementing interfaces in Java o Football Player will implement the interface Table Member 2. Overriding methods o when Football Player implements TableMember, Football Player will have to write the real Java code for all the interface abstract methods Deliverables A zipped Java project according to the How to submit Labs and Assignments guide, 0.0. Requirements (these items will be part of your grade) 1. One class, one file. Don't create multiple classes in the same.java file 2. Don't use static variables and methods 3. Encapsulation: make sure you protect your class variables and provide access to them through get and set methods 4. All the classes are required to have a constructor that receives all the attributes as parameters and update the attributes accordingly 5. All the classes are required to have an "empty" constructor that receives no parameters but updates all the attributes as needed 6. Follow Horstmann's Java Language Coding Guidelines 7. Organized in packages (MVC - Model - View Controller) Contents app Creates model, view and controller objects Person String name Height height int weight String hometown String highSchool Table Member public String getAttribute(int n) public ArrayListgetAttributes() public String getAttributeName(int n) public ArrayListgetAttributeNames Model ArrayList members No parameter constructor Methods void loadData() Get and set methods Two constructors No parameter All parameter Controller Model model View view Encapsulation Private attributes Get and set methods All parameter constructor String toString() View No parameter constructor Methods void basicDisplay/Strings) void basicDisplay(ArrayList arr) extends implements Height int feet Int inches Football Player int number String position Two constructors No parameter All parameter Two constructors No parameter All parameter Encapsulation Private attributes Get and set methods Encapsulation Private attributes Get and set methods String toString() String toString() Modified to display 999" You can keep developing from your previous assignment with the addition of o the new interface Table Member a to the Model package App.java E Controller Controller.java Model FootballPlayer.java Height.java Model.java Person.java TableMember.java View View.java Data The Model class will create 5 TableMember objects. TableMember does not have any extra attributes, so the data is the same as in the previous assignment. o using the full-parameter constructor with the data below name-Marcus Allen, height-52", weight-200, hometown-Upper Marlboro, Md., highSchool-Dr. Henry A. Wise, Jr., number-2, positions name-Kyle Alston, height-59", weight-180, hometown-Robbinsville, NJ., highSchool-Robbinsville, number-37, position-CB name=Troy Apke, height=6'1", weight-220, hometown Mt. Lebanon, Pa., highSchool-Mount Lebanon, number=28. position=s name=Matthew Baney, height=60", weight=225, hometown=State College, Pa., highSchool=State College, number=35, position=LB o using the no-parameter constructor Functionality Four classes will be updated o FootballPlayer implementing TableMember will have added functionality . the methods coming from the interface TableMember .getAttribute(int n) getAttributes() .getAttributeName(int n) getAttributeNames() Model will also be redesigned to be more generic. Although the loadData method is the same everything else will be updated to work with ArrayList members and new methods ArrayList getMembers() . setMembers(ArrayList members) o Controller will have new required code. It will be used to test your implementation o View : there is one update from the previous assignment in order to display the items in one line, basicDisplay(ArrayList arr) needs to use System.out.print instead of System.out.println number position name height weight hometown highSchool 37 CB Kyle Alston 5'9" 180 Robbinsville, NJ. Robbinsville It will give an output similar to height 5'9" number position name height weight hometown highSchool 37 CB Kyle Alston 5'9" 180 Robbinsville, N.J. Robbinsville size of names=7 size of attributes-7 The classes . App o it has the main method which is the method that Java looks for and runs to start any application it creates 3 objects, one of the Model class, one of the View class and one of the Controller class. o When it creates Controller, it passes the two other objects as input parameters so that Controller has access to Model and View. o Model model = new Model: View view = new ViewO; Controller controller = new Controller(view, model); Controller o will be used to test the new updates in Model and Football Player. public Controller(View v, Model m) model - m; view - V; view.basicDisplay (model.getMembers.get(1).getAttributeName(3)); view.basicDisplay (model.getMembers.get(1).getAttribute(3)); view.basicDisplay(model.getMembers.get(1).getAttributeNames); view.basicDisplay(model.getMembers.get(1).getAttributes(); view.basicDisplay("size of names=" + model.getMembers.get(1).getAttributeNames().size(); view.basicDisplay("size of attributes=" + model.getMembers().get(1).getAttributes().size(); } o Model o it needs to be redesigned to be more generic working with TableMember instead of the specific classes FootballPlayer or Person o ArrayList members o ArrayList getMembers() o setMembers(ArrayList members) View o it just needs to display the text data that it will receive from Controller o there is at least one update from the previous assignment in order to display the items in one line, basicDisplay(ArrayList arr) needs to use System.out.print instead of System.out.println number position name height weight hometown highSchool 37 CB Kyle Alston 5'9" 180 Robbinsville, NJ. Robbinsville other small changes might be need to adjust the formatting Person uses encapsulation private attributes a get and a set method for each attribute O has the following attributes String name: Height height; . int weight: String hometown; String highSchool; o has two constructors one with no parameters one with all the parameters (one for each attribute) o a toString() method Football Player o has the following attributes int number: String position; O two constructors one with no parameters . one with all the parameters (one for each attribute) o and a method . String toString() .toString() overrides the superclass Object toString() method toString() returns information about this class attributes as a String Height o it is a class (or type) which is used in Person defining the type of the attribute height o uses encapsulation . private attributes a get and a set method for each attribute o it has two attributes . int feet; int inches o two constructors one with no parameters one with all the parameters (one for each attribute) o and a method . String toString() toString() overrides the superclass Object toString() method toString() returns information about this class attributes as a String it returns a formatted String with feet and inches for instance: 5'2" The Interface TableMember it is an interface remember the interface definition Interfaces are often compared to a contract, to a promise to implement the methods described in the interface. An interface in Java does not have any real code for implementation of its methods, it has only the skeleton of the methods. When a class decides to implement an interface, it has to contain the real code for each method in the interface. These are the four methods that Football Player has to implement public String getAttribute(int n); Returns the value of a specific attribute. The input parameter start with for the first attribute, then 1 for the second attribute and so on. public ArrayList getAttributes; Returns the value of all attributes as an ArrayList of Strings. public String getAttributeName(int n); Returns the name of a specific attribute. The input parameter start with for the first attribute, then 1 for the second attribute and so on. public ArrayList getAttributeNamesO; Returns the name of all attributes as an ArrayList of Strings. the abstract methods are You are building an application to display data in the form of a table. This is the first step. With Football Player implementing TableMember Football Player objects can be displayed in a table. The methods you are implementing will support that. Below a screenshot of the graphics application in a future assignment that will use what you are building now. public String getAttributeName(int n); public ArrayList getAttributeNames(); ST Kyle Alte Troy Aske Robbinsville, Robinie Nt. Laban, Mount Lan State College State College Copi. Pe 225 Saguen Barkley S'HI Tamame DT Bainbridge Ahop Wood 52 Ryanes GC T Mesto Son Pep Brande Bell Mays Landing, Calcrest public String getAttribute(int n); Gordon Bentley 32 Joe Berg . IRL Carmel Catholic Nicho Phillipsburg, Philipsbury - public ArrayList getAttributes(); Manny Bowen Bamagat 255 Bumage King, Mechanicsburg- Park Ridge, Adarne Cedar CT Torce Brown DE TA Tuscaloosa Acad DE Jasa Cala Mandarden Cente A strategy o if you develop getAttribute(int n) first, then you can use it in getAttributes() with a for loop getting each getAttribute(int n). The other way is also true, if you develop getAttributes() first, then you can use it to retrieve one element at a time as needed by getAttributes(int n). The same is true for getAttributeName(int n) and getAttributeName(). Output o It should look like: height 5'9" number position name height weight hometown highSchool 37 CB Kyle Alston 5'9" 180 Robbinsville, N.J. Robbinsville size of names=7 size of attributes=7