Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Can someone please help me fix my code? I've been struggling to get it to run and I don't know where I went wrong. I
Can someone please help me fix my code? I've been struggling to get it to run and I don't know where I went wrong.
I have tried everything and I'm about to give up!
there are 3 classes:
1.
Main.java
import java.util.ArrayList; class Main { public static ArrayListtestHand1() { ArrayList h1 = new ArrayList (); h1.add("A of Hearts"); h1.add("K of Hearts"); h1.add("Q of Hearts"); h1.add("J of Hearts"); h1.add("10 of Hearts"); return h1; } public static ArrayList testHand2() { ArrayList h1 = new ArrayList (); h1.add("9 of Hearts"); h1.add("K of Hearts"); h1.add("Q of Hearts"); h1.add("J of Hearts"); h1.add("10 of Hearts"); return h1; } public static ArrayList testHand3() { ArrayList h1 = new ArrayList (); h1.add("9 of Hearts"); h1.add("9 of Spades"); h1.add("9 of Clubs"); h1.add("9 of Diamonds"); h1.add("2 of Hearts"); return h1; } public static ArrayList testHand4() { ArrayList h1 = new ArrayList (); h1.add("9 of Hearts"); h1.add("9 of Spades"); h1.add("2 of Clubs"); h1.add("2 of Diamonds"); h1.add("2 of Hearts"); return h1; } public static ArrayList testHand5() { ArrayList h1 = new ArrayList (); h1.add("2 of Hearts"); h1.add("9 of Hearts"); h1.add("A of Hearts"); h1.add("3 of Hearts"); h1.add("7 of Hearts"); return h1; } public static ArrayList testHand6() { ArrayList h1 = new ArrayList (); h1.add("5 of Hearts"); h1.add("8 of Clubs"); h1.add("9 of Diamonds"); h1.add("7 of Spades"); h1.add("6 of Hearts"); return h1; } public static ArrayList testHand7() { ArrayList h1 = new ArrayList (); h1.add("A of Hearts"); h1.add("A of Clubs"); h1.add("A of Spades"); h1.add("3 of Hearts"); h1.add("7 of Hearts"); return h1; } public static ArrayList testHand8() { ArrayList h1 = new ArrayList (); h1.add("A of Hearts"); h1.add("A of Clubs"); h1.add("7 of Spades"); h1.add("3 of Hearts"); h1.add("7 of Hearts"); return h1; } public static ArrayList testHand9() { ArrayList h1 = new ArrayList (); h1.add("A of Hearts"); h1.add("A of Clubs"); h1.add("7 of Spades"); h1.add("3 of Hearts"); h1.add("K of Hearts"); return h1; } public static ArrayList testHand10() { ArrayList h1 = new ArrayList (); h1.add("A of Hearts"); h1.add("4 of Clubs"); h1.add("7 of Spades"); h1.add("3 of Hearts"); h1.add("K of Hearts"); return h1; } public static void main(String[] args) { ArrayList royal = testHand1(); ArrayList straightFlush= testHand2(); ArrayList fourKind= testHand3(); ArrayList fullHouse= testHand4(); ArrayList flush= testHand5(); ArrayList straight= testHand6(); ArrayList threeKind= testHand7(); ArrayList twoPair= testHand8(); ArrayList onePair= testHand9(); ArrayList highCard= testHand10(); System.out.println(); Poker gameOne = new Poker(royal, straightFlush); gameOne.showHand(1); System.out.println(gameOne.scoreHand(1)); gameOne.showHand(2); System.out.println(gameOne.scoreHand(2)); System.out.println(); Poker gameTwo=new Poker(fourKind,fullHouse); gameTwo.showHand(1); System.out.println(gameTwo.scoreHand(1)); gameTwo.showHand(2); System.out.println(gameTwo.scoreHand(2)); System.out.println(); Poker gameThree=new Poker(flush,straight); gameThree.showHand(1); System.out.println(gameThree.scoreHand(1)); gameThree.showHand(2); System.out.println(gameThree.scoreHand(2)); System.out.println(); Poker gameFour=new Poker(threeKind,twoPair); gameFour.showHand(1); System.out.println(gameFour.scoreHand(1)); gameFour.showHand(2); System.out.println(gameFour.scoreHand(2)); System.out.println(); Poker gameFive=new Poker(onePair,highCard); gameFive.showHand(1); System.out.println(gameFive.scoreHand(1)); gameFive.showHand(2); System.out.println(gameFive.scoreHand(2)); System.out.println(); Poker gameSix=new Poker(royal, straightFlush); gameSix.showHand(1); System.out.println(gameSix.scoreHand(1)); gameSix.showHand(2); System.out.println(gameSix.scoreHand(2)); } }
2.
PlayingCards.java
import java.util.ArrayList; class PlayingCards { private ArrayListdeck = new ArrayList (); public PlayingCards() { String[] suite = new String[] {"Clubs","Diamonds","Hearts","Spades"}; String[] values= new String[] {"A","2","3","4","5","6","7","8","9","10","J","Q","K"}; for(int i=0;i<4;i++) { for(int j=0;j<13;j++) { String newCard=values[j]+" of "+suite[i]; deck.add(newCard); } } } public void Shuffle() { Random myRand=new Random(); int numShuffles=myRand.nextInt(500); int numCards=deck.size(); for(int i=0;i 0) { nc=deck.get(0); deck.remove(0); } else { nc="Deck is empty"; } return nc; } }
3.
Poker.java
import java.util.ArrayList; class Poker { private PlayingCards deck; private ArrayListhand1; private ArrayList hand2; public Poker(){ deck = new PlayingCards(); hand1 = new ArrayList (); hand2 = new ArrayList (); deck.shuffle(); dealHands(); } // constructor that takes in two Lists public Poker(List h1, List h2) { deck = new PlayingCards(); hand1 = h1; hand2 = h2; } public void dealHands() { for(int i = 0; i < 5; i++) { hand1.add(deck.draw()); hand2.add(deck.draw()); } } // method to show a hand public void showHand(int playerNum) { if (playerNum == 1) { Console.WriteLine("Player 1's hand:"); foreach (string card in hand1) { Console.WriteLine(card); } Console.WriteLine(); } else { Console.WriteLine("Player 2's hand:"); foreach (string card in hand2) { Console.WriteLine(card); } } } // method to count suite in a hand public int[] countSuite(List hand) { int[] suiteCount = new int[4]; foreach (string card in hand) { string suite = card.Split(' ')[1]; if (suite == "Clubs"){ suiteCount[0]++; } else if (suite == "Diamonds") { suiteCount[1]++; } else if (suite == "Hearts") { suiteCount[2]++; } else if (suite == "Spades") { suiteCount[3]++; } } return suiteCount; } public static int[] countValues(ArrayList hand) { int[] values = new int[14]; for (String card : hand) { char value = card.charAt(0); if (value == 'A') values[1]++; else if (value == '2') values[2]++; else if (value == '3') values[3]++; else if (value == '4') values[4]++; else if (value == '5') values[5]++; else if (value == '6') values[6]++; else if (value == '7') values[7]++; else if (value == '8') values[8]++; else if (value == '9') values[9]++; else if (value == 'T') values[10]++; else if (value == 'J') values[11]++; else if (value == 'Q') values[12]++; else if (value == 'K') values[13]++; } return values; } public static int numPairs(int[] values) { int pairs = 0; for (int value : values) { if (value == 2) pairs++; } return pairs; } public static int threeOfAKind(int[] values) { for (int i = 1; i < values.length; i++) { if (values[i] == 3) return i; } return 0; } public static int fourOfAKind(int[] values) { for (int i = 1; i < values.length; i++) { if (values[i] == 4) return i; } return 0; } public static boolean fullHouse(int[] values) { int three = threeOfAKind(values); int pairs = numPairs(values); if (three > 0 && pairs > 1) return true; return false; } public static boolean straight(int[] values) { boolean straight = false; for (int i = 1; i < values.length - 4; i++) { if (values[i] == 1 && values[i + 1] == 1 && values[i + 2] == 1 && values[i + 3] == 1 && values[i + 4] == 1) { straight = true; break; } } if (!straight && values[10] == 1 && values[11] == 1 && values[12] == 1 && values[13] == 1 && values[1] == 1) { straight = true; } return straight; } public static boolean flush(int clubs, int diamonds, int hearts, int spades) { if (clubs == 5 || diamonds == 5 || hearts == 5 || spades == 5) return true; return false; } void straightFlush(),royalFlush(): public boolean straightFlush(int[] countValues, int clubs, int diamonds, int hearts, int spades) { if (flush(clubs, diamonds, hearts, spades) && straight(countValues)) { return true; } return false; } public boolean royalFlush(int[] countValues, int clubs, int diamonds, int hearts, int spades) { if ((clubs == 5 || diamonds == 5 || hearts == 5 || spades == 5) && countValues[10] == 1 && countValues[11] == 1 && countValues[12] == 1 && countValues[13] == 1 && countValues[1] == 1) { return true; } return false; } scoreHand() public String scoreHand(int handNumber, int[] countValues, int clubs, int diamonds, int hearts, int spades) { if (royalFlush(countValues, clubs, diamonds, hearts, spades)) { return "Royal Flush"; } else if (straightFlush(countValues, clubs, diamonds, hearts, spades)) { return "Straight Flush"; } else if (fourOfAKind(countValues) != 0) { return "Four of a Kind"; } else if (fullHouse(countValues)) { return "Full House"; } else if (flush(clubs, diamonds, hearts, spades)) { return "Flush"; } else if (straight(countValues)) { return "Straight"; } else if (threeOfAKind(countValues) != 0) { return "Three of a Kind"; } else if (numPairs(countValues) == 2) { return "Two Pairs"; } else if (numPairs(countValues) == 1) { return "One Pair"; } else { return "High Card"; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
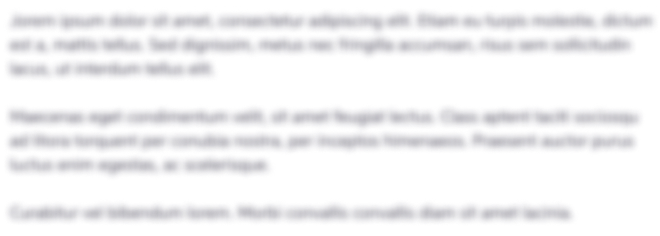
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started