Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Can you create code for the assignment below: Black background with two identical white triangles. Each triangle has bottom corner cut off. The above image
Can you create code for the assignment below:
Black background with two identical white triangles. Each triangle has bottom corner cut off.
The above image shows two four
sided pyramids. The goal of this assignment is to change the transformation code in the SceneManager::RenderScene
method for each pyramid to make the two pyramids match the following picture:
A white triangle inverted above another white triangle so their tips meet form an hourglass shape.
Specifically, you must address the following rubric criteria:
Create code to address the required functionality. The code you add must meet the required functionality and visual representation outlined for this assignment. This result may require multiple attempts and strategies to get it right, but that is okay! Working in stages is an important part of any coding project.
Apply logic and proper syntax to code. Source code should be free of logical or syntax errors that prevent the application from running as expected. You will be given credit for code that is set up to meet specifications or solve the problem.
Apply commenting and formatting standards to facilitate understanding of the code. All code should be well commented. Commenting is a practiced art. Your comments should be as clear and brief as possible. Your comments should explain the purpose of lines or sections of the code. You may also include the method you used to achieve a specific task in the code. Be sure to document any sections of code that produce errors or incorrect results. Organize all code to meet formatting standards.
SceneManager.cpp
scenemanager.h
manage the preparing and rendering of D scenes textures, materials, lighting
#pragma once
#include "ShaderManager.h
#include "ShapeMeshes.h
#include
#include
SceneManager
This class contains the code for preparing and rendering
D scenes, including the shader settings.
class SceneManager
public:
constructor
SceneManagerShaderManager pShaderManager;
destructor
~SceneManager;
properties for loaded texture access
struct TEXTUREINFO
std::string tag;
uintt ID;
;
properties for object materials
struct OBJECTMATERIAL
float ambientStrength;
glm::vec ambientColor;
glm::vec diffuseColor;
glm::vec specularColor;
float shininess;
std::string tag;
;
private:
pointer to shader manager object
ShaderManager mpShaderManager;
pointer to basic shapes object
ShapeMeshes mbasicMeshes;
total number of loaded textures
int mloadedTextures;
loaded textures info
TEXTUREINFO mtextureIDs;
defined object materials
std::vector mobjectMaterials;
set the transformation values
into the transform buffer
void SetTransformations
glm::vec scaleXYZ,
float XrotationDegrees,
float YrotationDegrees,
float ZrotationDegrees,
glm::vec positionXYZ;
set the color values into the shader
void SetShaderColor
float redColorValue,
float greenColorValue,
float blueColorValue,
float alphaValue;
public:
The following methods are for the students to
customize for their own D scene
void PrepareScene;
void RenderScene;
;
ViewManager.cpp
viewmanager.h
manage the viewing of D objects within the viewport camera, projection
#pragma once
#include "ShaderManager.h
#include "camera.h
GLFW library
#include GLFWglfwh
class ViewManager
public:
constructor
ViewManager
ShaderManager pShaderManager;
destructor
~ViewManager;
mouse position callback for mouse interaction with the D scene
static void MousePositionCallbackGLFWwindow window, double xMousePos, double yMousePos;
mouse wheel scrolling callback for mouse interaction with the D scene
static void MouseWheelCallbackGLFWwindow window, double x double yScrollDistance;
private:
pointer to shader manager object
ShaderManager mpShaderManager;
active OpenGL display window
GLFWwindow mpWindow;
process keyboard events for interaction with the D scene
void ProcessKeyboardEvents;
public:
create the initial OpenGL display window
GLFWwindow CreateDisplayWindowconst char windowTitle;
prepare the conversion from D object display to D scene display
void PrepareSceneView;
;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
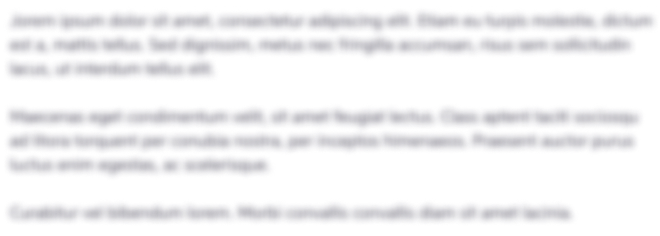
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started