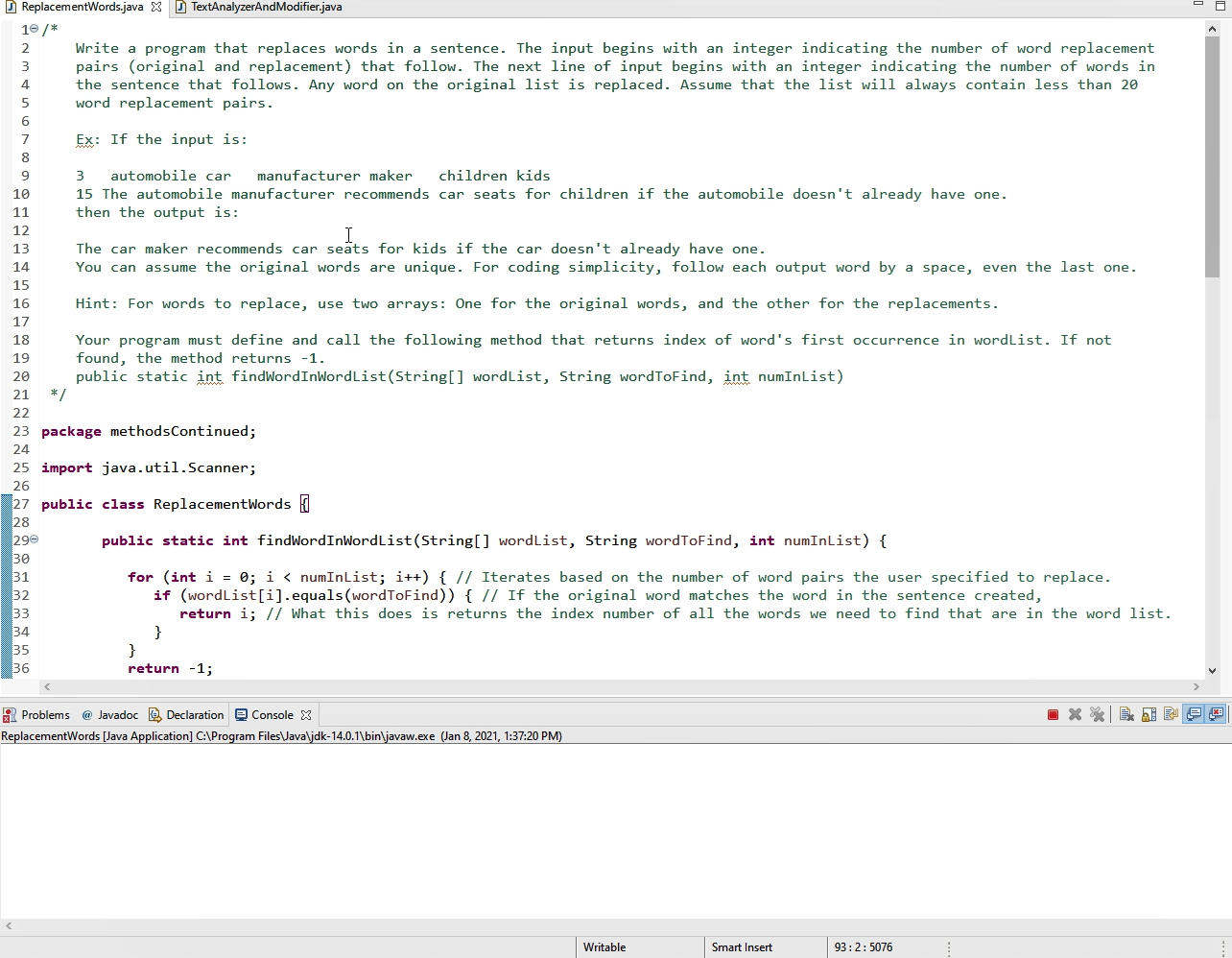
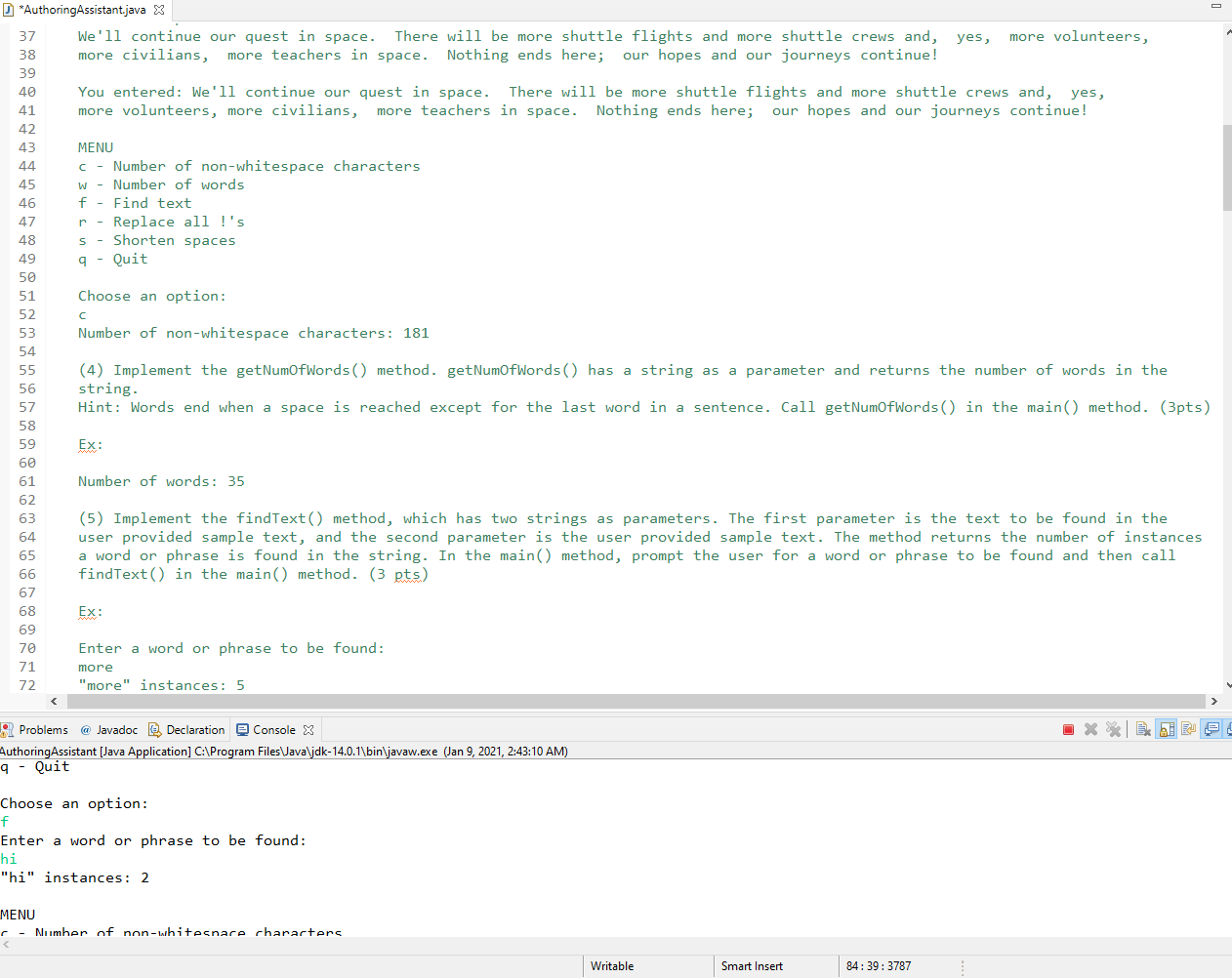
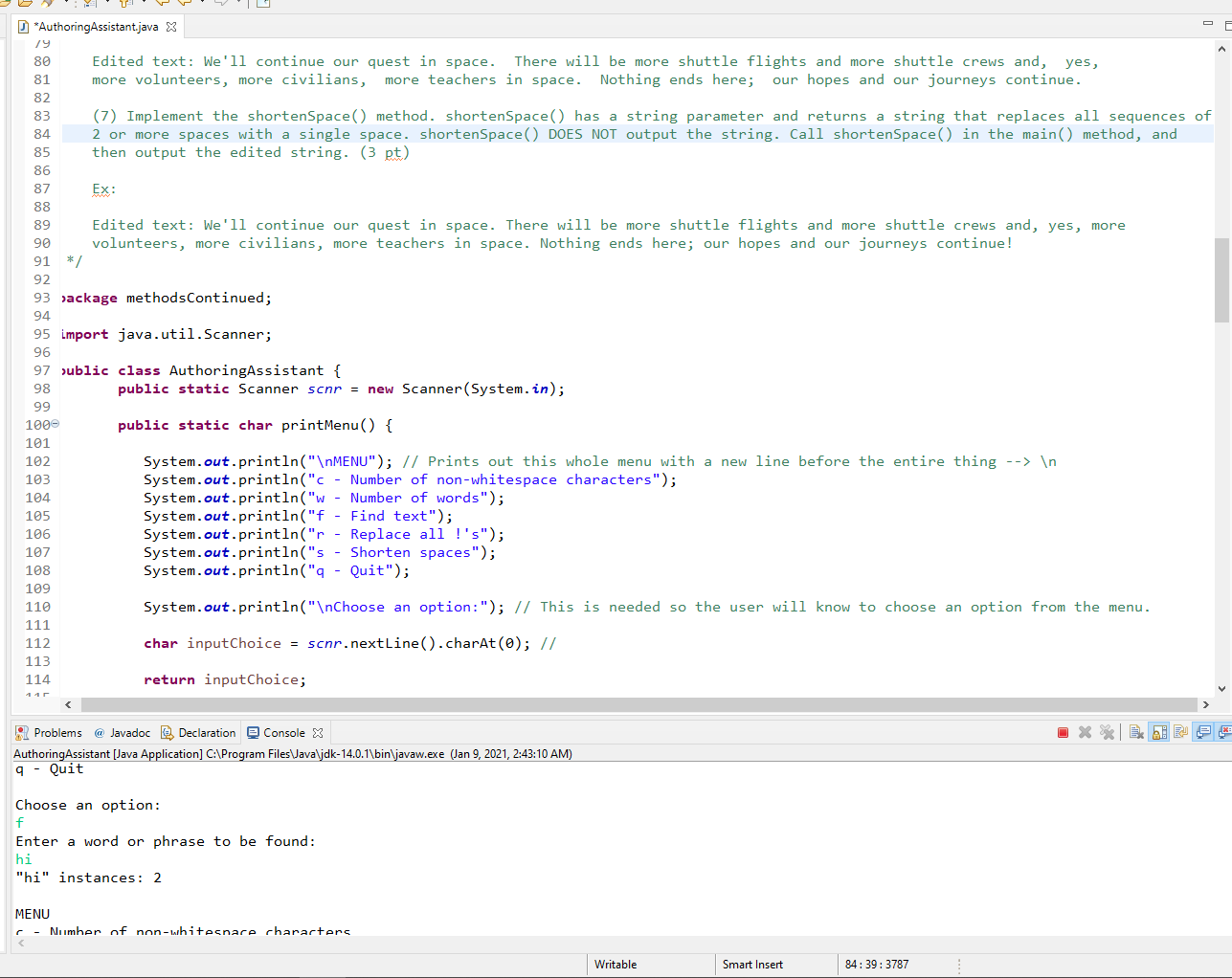
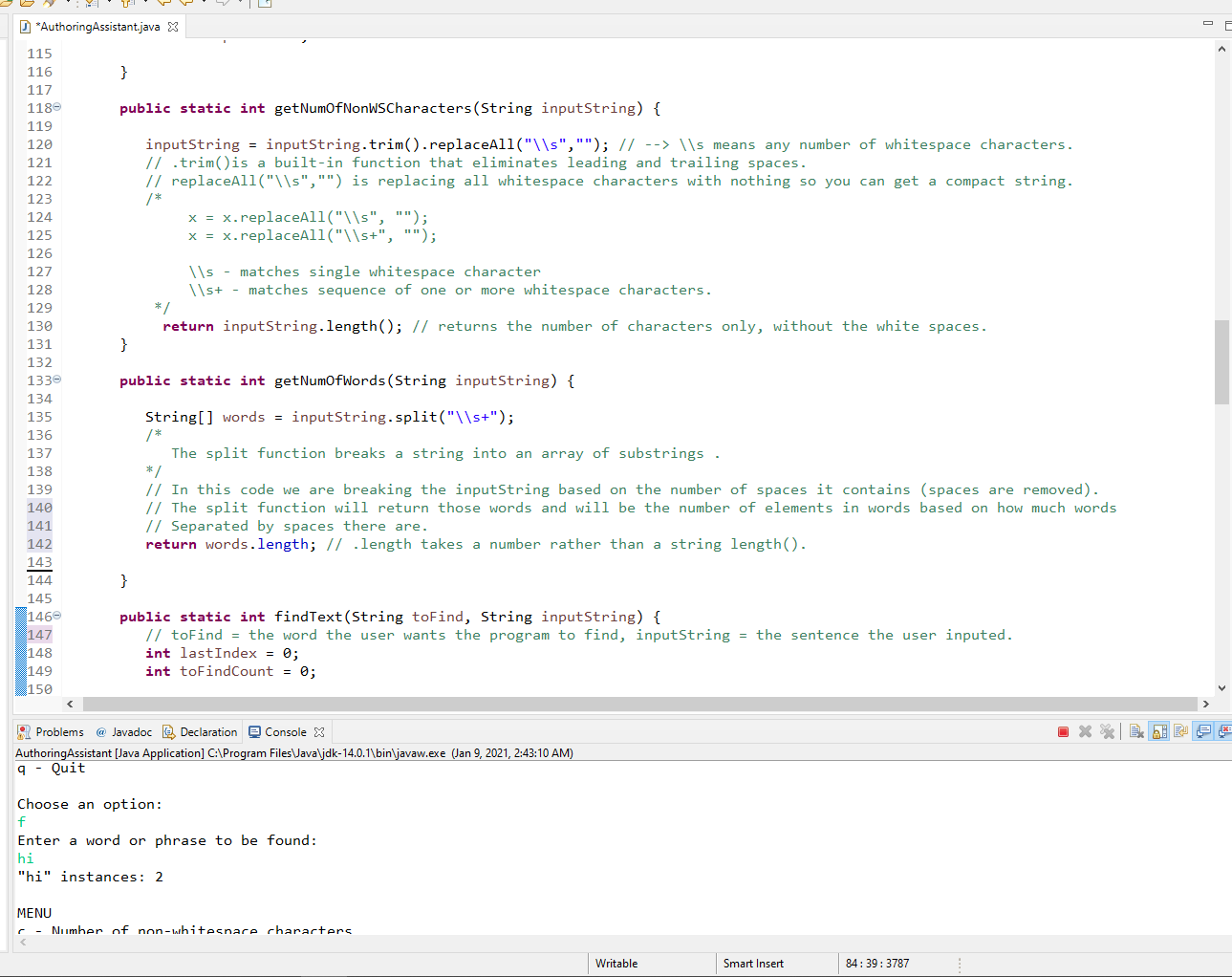
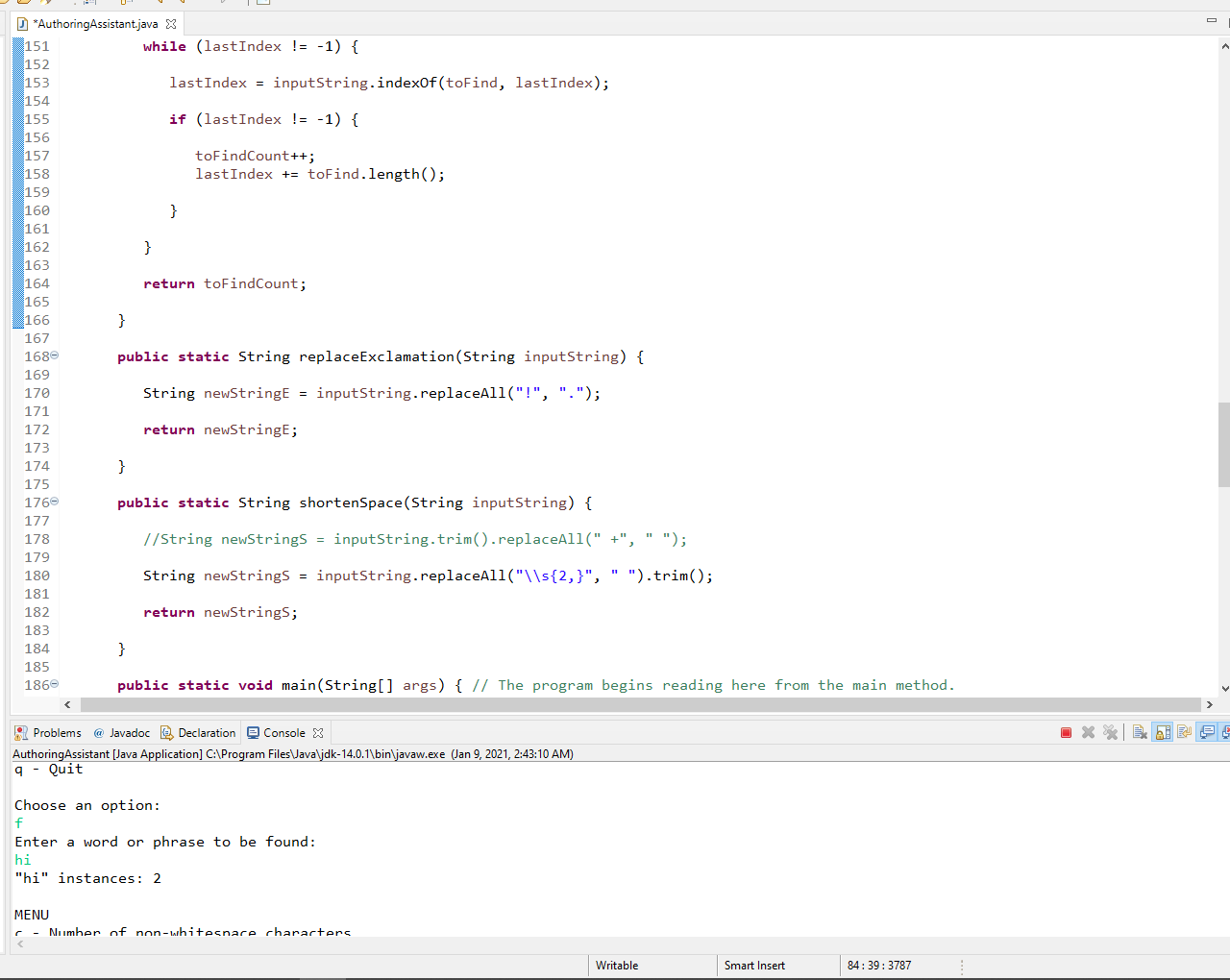
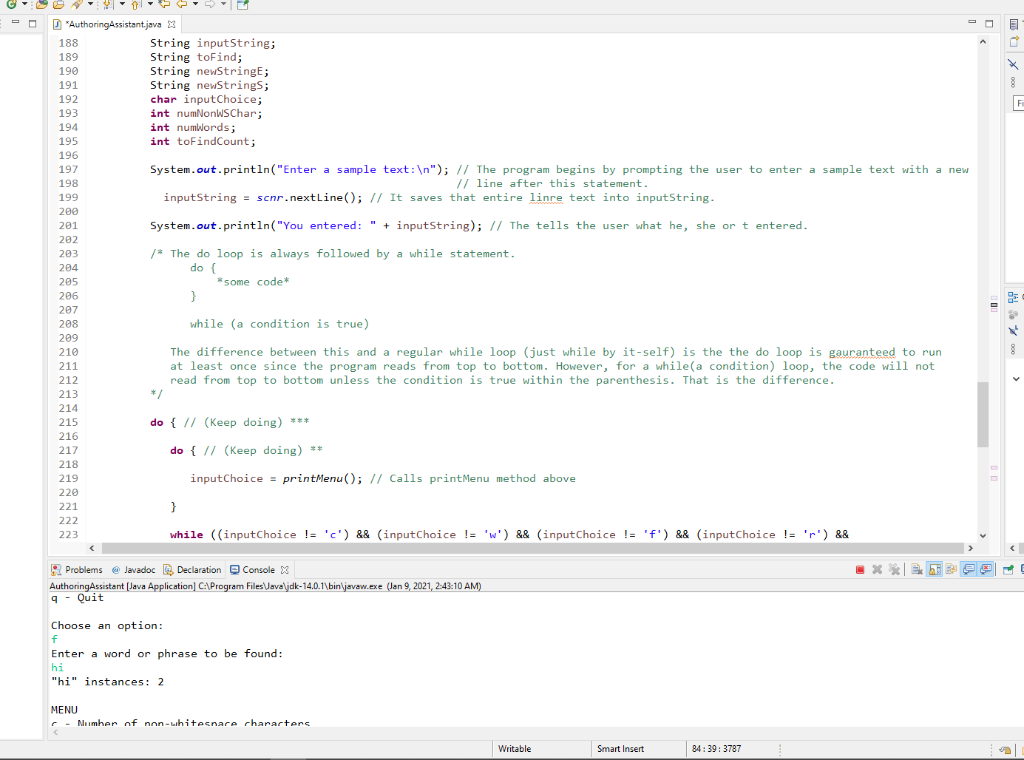
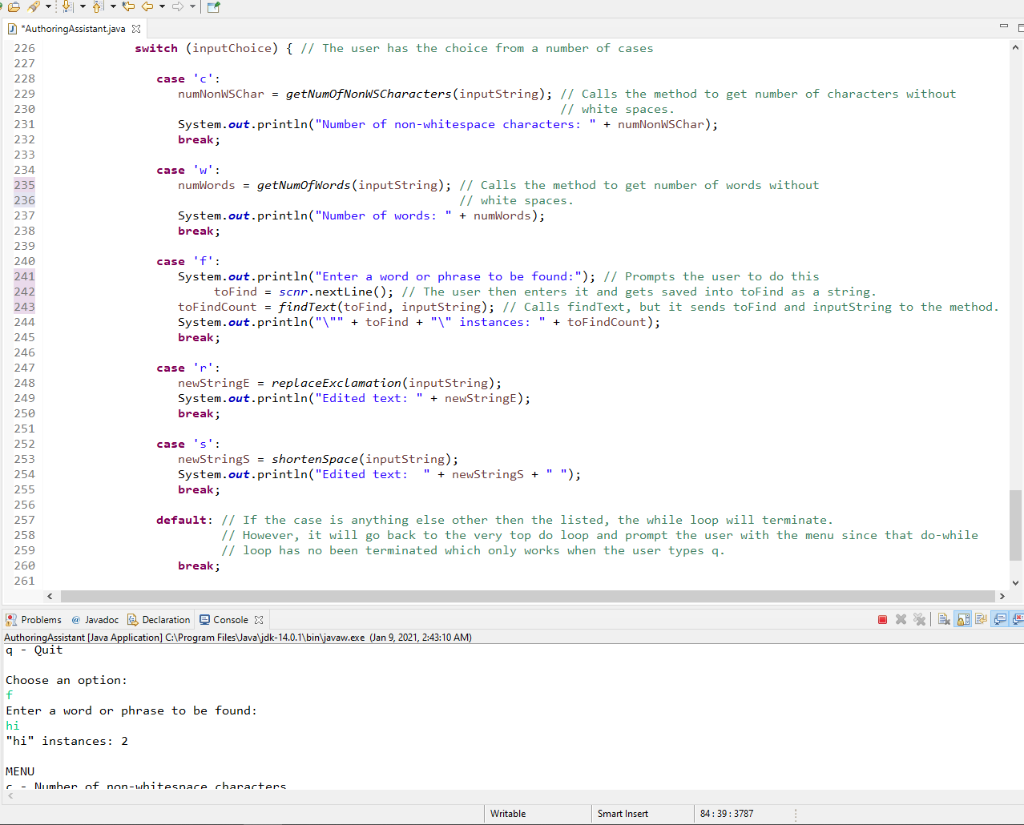
Can you explain how lines 146 - 184 work? I want to add comments or boxes to help me understand this code.
ReplacementWords.java X TextAnalyzerAnd Modifier.java 1/* 2 Write a program that replaces words in a sentence. The input begins with an integer indicating the number of word replacement 3 pairs (original and replacement) that follow. The next line of input begins with an integer indicating the number of words in 4 the sentence that follows. Any word on the original list is replaced. Assume that the list will always contain less than 20 5 word replacement pairs. 6 7 Ex: If the input is: 8 3 automobile car manufacturer maker children kids 15 The automobile manufacturer recommends car seats for children if the automobile doesn't already have one. then the output is: I The car maker recommends car seats for kids if the car doesn't already have one. You can assume the original words are unique. For coding simplicity, follow each output word by a space, even the last one. 9 10 11 12 13 14 15 16 Hint: For words to replace, use two arrays: One for the original words, and the other for the replacements. 17 18 19 Your program must define and call the following method that returns index of word's first occurrence in wordList. If not found, the method returns -1. 20 public static int findWordInWordList(String[] wordList, String wordToFind, int numInList) 21 */ 22 23 package methodsContinued; 24 25 import java.util.Scanner; 26 27 public class ReplacementWords ! 28 290 public static int findWordInWordList(String[] wordList, String wordToFind, int numInList) { 30 for (int i = 0; i 103 System.out.println("c - Number of non-whitespace characters"); 104 System.out.println("W Number of words"); 105 System.out.println("f Find text"); 106 System.out.println("r Replace all !'s"); 107 System.out.println("s Shorten spaces"); 108 System.out.println("q - Quit"); 109 11 System.out.println(" Choose an option:"); // This is needed so the user will know to choose an option from the menu. 111 112 char inputChoice = scnr.nextLine().charAt(0); // 113 114 return inputChoice; A Problems @ Javadoc Declaration Console X AuthoringAssistant [Java Application] C:\Program Files\Java\jdk-14.0.1\bin\javaw.exe (Jan 9, 2021, 2:43:10 AM) 9 - Quit Choose an option: f Enter a word or phrase to be found: hi "hi" instances: 2 MENU - Number of non-whitesnace characters Writable Smart Insert 84: 39: 3787 D'AuthoringAssistant.java X } public static int getNumOfNonWSCharacters (String inputString) { inputString = inputString.trim().replaceAll("\\s",""); // --> \\s means any number of whitespace characters. Il .trim()is a built-in function that eliminates leading and trailing spaces. // replaceAll("\\s","") is replacing all whitespace characters with nothing so you can get a compact string. /* x = x.replaceAll("s", ""); x = x.replaceAll("\\s+", ""); \\s - matches single whitespace character \\s+ - matches sequence of one or more whitespace characters. */ return inputString.length(); // returns the number of characters only, without the white spaces. } 115 116 117 1180 119 120 121 122 123 124 125 126 127 128 129 130 131 132 1330 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 public static int getNumOfWords (String inputString) { String[] words = inputString.split("\\s+"); The split function breaks a string into an array of substrings. */ // In this code we are breaking the inputString based on the number of spaces it contains (spaces are removed). // The split function will return those words and will be the number of elements in words based on how much words // Separated by spaces there are. return words.length; // length takes a number rather than a string length(). } public static int findText(String toFind, String inputString) { // toFind = the word the user wants the program to find, inputString = the sentence the user inputed. int lastIndex = 0; int toFindCount = 0; A Problems @ Javadoc Declaration Console X Authoring Assistant [Java Application] C:\Program FilesVava\jdk-14.0.1\bin\javaw.exe (Jan 9, 2021, 2:43:10 AM) 4 - Quit Choose an option: f Enter a word or phrase to be found: hi "hi" instances: 2 MENU - Number of non-whitesnace characters Writable Smart Insert 84: 39: 3787 151 D'Authoring Assistant.java X while (lastIndex != -1) { 152 153 lastIndex = inputString.indexOf(toFind, lastIndex); 154 155 if (lastIndex != -1) { 156 157 toFindCount++; 158 lastIndex += toFind.length(); 159 160 } 161 162 } 163 164 return toFindCount; 165 166 } 167 1680 public static String replaceExclamation(String inputString) { 169 String newStringe = inputString.replaceAll("!", "."); 171 172 return newStringE; 173 174 } 175 1760 public static String shortenSpace(String inputString) { 177 178 //String newStrings = inputString.trim().replaceAll(" +", " "); 179 180 String newStrings = inputString.replaceAll("\ls{2,}", " ").trim(); 181 182 return newStrings; 183 184 } 185 1860 public static void main(String[] args) { // The program begins reading here from the main method. 170 PS A Problems @ Javadoc Declaration Console X Authoring Assistant [Java Application] C:\Program Files\Java\jdk-14.0.1\bin\javaw.exe (Jan 9, 2021, 2:43:10 AM) q - Quit Choose an option: f Enter a word or phrase to be found: hi "hi" instances: 2 MENU - Number of non-whitesnace characters Writable Smart Insert 84: 39: 3787 8 F "AuthoringAssistant.java 2 188 String inputString; 189 String to Find; 190 String newStringE; 191 String newStrings; 192 char inputChoice; 193 int numNonWsChar; 194 int numWords; 195 int to FindCount; 196 197 System.out.println("Enter a sample text: "); // The program begins by prompting the user to enter a sample text with a new 198 // line after this statement. 199 inputString = scnr.nextLine(); // It saves that entire linre text into inputString. 200 201 System.out.println("You entered: " + inputString); // The tells the user what he, she or t entered. 202 203 /* The do loop is always followed by a while statement. 204 do { 205 *some code* 206 } 207 208 while (a condition is true) 209 210 The difference between this and a regular while loop (just while by it-self) is the the do loop is gauranteed to run 211 at least once since the program reads from top to bottom. However, for a while(a condition) loop, the code will not 212 read from top to bottom unless the condition is true within the parenthesis. That is the difference. 213 */ 214 215 do { // (Keep doing) *** 216 217 do { // (Keep doing) ** 218 219 inputChoice = printMenu(); // Calls printMenu method above 220 221 } 222 223 while ((inputChoice != 'c') && (inputChoice != 'W') && (inputChoice != 'f') && (inputChoice != 'r' && R Problems Javadoc Declaration Console X AuthoringAssistant [Java Application] C:\Program Files\Javaljdk-14.0.1\bin\javaw.exe (Jan 9, 2021, 2:43:10 AM) 4 - Quit Choose an option: f Enter a word or phrase to be found: hi "hi" instances: 2 MENU Number of non-whitesnare characters Writable Smart Insert 84:39:3787 Authoring Assistant.java X 226 switch (inputChoice) { // The user has the choice from a number of cases 227 228 case 'c': 229 numNonWSchar = getNumOfNonsCharacters (inputString); // Calls the method to get number of characters without 230 // white spaces. 231 System.out.println("Number of non-whitespace characters: " + numNonWSChar); 232 break; 233 234 case 'w': 235 numbords = getNumOfWords (inputString); // Calls the method to get number of words without 236 // white spaces. 237 System.out.println("Number of words: " + numWords); 238 break; 239 240 case 'f': 241 System.out.println("Enter a word or phrase to be found :"); // Prompts the user to do this 242 toFind = scnr.nextLine(); // The user then enters it and gets saved into toFind as a string. 243 toFindCount = findText (toFind, inputString); // Calls findText, but it sends toFind and inputString to the method. 244 System.out.println(""* + toFind + "\" instances: " + toFind Count); 245 246 247 case 'r': 248 newStringe = replaceExclamation (inputString); 249 System.out.println("Edited text: " + newString); 250 break; 251 252 case 's': 253 newStrings = shortenSpace(inputString); 254 System.out.println("Edited text: " + newStrings + " "); 255 break; 256 257 default: // If the case is anything else other then the listed, the while loop will terminate. 258 // However, it will go back to the very top do loop and prompt the user with the menu since that do-while 259 // loop has no been terminated which only works when the user types q. 260 break; 261 break; X XB Problems @ Javadoc Declaration Console x3 AuthoringAssistant [Java Application] C:\Program Files\Java\jdk-14.0.1\binjavaw.exe (Jan 9, 2021, 2:43:10 AM) g Quit Choose an option: f Enter a word or phrase to be found: hi "hi" instances: 2 MENU - Number of non-whitesnare characters Writable Smart Insert 84:39:3787 D "AuthoringAssistant.java X 234 case 'w': numWords = getNumOfWords (inputString); // Calls the method to get number of words without // white spaces. System.out.println("Number of words: " + numWords); break; case 'f': System.out.println("Enter a word or phrase to be found :"); // Prompts the user to do this toFind = scnr.nextLine(); // The user then enters it and gets saved into toFind as a string. toFindCount = findText(toFind, inputString); // Calls findText, but it sends toFind and inputString to the method. System.out.println(""" + toFind + "\" instances: " + toFindCount); break; case 'r': newStringE = replaceExclamation(inputString); System.out.println("Edited text: " + newStringE); break; 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 ) 269 case 's': newStrings = shortenSpace(inputString); System.out.println("Edited text: + newStrings + " "); break; default: // If the case is anything else other then the listed, the while loop will terminate. // However, it will go back to the very top do loop and prompt the user with the menu since that do-while // loop has no been terminated which only works when the user types q. break; } } while (inputChoice != 'q'); // (while the inputChoice does not = q) *** A Problems @ Javadoc Declaration Console X AuthoringAssistant [Java Application] C:\Program Files\Java\jdk-14.0.1\bin\javaw.exe (Jan 9, 2021, 2:43:10 AM) a Quit Choose an option: f Enter a word or phrase to be found: hi "hi" instances: 2 MENU Number of non-bitocnacochanactone