Answered step by step
Verified Expert Solution
Question
...
1 Approved Answer
Can you fix my driver class and checkbudget method. The checkbudget method should check to see if the given budget is large enough to pay
Can you fix my driver class and checkbudget method. The checkbudget method should check to see if the given budget is large enough to pay for everything in the cart. If not, remove an Item from the shopping cart, one at a time Here is the code import java.util.ArrayList; import java.util.List; import java.util.Random; public class ShoppingCart private List cart; array based implementation public ShoppingCart cart new ArrayList; initializing an array list for cart public void addItemItem item cart.additem; add an item to the cart public void addMultipleItemsItem item, int quantity for int i ; i quantity; i cart.additem; add nultiple items to the cart public boolean removeItemItem item return cart.removeitem; remove a specified item removes random unspecified item public void removeUnspecifiedItem if cart.isEmpty Random random new Random; int randomIndex random.nextIntcartsize; cart.removerandomIndex; public void checkout double totalCost ; System.out.printlnItems in the cart:"; for Item item : cart System.out.printlnitem; totalCost item.getPrice; Convert price to dollars and add to total System.out.printlnTotal cost: $ totalCost; public boolean checkBudgetdouble budget double totalCost calculateTotalCost; if totalCost budget System.out.printlnBudget is sufficient."; return true; else while totalCost budget removeUnspecifiedItem; return true; private double calculateTotalCost double totalCost ; for Item item : cart totalCost item.getPrice; Convert price to dollars and add to total return totalCost; Item.java implementation of an Item to be placed in ShoppingCart public class Item private String name; private int price; in cents Constructor public ItemString n int p name n; price p; public boolean equalsObject other if thisgetClass other.getClass other null return false; Item otherItem Item other; return this.name.equalsotherItemname && this.price otherItem.price; displays name of item and price in properly formatted manner public String toString return name price: $ price price; Getter methods public int getPrice return price; public String getName return name; public class ShoppingCartDriver public static void mainString args Create some Item instances Item item new ItemItem ; $ Item item new ItemItem ; $ Item item new ItemItem ; $ Item item new ItemItem ; $ Create a shopping cart ShoppingCart cart new ShoppingCart; Add items to the shopping cart cart.addItemitem; cart.addMultipleItemsitem; cart.addItemitem; cart.addItemitem; remove items cart.removeItemitem; cart.removeUnspecifiedItem; Test the checkBudget method double budget ; $ budget boolean isBudgetSufficient cart.checkBudgetbudget; if isBudgetSufficient System.out.printlnProceed with the purchase."; else System.out.printlnAdjust your cart items to fit the budget."; Simulate the checkout process System.out.printlnItems in the cart:"; cart.checkout;
Can you fix my driver class and checkbudget method. The checkbudget method should check to see if the given budget is large enough to pay
for everything in the cart. If not, remove an Item from the shopping cart, one at a time Here is the code import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class ShoppingCart
private List cart; array based implementation
public ShoppingCart
cart new ArrayList; initializing an array list for cart
public void addItemItem item
cart.additem; add an item to the cart
public void addMultipleItemsItem item, int quantity
for int i ; i quantity; i
cart.additem; add nultiple items to the cart
public boolean removeItemItem item
return cart.removeitem; remove a specified item
removes random unspecified item
public void removeUnspecifiedItem
if cart.isEmpty
Random random new Random;
int randomIndex random.nextIntcartsize;
cart.removerandomIndex;
public void checkout
double totalCost ;
System.out.printlnItems in the cart:";
for Item item : cart
System.out.printlnitem;
totalCost item.getPrice; Convert price to dollars and add to total
System.out.printlnTotal cost: $ totalCost;
public boolean checkBudgetdouble budget
double totalCost calculateTotalCost;
if totalCost budget
System.out.printlnBudget is sufficient.";
return true;
else
while totalCost budget
removeUnspecifiedItem;
return true;
private double calculateTotalCost
double totalCost ;
for Item item : cart
totalCost item.getPrice; Convert price to dollars and add to total
return totalCost;
Item.java implementation of an Item to be placed in ShoppingCart
public class Item
private String name;
private int price; in cents
Constructor
public ItemString n int p
name n;
price p;
public boolean equalsObject other
if thisgetClass other.getClass other null
return false;
Item otherItem Item other;
return this.name.equalsotherItemname && this.price otherItem.price;
displays name of item and price in properly formatted manner
public String toString
return name price: $ price price;
Getter methods
public int getPrice
return price;
public String getName
return name;
public class ShoppingCartDriver
public static void mainString args
Create some Item instances
Item item new ItemItem ; $
Item item new ItemItem ; $
Item item new ItemItem ; $
Item item new ItemItem ; $
Create a shopping cart
ShoppingCart cart new ShoppingCart;
Add items to the shopping cart
cart.addItemitem;
cart.addMultipleItemsitem;
cart.addItemitem;
cart.addItemitem;
remove items
cart.removeItemitem;
cart.removeUnspecifiedItem;
Test the checkBudget method
double budget ; $ budget
boolean isBudgetSufficient cart.checkBudgetbudget;
if isBudgetSufficient
System.out.printlnProceed with the purchase.";
else
System.out.printlnAdjust your cart items to fit the budget.";
Simulate the checkout process
System.out.printlnItems in the cart:";
cart.checkout;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
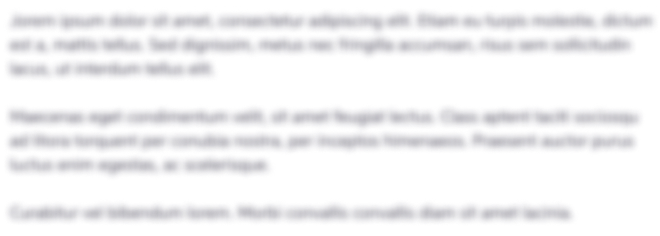
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started