Question
Can you fix the errors in the code, please? The code needs to print the predictions to a new .csv file. Please don't abuse/spam... #include
Can you fix the errors in the code, please? The code needs to print the predictions to a new .csv file. Please don't abuse/spam...
#include
// A struct to represent a rating given by a user to an item struct Rating { int user_id; int item_id; float rating; };
// A struct to represent a predicted rating for an item struct PredictedRating { int id; int user_id; int item_id; float rating; };
// Reads the ratings from a .csv file and returns them as a vector std::vector
std::ifstream file(filename); if (file.is_open()) { std::string line; // Skip the first line (header) std::getline(file, line); while (std::getline(file, line)) { Rating rating; std::sscanf(line.c_str(), "%d,%d,%f", &rating.user_id, &rating.item_id, &rating.rating); ratings.push_back(rating); } file.close(); }
return ratings; }
// Reads the test cases from a .csv file and returns them as a vector std::vector
std::ifstream file(filename); if (file.is_open()) { std::string line; // Skip the first line (header) std::getline(file, line); while (std::getline(file, line)) { PredictedRating test_case; std::sscanf(line.c_str(), "%d,%d,%d", &test_case.id, &test_case.user_id, &test_case.item_id); test_cases.push_back(test_case); } file.close(); }
return test_cases; }
// Calculates the root mean squared error between the predicted ratings and the actual ratings float calculate_rmse(const std::vector
// Calculates the dot product of two vectors float dot_product(const std::unordered_map
// Calculates the cosine similarity between two vectors float cosine_similarity(const std::unordered_map
// Predict the rating for a given user and item using cosine similarity float predict_rating_cosine(int user_id, int item_id, const std::unordered_map
// Calculate the cosine similarity between the given user and all other users std::vector
// Sort the users by their similarity to the given user std::sort(similarities.begin(), similarities.end(), [](const auto& a, const auto& b) { return a.second > b.second; });
// Use the top k most similar users to predict the rating constexpr int k = 50; if (similarities.size() < k) { return 0.0f; } float sum_similarities = 0.0f; float sum_ratings = 0.0f; for (int i = 0; i < k; i++) { int other_user = similarities[i].first; float similarity = similarities[i].second; float rating = user_item_ratings.at(other_user).at(item_id); sum_similarities += similarity; sum_ratings += similarity * rating; } if (sum_similarities == 0) { return 0.0f; } return sum_ratings / sum_similarities; }
int main() { // Read in the training and test data const auto ratings = read_ratings("train.csv"); const auto test_cases = read_test_cases("test.csv");
// Initialize a map to store the ratings given by each user std::unordered_map
// Initialize a map to store the ratings received by each item std::unordered_map
std::fstream fout;
// opens an existing csv file or creates a new file. fout.open("report.csv", std::ios::out | std::ios::app);
// Predict the ratings for the test cases std::vector
// Calculate the root mean squared error float rmse = calculate_rmse(predicted_ratings, ratings); std::cout << "RMSE: " << rmse << std::endl;
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
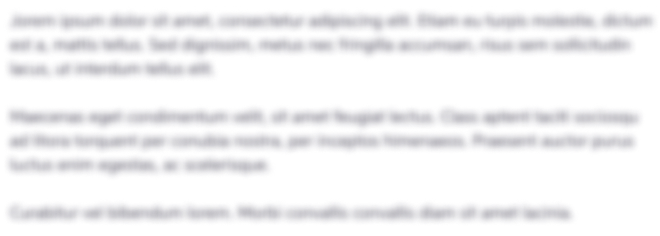
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started