Question
Can you help me fix my PlayTest.java class so it can compile? 1. This class should consist of a main method that tests all the
Can you help me fix my PlayTest.java class so it can compile? 1. This class should consist of a main method that tests all the methods in each class, either directly by calling the method from the Tester or indirectly by having another method call a method.
2. Players, Course and Score information will be read from a file.
3. The file created should include valid and invalid data that fully tests the application.
4. Included file called data.txt contains Player's data. Note the file format is as follows:
1. Number of scores
2. Char: Type of player (G - golfer, B - bowler)
3. Name data etc
4. Score data
5. There will be an array of Players objects.
1. Populate the Array with both Golfer and Bowler objects.
2. After the Array is filled, call the toString method on each object in the Array. Ensure each iteration calls the correct object.
Data.txt (input from file)
2 G Hugh Hefner,Rocky Bayou Country Club Bluewater Country Club,75,10/23/2013,70.5,113 Rocky Bayou Country Club,82,01/21/2014,68.5,123 3 B Dan Bilzerian,Ignite Felton Lanes, 112,09/22/2012 Bowlarama, 142,09/24/2012 Cordova Lanes,203,09/24/2012 2 G Tiger Woods,Isleworth Augusta,68,04/08/2013,76.2,135 Bethpage Black,71,06/21/2012,76.6,144 3 B Earl Anthony,Nine Mile Denny's Firestone Lanes, 223,09/22/1968 Bowlarama, 246,09/24/1976 Cordova Lanes,210,09/24/1977
PlayerTest.java
import java.util.Scanner; import java.io.FileNotFoundException; import java.io.FileInputStream; public class PlayerTest { public static void main(String[] args) { Scanner fileIn = null; try{ fileIn = new Scanner(new FileInputStream("data.txt")); }catch (FileNotFoundException e){ System.out.println("File not found."); System.exit(0); } Player[] playerList = new Player[100]; int i = 0; int numScores; char type; String namesAndOthers; String blankLine; String singleScore; do{ numScores = fileIn.nextInt(); type = fileIn.next().charAt(0); blankLine = fileIn.nextLine(); namesAndOthers = fileIn.nextLine(); String[] nameAndOthersArray = namesAndOthers.split("s*,s*"); if( type == 'G' ){ Golfer newGolferPlayer = new Golfer(nameAndOthersArray[0], nameAndOthersArray[1]); //HELP for (int j = 0; j < numScores; j++) { singleScore = fileIn.nextLine(); String[] singleScoreArray = singleScore.split("s*,s*"); //HELP newGolferPlayer.addScore( singleScoreArray[0], Integer.parseInt(singleScoreArray[1]), singleScoreArray[2], Double.parseDouble(singleScoreArray[3]), Integer.parseInt(singleScoreArray[4])); //HELP } playerList[i] = newGolferPlayer; }else if ( type == 'B' ){ Bowler newBowlerPlayer = new Bowler(nameAndOthersArray[0], nameAndOthersArray[1]); //HELP for (int j = 0; j < numScores; j++) { singleScore = fileIn.nextLine(); String[] singleScoreArray = singleScore.split("s*,s*"); BowlerScore newBowlerScore = new BowlerScore( singleScoreArray[0], Integer.parseInt(singleScoreArray[1]), singleScoreArray[2] ); newBowlerPlayer.addScore( newBowlerScore ); } playerList[i] = newBowlerPlayer; }else{ System.out.println("Not Golfer or Bowler Player"); } i++; }while ( fileIn.hasNextInt()); { int counter = 0; for ( i = 0; i < playerList.length; i ++) if (playerList[i] != null) counter ++; for ( i =0; i< counter; i++ ){ System.out.println(playerList[i]); } } } fileIn.close(); //ERROR }
Player.java
public abstract class Player { String name; int IDNum; static int nextIDNum = 1000; public Player() { super(); name =""; IDNum = 0; } public Player(String name, int iDNum) { super(); this.name = name; this.IDNum = iDNum; } public String getName() { return name; } public void setName(String fName) { this.name = fName; } public int getIDNum() { return IDNum; } public void setIDNum(int iDNum) { IDNum = getNextIDNum()+1; setNextIDNum(getIDNum()); } public static int getNextIDNum() { return nextIDNum; } public void setNextIDNum(int nextIDNum) { Player.nextIDNum = nextIDNum; } public abstract double calculateHandicap(); //represents # of handicap players }
Course.java
public class Course { String courseName; double courseRating; int courseSlope; public Course() { super(); courseName = ""; courseRating = 0.0; courseSlope = 0; } public Course(String courseName, double courseRating, int courseSlope) { super(); this.courseName = courseName; this.courseRating = courseRating; this.courseSlope = courseSlope; } public String getCourseName() { return courseName; } public void setCourseName(String courseName) { this.courseName = courseName; } public double getCourseRating() { return courseRating; } public void setCourseRating(double fcourseRating) { if( fcourseRating >= 60 && fcourseRating <= 80 ) { courseRating = fcourseRating; }else{ System.out.println("Course rating invalid."); courseRating = 9999; } } public int getCourseSlope() { return courseSlope; } public void setCourseSlope(int fcourseSlope) { if( fcourseSlope >= 55 && fcourseSlope <= 155 ) { courseSlope = fcourseSlope; }else{ System.out.println("Course slope invalid."); courseSlope = 9999; } } public String toString() { return getCourseName() + " " + getCourseRating() + " " + getCourseSlope() + " "; } }
Score.java
public class Score { int score; String date; private Course theCourse; public Score() { super(); score = 0; date = ""; theCourse = new Course("", 0, 0); } public Score(int score, String date, Course course) { super(); this.score = score; this.date = date; } public int getScore() { return score; } public void setScore(int fScore) { if( fScore >= 40 && fScore <= 200 ) { score = fScore; }else{ System.out.println("Invalid Score Entry."); score = 9999; } } public String getDate() { return date; } public void setDate(String date) { this.date = date; } public Course getCourse() { return theCourse; } public String toString() { if( getScore() == 9999 && !getDate().equals("INVALID") ){ return getScore() + " " + getDate() + " " + getCourse().toString(); }else if ( getDate().equals("INVALID") && getScore() != 9999 ){ return getScore() + " " + getDate() + " " + getCourse().toString(); }else if ( getScore() == 9999 && getDate().equals("INVALID") ){ return getScore() + " " + getDate() + " " + getCourse().toString(); } return getScore() + " " + getDate() + " " + getCourse().toString(); } }
BowlerScore.java
import java.util.GregorianCalendar; public class BowlerScore { String laneName; int score; String date; public BowlerScore() { super(); } public BowlerScore(String laneName, int score, String date) { super(); this.laneName = laneName; this.score = score; this.date = date; } public String getLaneName() { return laneName; } public void setLaneName(String laneName) { this.laneName = laneName; } public int getScore() { return score; } public void setScore(int fscore) { if( fscore >= 0 && fscore <= 300 ) { score = fscore; }else{ score = 9999; } } public String getDate() { return date; } public void setDate(String fdate) { GregorianCalendar calendar = new GregorianCalendar(); calendar.setLenient(false); String[] splitDate = fdate.split("/"); int month = Integer.parseInt( splitDate[0] ); int days = Integer.parseInt( splitDate[1] ); int year = Integer.parseInt( splitDate[2] ); calendar.set(year, month, days); try{ calendar.getTime(); }catch (Exception e){ fdate = "INVALID"; } date = fdate; } public String toString() { if ( getScore() == 9999 && !getDate().equals("INVALID") ){ return getScore() + " " + getDate() + " " + getLaneName() + " "; }else if ( getDate().equals("INVALID") && getScore() != 9999 ){ return getScore() + " " + getDate() + " " + getLaneName() + " "; }else if ( getScore() == 9999 && getDate().equals("INVALID") ){ return getScore() + " " + getDate() + " " + getLaneName() + " "; } return getScore() + " " + getDate() + " " + getLaneName() + " "; } }
Step by Step Solution
3.44 Rating (151 Votes )
There are 3 Steps involved in it
Step: 1
Remove the unnecessary block after the dowhile loop The closing bracket before the for loop is causi...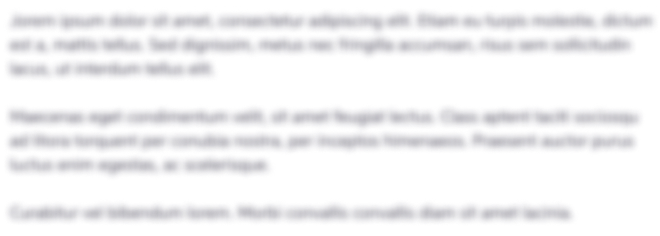
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started