Question
Can you help me fix the exception? I have an Exception in thread main java.lang.NullPointerException import java.util.List; public class Scheduler { static double idleTime =
Can you help me fix the exception? I have an Exception in thread "main" java.lang.NullPointerException
import java.util.List;
public class Scheduler {
static double idleTime = 0;
static Queue queue = new FIFOQueue();
public static void executeProcesses() {
double currentTime = 0;
System.out.println("Simulation starts with default parameters.");
while (!queue.isEmpty()) {
Process process = queue.poll();
if (process.getArrivalTime() > currentTime) {
idleTime = process.getArrivalTime() - currentTime;
currentTime = process.getArrivalTime();
}
System.out.printf("process %d started at time %f. ", process.getId(), currentTime);
do {
double currentBurstTime = process.getNextBurstTime();
System.out.printf("process %d has CPU burst %f. ", process.getId(), currentBurstTime);
currentTime += currentBurstTime;
} while (process.hasMoreBurst());
System.out.printf("process %d completes and terminates at %f. ", process.getId(), currentTime);
}
System.out.println("Simulation summary");
System.out.printf("CPU idle time: %f ", idleTime);
System.out.printf("CPU idle percentage: %f ", idleTime / currentTime);
System.out.println("Signature name: " + queue.getSignature());
}
public static void main(String[] args) {
ProcessFactory factory = new ProcessFactory();
List
processList.forEach(p -> queue.offer(p));
executeProcesses();
}
}
package csc3502.processsimulator;
public interface Queue {
void offer(Process p);
Process poll();
boolean isEmpty();
String getSignature();
}
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class ProcessFactory {
private int id = 1;
private double arrivalTime = 0;
private int priority = 1;
// setting parameters
private double maxBurst = 60;
private double minBurst = 10;
private double mean = 50;
private double stdDeviance = 30;
private int numOfProcess = 100;
public ProcessFactory() {
}
public ProcessFactory(double mean, double stdDeviance, double maxBurst, double minBurst, int numOfProcess) {
this.mean = mean;
this.stdDeviance = stdDeviance;
this.maxBurst = maxBurst;
this.minBurst = minBurst;
this.numOfProcess = numOfProcess;
}
public List
List
for (int i = 0; i < numOfProcess; i++) {
processList.add(generate());
}
return processList;
}
public Process generate() {
return new Process(getId(), getArrivalTime(), getPriority(), maxBurst, minBurst);
}
private int getId() {
return id++;
}
private double getArrivalTime() {
arrivalTime += new Random().nextGaussian() * stdDeviance + mean;
return arrivalTime;
}
private int getPriority() {
return priority;
}
}
public class Process {
private int id;
private double arrivalTime;
private int priority;
// setting parameters
private double maxBurst = 60;
private double minBurst = 10;
public Process(int id, double arrivalTime, int priority, double maxBurst, double minBurst) {
this.id = id;
this.arrivalTime = arrivalTime;
this.priority = priority;
this.maxBurst = maxBurst;
this.minBurst = minBurst;
}
public int getId() {
return id;
}
public double getArrivalTime() {
return arrivalTime;
}
public int getPriority() {
return priority;
}
public boolean hasMoreBurst() {
return Math.random() > 0.3;
}
public double getNextBurstTime() {
return (int) (Math.random() * (maxBurst - minBurst) + minBurst);
}
}
public class FIFOQueue implements Queue {
//put your name as the value of the signature.
String signature = "Name";
Node head = new Node(null);
Node pointer = head;
class Node {
Process process;
Node next;
Node(Process p) {
this.process = p;
this.next = null;
}
}
@Override
public void offer(Process p) {
// TODO Implement you code here
pointer.next = new Node(p);
pointer = pointer.next;
}
@Override
public Process poll() {
// TODO Implement you code here
if(isEmpty())
return null;
else{
Process item = head.next.process;
if(head.next == pointer){
head.next = null;
pointer = head;
}else{
head.next = head.next.next;
}
return item;
}
}
@Override
public boolean isEmpty() {
// TODO Implement you code here
return pointer == head;
}
@Override
public String getSignature() {
return signature;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
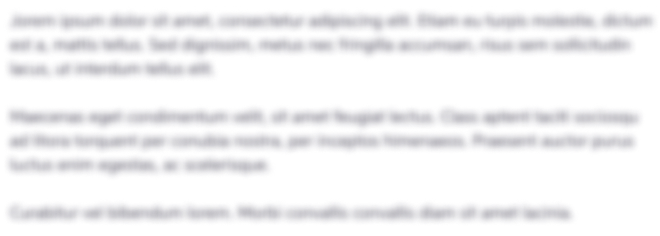
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started