Answered step by step
Verified Expert Solution
Question
1 Approved Answer
can you help me troubleshoot my c + + code? #include inventory.h #include NodeList.h #include exceptions.h #include #include #include Inventory::Inventory ( )
can you help me troubleshoot my c code? #include "inventory.h #include "NodeList.h #include "exceptions.h #include #include #include Inventory::Inventory Inventory::Inventoryint capacity Initialize capacity if needed Inventory::~Inventory Inventory::Inventoryconst Inventory& obj : vListobjvList Inventory& Inventory::operatorconst Inventory& rhs if this &rhs vList rhsvList; return this; bool Inventory::pushBackVehicle vehicle try vList.insertBackvehicle; return true; Assuming always successful for simplicity catch const std::exception& e std::cerr "Error inserting vehicle: ewhat std::endl; return false; void Inventory::sortList Bubble sort implementation for NodeList try for auto it vList.begin; it vList.end; it for auto it it; it vList.end; it if itit Assuming Vehicle comparison operators are defined Vehicle temp it; itit; it temp; catch const std::exception& e std::cerr "Error sorting list: ewhat std::endl; void Inventory::printList try printListRecursivevList.begin; catch const std::exception& e std::cerr "Error printing list: ewhat std::endl; void Inventory::printListRecursiveNodeList::Iterator it const if it vList.end return; std::cout it ; printListRecursiveit; void Inventory::printReservedList try printReservedListRecursivevList.begin; catch const std::exception& e std::cerr "Error printing reserved list: ewhat std::endl; void Inventory::printReservedListRecursiveNodeList::Iterator it const if it vList.end return; if itstatus std::cout it; printReservedListRecursiveit; void Inventory::printAvailableList try printAvailableListRecursivevList.begin; catch const std::exception& e std::cerr "Error printing available list: ewhat std::endl; void Inventory::printAvailableListRecursiveNodeList::Iterator it const if it vList.end return; if itstatus std::cout it; printAvailableListRecursiveit; bool Inventory::foundint seatsNo try return foundRecursivevList.begin seatsNo; catch const std::exception& e std::cerr "Error finding vehicle: ewhat std::endl; return false; bool Inventory::foundRecursiveNodeList::Iterator it int seatsNo const if it vList.end return false; if itstatus && itgetSeats seatsNo std::cout it; return true; return foundRecursiveit seatsNo; bool Inventory::reserveVehicleint vehicleID try int index checkIDvehicleID; if index throw InvalidVehicleIDException; if vListindexstatus throw VehicleAlreadyReservedException; vListindexreserve; return true; catch const InvalidVehicleIDException& e std::cerr ewhat std::endl; return false; catch const VehicleAlreadyReservedException& e std::cerr ewhat std::endl; return false; catch const std::exception& e std::cerr "Error reserving vehicle: ewhat std::endl; return false; bool Inventory::returnVehicleint vehicleID try int index checkIDvehicleID; if index throw InvalidVehicleIDException; if vListindexstatus throw std::runtimeerrorVehicle is already available."; vListindexunReserve; return true; catch const InvalidVehicleIDException& e std::cerr ewhat std::endl; return false; catch const std::exception& e std::cerr "Error returning vehicle: ewhat std::endl; return false; int Inventory::checkIDint vehicleID try int index ; for auto it vList.begin; it vList.end; itindex if itgetID vehicleID return index; throw InvalidVehicleIDException; catch const InvalidVehicleIDException& e std::cerr ewhat std::endl; return ;
can you help me troubleshoot my c code?
#include "inventory.h
#include "NodeList.h
#include "exceptions.h
#include
#include
#include
Inventory::Inventory
Inventory::Inventoryint capacity
Initialize capacity if needed
Inventory::~Inventory
Inventory::Inventoryconst Inventory& obj : vListobjvList
Inventory& Inventory::operatorconst Inventory& rhs
if this &rhs
vList rhsvList;
return this;
bool Inventory::pushBackVehicle vehicle
try
vList.insertBackvehicle;
return true; Assuming always successful for simplicity
catch const std::exception& e
std::cerr "Error inserting vehicle: ewhat std::endl;
return false;
void Inventory::sortList
Bubble sort implementation for NodeList
try
for auto it vList.begin; it vList.end; it
for auto it it; it vList.end; it
if itit Assuming Vehicle comparison operators are defined
Vehicle temp it;
itit;
it temp;
catch const std::exception& e
std::cerr "Error sorting list: ewhat std::endl;
void Inventory::printList
try
printListRecursivevList.begin;
catch const std::exception& e
std::cerr "Error printing list: ewhat std::endl;
void Inventory::printListRecursiveNodeList::Iterator it const
if it vList.end return;
std::cout it
;
printListRecursiveit;
void Inventory::printReservedList
try
printReservedListRecursivevList.begin;
catch const std::exception& e
std::cerr "Error printing reserved list: ewhat std::endl;
void Inventory::printReservedListRecursiveNodeList::Iterator it const
if it vList.end return;
if itstatus
std::cout it;
printReservedListRecursiveit;
void Inventory::printAvailableList
try
printAvailableListRecursivevList.begin;
catch const std::exception& e
std::cerr "Error printing available list: ewhat std::endl;
void Inventory::printAvailableListRecursiveNodeList::Iterator it const
if it vList.end return;
if itstatus
std::cout it;
printAvailableListRecursiveit;
bool Inventory::foundint seatsNo
try
return foundRecursivevList.begin seatsNo;
catch const std::exception& e
std::cerr "Error finding vehicle: ewhat std::endl;
return false;
bool Inventory::foundRecursiveNodeList::Iterator it int seatsNo const
if it vList.end return false;
if itstatus && itgetSeats seatsNo
std::cout it;
return true;
return foundRecursiveit seatsNo;
bool Inventory::reserveVehicleint vehicleID
try
int index checkIDvehicleID;
if index throw InvalidVehicleIDException;
if vListindexstatus throw VehicleAlreadyReservedException;
vListindexreserve;
return true;
catch const InvalidVehicleIDException& e
std::cerr ewhat std::endl;
return false;
catch const VehicleAlreadyReservedException& e
std::cerr ewhat std::endl;
return false;
catch const std::exception& e
std::cerr "Error reserving vehicle: ewhat std::endl;
return false;
bool Inventory::returnVehicleint vehicleID
try
int index checkIDvehicleID;
if index throw InvalidVehicleIDException;
if vListindexstatus throw std::runtimeerrorVehicle is already available.";
vListindexunReserve;
return true;
catch const InvalidVehicleIDException& e
std::cerr ewhat std::endl;
return false;
catch const std::exception& e
std::cerr "Error returning vehicle: ewhat std::endl;
return false;
int Inventory::checkIDint vehicleID
try
int index ;
for auto it vList.begin; it vList.end; itindex
if itgetID vehicleID
return index;
throw InvalidVehicleIDException;
catch const InvalidVehicleIDException& e
std::cerr ewhat std::endl;
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
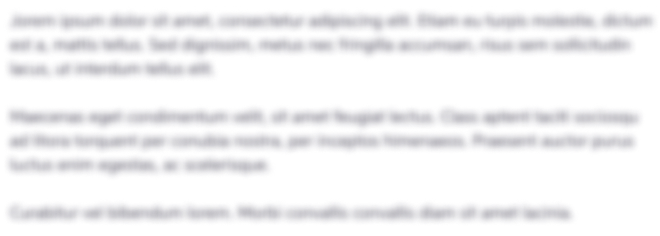
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started