Question
Can you run this and send me a screen shot of the input and output? Driver class import java.util.Scanner; public class Driver { public static
Can you run this and send me a screen shot of the input and output?
Driver class
import java.util.Scanner;
public class Driver {
public static void main(String[] args) { //Initialize Scanner Scanner sc = new Scanner(System.in);
//Get number of students int students = 0; do { System.out.print("Enter number of students participating in the events: "); try { students = sc.nextInt(); if (students < 1) { System.out.println("Invalid number of students entered."); sc.nextLine(); } } catch (Exception e) { System.out.println("Invalid number of students entered."); sc.nextLine(); } } while (students < 1); sc.nextLine(); //I'm going to assume you want to store many student in physed class //I will use an array of objects to store them //Initialize PhysEd class with int parameter //PhysEd pe = new PhysEd(students); //declaring an array of PhysEd PhysEd[] arr; //Allocate memory for students(int) objects //of type PhysEd arr = new PhysEd[students]; //Initialize the objects with a loop for(int i = 0 ; i < students ; i++) { System.out.println("Please enter name:"); sc.nextLine(); String n = sc.nextLine(); System.out.println("Please enter strength:"); int strgth = sc.nextInt(); System.out.println("Please enter speed:"); int spd = sc.nextInt(); System.out.println("Please enter intelligience:"); int intglnce = sc.nextInt(); arr[i] = new PhysEd(n, strgth, spd, intglnce); } //close Scanner sc.close(); //print students for(int i = 0 ; i < students ; i++) { System.out.println("Student "+i+": "); arr[i].printStudent(); } //need further clarification on the purpose of this section //tell me about it in the comments, will answer promptly // System.out.print("Rope Climb: "); // pe.sortByAttributes(1, 2, 3); // Sort by Str, Spd, and Int // // System.out.print("Freeze Tag: "); // pe.sortByAttributes(2, 3, 1); // Sort by Spd, Int, and Str // // System.out.print("Programming: "); // pe.sortByAttributes(3, 2, 1); // Sort by Int, Spd, and Str }
} PhysEd class
public class PhysEd {
private String name; private int strength; private int speed; private int intelligence;
//this is impossible cause you cant construct an object from a different class name //PhysEd class requires PhysEd() constructor //public Student(String name, int strength, int speed, int intelligence) { //this.name = name; //this.strength = strength; //this.speed = speed; //this.intelligence = intelligence; //} public PhysEd(String name, int strength, int speed, int intelligence) { this.name = name; this.strength = strength; this.speed = speed; this.intelligence = intelligence; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getStrength() { return strength; }
public void setStrength(int strength) { this.strength = strength; }
public int getSpeed() { return speed; }
public void setSpeed(int speed) { this.speed = speed; }
public int getIntelligence() { return intelligence; }
public void setIntelligence(int intelligence) { this.intelligence = intelligence; }
public void printStudent() { System.out.println("Student name: "+getName()+" Strength: "+getStrength()+" Speed: "+getSpeed()+" Intelligence: "+getIntelligence()+". "); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
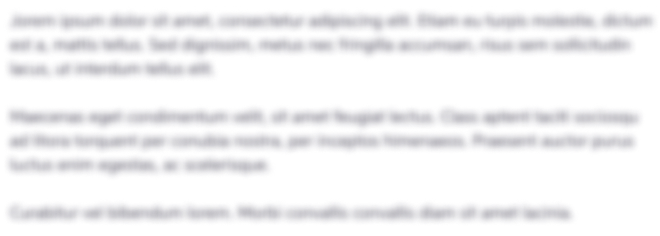
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started