Question
Can you update the given codes with their requirements: readerPrint (BufferPointer) o This function is intended to print the content of reader (in the
Can you update the given codes with their requirements:
❖ readerPrint (BufferPointer)
o This function is intended to print the content of reader (in the content field).
o Using the printf() library function the function prints character by character the contents of the
character reader to the standard output (stdout).
o You must use a loop that invokes readerGetChar () and checking the flags (END - End Of the
Reader) can print the content.
o Flag: During the reading, you can use END bit for checking if you had finished the process.
o Return value: The number of characters printed.
o DEFENSIVE PROGRAMMING: BufferPointer must exist (different from NULL).
Its code:
sofia_intg readerPrint(BufferPointer const readerPointer) {
sofia_intg cont = 0;
sofia_char c;
/* TO_DO: Defensive programming (including invalid chars) */
c = readerGetChar(readerPointer);
/* TO_DO: Check flag if buffer EOB has achieved */
while (cont < readerPointer->position.wrte) {
cont++;
printf("%c", c);
c = readerGetChar(readerPointer);
}
return cont;
}
❖ readerLoad (BufferPointer, fi)
o The function loads (reads) an input file specified by fi and put its content into a reader (the
field content).
▪ Note: The file is supposed to be a plain file (for instance, a source code).
o TIP_03: The function must use the standard function fgetc(fi) to read one character at a time
and the function readerAddChar () to add the character to the reader.
▪ The operation is repeated until the standard macro feof(fi) detects end-of-file on the
input file. The end-of-file character must not be added to the content of the reader.
▪ The standard macro feof(fi) can be used to detect end-of-file on the input file.
o Return value: The function returns the number of characters read from the file stream.
o Error Condition: If the current character cannot be added to the reader (readerAddChar ()
returns NULL. It is also necessary to use the ANSI C function ungetc() when if the function
gets an error.
▪ Note: This "ungetc()" operation is required because this latest char could not be
included into the reader.
o DEFENSIVE PROGRAMMING: BufferPointer must exist (different from NULL) and file must
be valid.
Note 14: About Load
This function is especially important to initialize the content of the reader: you need to be able to
manipulate all files (the only requirement is that you can read only ASCII chars) and then, you can
include them the content in the reader. If you are in a fixed mode or, eventually, the size of file is
too big, the content can be truncated. Remember that you do it char by char, invoking the
readerAddChar ().
Its code:
sofia_intg readerLoad(BufferPointer const readerPointer, FILE* const fileDescriptor) {
sofia_intg size = 0;
sofia_char c;
/* TO_DO: Defensive programming */
c = (sofia_char)fgetc(fileDescriptor);
while (!feof(fileDescriptor)) {
if (!readerAddChar(readerPointer, c)) {
ungetc(c, fileDescriptor);
return READER_ERROR;
}
c = (char)fgetc(fileDescriptor);
size++;
}
/* TO_DO: Defensive programming */
return size;
}
❖ readerRecover (BufferPointer)
o The function sets both read and mark offset to 0, so that the reader can be reread again.
o Return value: Boolean value defined by your language.
o DEFENSIVE PROGRAMMING: BufferPointer must exist (different from NULL).
Its code:
sofia_boln readerRecover(BufferPointer const readerPointer) {
/* TO_DO: Defensive programming */
/* TO_DO: Recover positions */
readerPointer->position.read = 0;
return SOFIA_TRUE;
}
❖ readerRetract (BufferPointer)
o The function simulates the operation to "unread" one char from the reader.
▪ It is a logical function that decrements read offset by 1.
▪ It means that it is required to be sure that read is positive.
o Return value: Boolean value defined by your language.
o DEFENSIVE PROGRAMMING: BufferPointer must exist (different from NULL).
Its code:
sofia_boln readerRetract(BufferPointer const readerPointer) {
/* TO_DO: Defensive programming */
/* TO_DO: Retract (return 1 pos read) */
return SOFIA_TRUE;
}
❖ readerRestore (BufferPointer)
o The function sets read offset to the value of the current mark offset.
▪ It will be used when it is necessary to "undo" several reading operations (and, for this
reason, mark offset is used).
o Return value: Boolean value defined by your language.
o DEFENSIVE PROGRAMMING: BufferPointer must exist (different from NULL).
Its code:
sofia_boln readerRestore(BufferPointer const readerPointer) {
/* TO_DO: Defensive programming */
/* TO_DO: Restore positions (read/mark) */
readerPointer->position.read = readerPointer->position.mark;
return SOFIA_TRUE;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
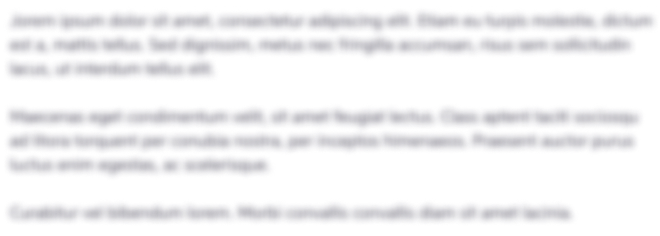
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started