Question
CarLot Continued Sophie, Sally and Jack are about to open for business. Their CarLot class needs some enhancements, however. Add to our previous CarLot the
CarLot Continued
Sophie, Sally and Jack are about to open for business. Their CarLot class needs some enhancements, however. Add to our previous CarLot the following methods:
Accessors
Car getCarHighestMilage() returns the Car with the highest mileage in the CarLot. If the CarLot is empty, return null.
int getTotalMiles() returns the total mileage of all cars on the CarLot
modify toString to include the above information, but don't include the sorted-by-MPG list.
ArrayList getSortedByMPG() returns a new ArrayList that is ordered by MPG (highest MPG first). The returned ArrayList is empty if the CarLot is empty. Note that this is an accessor; the method must not modify the instance variable in any way. Modify the SelectionSort code from the book to perform the sort.
Test Code
Enhance CarLotMain to add the new information. For example, after all Cars have been entered, print the CarLot sorted by MPG .
Grading Elements
-getCarHighestMilage, getTotalMiles are accessors, have correct return types and signatures.
-getCarHighestMilage, getTotalMiles return the expected values
-getSortedByMPG returns a copy of the CarLot inventory that is sorted appropriately; it does not change the CarLot instance variable.
toString enhanced to include new information
-CarLotMain enhanced to report new information and print the sorted-by-MPG list after all cars have been entered.
CarTest.java
import static org.junit.Assert.*; // note: all the new String(new String("xyz") // is to flush out anyone using == to compare String objects import java.util.HashMap; import java.util.Map; import org.junit.Test; public class CarTest { public static final Car MID_EVERYTHING = new Car("MID_EVERYTHING", 1000, 20, 3000, 4000); public static final Car HI_EVERYTHING = new Car("HI_EVERYTHING", 20000, 2, 300, 40000); public static final Car LO_EVERYTHING = new Car("LO_EVERYTHING", 10000, 2, 300, 400); static final Car[] CAR_ARRAY = { MID_EVERYTHING, HI_EVERYTHING, LO_EVERYTHING, }; static MapmakeCars() { Map cars = new HashMap(); /ames are weird because of historical reasons Car MID_EVERYTHING = new Car("MID_EVERYTHING", 1000, 20, 3000, 4000); Car HI_EVERYTHING = new Car("HI_EVERYTHING", 20000, 2, 300, 40000); Car LO_EVERYTHING = new Car("LO_EVERYTHING", 100, 2, 300, 400); cars.put("MID_EVERYTHING", MID_EVERYTHING); cars.put("HI_EVERYTHING", HI_EVERYTHING); cars.put("LO_EVERYTHING", LO_EVERYTHING); return cars; } @Test public void testComparePrice0() { Car c0 = makeCars().get(new String("LO_EVERYTHING")); Car c1 = makeCars().get(new String("MID_EVERYTHING")); assertTrue(new String("comparePrice LO(MID) failed"), c0.comparePrice(c1) 0); } @Test public void testComparePrice2() { Car c0 = makeCars().get(new String("LO_EVERYTHING")); Car c1 = new Car("LO2_EVERYTHING", 10000, 2, 300, 400); assertTrue(new String("comparePrice LO2(LO) failed"), c1.comparePrice(c0) == 0); } @Test public void testAddMiles() { Car c0 = makeCars().get(new String("LO_EVERYTHING")); c0.addMiles(5); assertEquals(new String("addMiles LO_EVERYTHING failed"), 105, c0.getMilage()); } @Test public void testProfit0() { Car c0 = makeCars().get(new String("LO_EVERYTHING")); assertEquals(new String("unsold car has profit"), 0, c0.getProfit()); } @Test public void testProfit1() { Car c0 = makeCars().get(new String("LO_EVERYTHING")); c0.setSold(true); assertEquals(new String("sold care profit incorrect"), 400 - 300, c0.getProfit()); } @Test public void testGetMPG() { Car c0 = makeCars().get(new String("MID_EVERYTHING")); assertEquals(new String("getMPG MID_EVERYTHING failed"), 20, c0.getMPG(), .001); } @Test public void testCompareMPG0() { Car c0 = makeCars().get(new String("LO_EVERYTHING")); Car c1 = makeCars().get(new String("MID_EVERYTHING")); assertTrue(new String("compareMiles MID_EVERYTHING has lower MPG than LO_EVERYTHING "), c0.compareMPG(c1) 0); } @Test public void testCompareId1() { Car c0 = makeCars().get(new String("LO_EVERYTHING")); Car c1 = makeCars().get(new String("MID_EVERYTHING")); assertTrue(new String("compareMiles MID_EVERYTHING is lower than LO_EVERYTHING "), c0.compareId(c1) 0); } @Test public void testCompareMiles1() { Car c0 = makeCars().get(new String("LO_EVERYTHING")); Car c1 = makeCars().get(new String("MID_EVERYTHING")); assertTrue(new String("compareMiles MID_EVERYTHING is lower than LO_EVERYTHING "), c0.compareMiles(c1) 0); } }
CarLotTest.java
import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import static org.junit.Assert.assertFalse; import static org.junit.Assert.fail; import java.util.Map; import org.junit.Test; import java.util.ArrayList; /ote: all the new String(new String("xyz") //is to flush out anyone using == to compare String objects public class CarLotTest { @Test public void testFind() { CarLot fl = new CarLot(); for (Car c : CarTest.CAR_ARRAY) { assertTrue(fl.add(c)); } Car c = fl.find( new String("MID_EVERYTHING")); assertEquals( new String("find failed"), "MID_EVERYTHING", c.getIdentifier()); } @Test public void testGetCarAverageMPG() { CarLot fl = new CarLot(); Mapall = CarTest.makeCars(); assertTrue(fl.add(all.get( new String(CarTest.MID_EVERYTHING.getIdentifier())))); assertTrue(fl.add(all.get( new String(CarTest.HI_EVERYTHING.getIdentifier())))); double mpg = fl.getCarAverageMPG(); assertEquals( new String("avg mpg bad for LO, HI"), 11, mpg, .001); } @Test public void testGetTotalMilage() { CarLot fl = new CarLot(); Map all = CarTest.makeCars(); assertTrue(fl.add(all.get( new String("MID_EVERYTHING")))); assertTrue(fl.add(all.get( new String("HI_EVERYTHING")))); int miles = fl.getTotalMiles(); assertEquals( new String("total miles bad for mhl, hmm"), 21000, miles); } @Test public void testGetCarBestMPG() { CarLot fl = new CarLot(); for (Car c : CarTest.CAR_ARRAY) { assertTrue(fl.add(c)); } Car best = fl.getCarBestMPG(); assertEquals( new String("best mpg bad"), new String("MID_EVERYTHING"), best.getIdentifier()); } @Test public void testGetCarHighestMilage() { CarLot fl = new CarLot(); for (Car c : CarTest.CAR_ARRAY) { assertTrue(fl.add(c)); } Car best = fl.getCarHighestMilage(); assertEquals( new String("highest milage bad"), new String("HI_EVERYTHING"), best.getIdentifier()); } @Test public void testtoString() { CarLot fl = new CarLot(); for (Car c : CarTest.CAR_ARRAY) { assertTrue(fl.add(c)); } System.out.println(fl); //assertEquals( new String("highest milage bad"), new String("HI_EVERYTHING"), best.getIdentifier()); } @Test public void testSortByMPG() { CarLot fl = new CarLot(); for (Car c : CarTest.CAR_ARRAY) { fl.add(c); } ArrayList sorted = fl.getSortedByMPG(); //System.out.println(sorted); if (sorted.size() != CarTest.CAR_ARRAY.length) fail( new String("sortedByMPG returned wrong number of elements")); for (int i = 0; i = next.getMPG()); } boolean sameOrder = true; int j = 0; while(sameOrder && j
How To Run Testing Software for lab
How to run the testing software for Unit 12 Carlot Lab Your instructor uses a Java Unit test program to test out your Carlot submission for Unit 12. This code is being provided to you so that you can run the tests prior to submission and correct any code shortcomings that may exist. Basically, CarLotTest.java and CarTest.java exercise your Carlot and Car classes. By providing known input into these classes, the output produced can be validated. Tests which fail will have a terse description of what was expected versus what was obtained. It doesn't tell you how to fix the problem..just that you have one. It's up to you to locate and fix the faulty code There's nothing particularly difficult with using Unit Tests, but it does have a couple of nuisances as described below: 1. Create a Java project in Eclipse and place Car.java, CarLot.java, CarTest.java, and CarLotTest.java in the src folder. Below is a screenshot of the Package Explorer taken immediately after relocating the files. vTest Unit 12 A JRE System Library [JavaSE-1.8] src ? (default package) > Carjava Carlot.java CarLotTest java CarTest,java ???.ypt Hleydy ???? 2. Note the red x's. That's because Eclipse doesn't know where to look for the Unit Test libraries. Right-click on the package name (Test Unit 12 in this case) and a dialog box appears like shown to the right. How to run the testing software for Unit 12 Carlot Lab Your instructor uses a Java Unit test program to test out your Carlot submission for Unit 12. This code is being provided to you so that you can run the tests prior to submission and correct any code shortcomings that may exist. Basically, CarLotTest.java and CarTest.java exercise your Carlot and Car classes. By providing known input into these classes, the output produced can be validated. Tests which fail will have a terse description of what was expected versus what was obtained. It doesn't tell you how to fix the problem..just that you have one. It's up to you to locate and fix the faulty code There's nothing particularly difficult with using Unit Tests, but it does have a couple of nuisances as described below: 1. Create a Java project in Eclipse and place Car.java, CarLot.java, CarTest.java, and CarLotTest.java in the src folder. Below is a screenshot of the Package Explorer taken immediately after relocating the files. vTest Unit 12 A JRE System Library [JavaSE-1.8] src ? (default package) > Carjava Carlot.java CarLotTest java CarTest,java ???.ypt Hleydy ???? 2. Note the red x's. That's because Eclipse doesn't know where to look for the Unit Test libraries. Right-click on the package name (Test Unit 12 in this case) and a dialog box appears like shown to the right
Step by Step Solution
There are 3 Steps involved in it
Step: 1
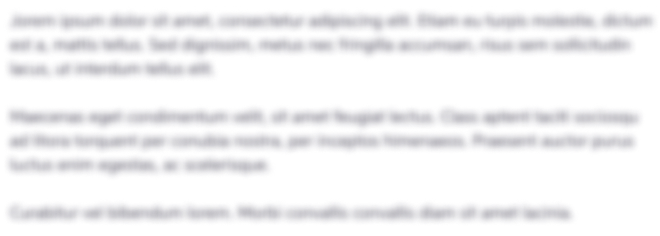
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started