Question
CartSystem: The purpose of the CartSystem class is to implement the logic related only to the cart. The following methods must be implemented: Return Type
CartSystem:
The purpose of theCartSystemclass is to implement the logic related only to the cart.
The following methods must be implemented:
Return Type | Description | Input Parameters |
void | display()-This method takas no parameter and displays every item in the Cart system, along with the sub-total, tax, and total: sub-total= the total sum of the products of the price and quantity of each item in the cart (Sum (item's price * item's quantity)). tax= sub-total * 0.05 total= sub-total + tax Your output should be formatted as follows: The header "Cart:" followed by the flowing columns.
Each column must be properly labeled. Finally, you should output the Pre-tax Total, Tax, Total for the following format.
please see the example below. | None |
Sample Output:
Display Cart
AppSystem Inventory:
Name | Description | Price | Quantity | Sub Total |
pizza | very cheesy | 12.30 | 1 | 12.30 |
salad | cobb salad | 15.50 | 1 | 15.50 |
Pre-tax Total | 27.80 |
Tax | 1.39 |
Total | 29.19 |
package com.example; public class CartSystem extends TheSystem { CartSystem() { } @Override public void display() { // Your code here } }
package com.example; public class Item{ private String itemName; private String itemDesc; private Double itemPrice; private Integer quantity; private Integer availableQuantity; public Item() { this.quantity=1; } public Item(String itemName, String itemDesc, Double itemPrice, Integer availableQuantity) { this.itemName = itemName; this.itemDesc = itemDesc; this.itemPrice = itemPrice; this.availableQuantity = availableQuantity; } public String getItemName() { return itemName; } public void setItemName(String itemName) { this.itemName = itemName; } public String getItemDesc() { return itemDesc; } public void setItemDesc(String itemDesc) { this.itemDesc = itemDesc; } public Double getItemPrice() { return itemPrice; } public void setItemPrice(Double itemPrice) { this.itemPrice = itemPrice; } public Integer getQuantity() { return quantity; } public void setQuantity(Integer quantity) { this.quantity = quantity; } public Integer getAvailableQuantity() { return availableQuantity; } public void setAvailableQuantity(Integer availableQuantity) { this.availableQuantity = availableQuantity; } }
package com.example; import java.io.IOException; import java.util.Scanner; public class MainEntryPoint { public static Scanner reader = new Scanner(System.in); public static void main(String[] args) throws IOException { AppSystem app = new AppSystem(); CartSystem cart = new CartSystem(); Integer choice = 0; while (choice != 7) { menu(); choice = reader.nextInt(); switch (choice) { case 1: Item item = new Item(); reader.nextLine(); System.out.print(" Enter the item name: "); item.setItemName(reader.nextLine()); System.out.print(" Enter a description for the item: "); item.setItemDesc(reader.nextLine()); System.out.print(" Enter the item's price: "); item.setItemPrice(reader.nextDouble()); System.out.print(" Enter the quantity available in the System: "); item.setAvailableQuantity(reader.nextInt()); System.out.println(item.getItemName()); if (app.add(item)) { System.out.println("Item successfully added"); } else { System.out.println("Try Again"); } break; case 2: app.display(); System.out.println("Enter the name of the item"); reader.nextLine(); String item_name = reader.nextLine(); Item item1 = app.getItemCollection().get(item_name); if(app.checkAvailability(item1)) if (cart.add(item1)) { app.reduceAvailableQuantity(item_name); System.out.println("Item successfully added"); } else { System.out.println("Invalid or Unavailable Item, Please Try Again"); } ; break; case 3: cart.display(); break; case 4: app.display(); break; case 5: cart.display(); System.out.println("Enter the name of the item"); reader.nextLine(); item_name = reader.nextLine(); if (cart.remove(item_name) != null) { System.out.println(item_name + " was removed from the cart"); } else { System.out.println("Invalid Item, Please Try Again"); } break; case 6: app.display(); System.out.println("Enter the name of the item"); reader.nextLine(); item_name = reader.nextLine(); if (app.remove(item_name) != null) { System.out.println(item_name + " was removed from the System"); if (cart.remove(item_name) != null) { System.out.println(item_name + " was also removed from the cart"); } } else { System.out.println("Invalid Item, Please Try Again"); } break; case 7: System.out.println(" Byyyeee!!"); break; } } reader.close(); } public static void menu() { System.out.println("Choose an action:"); System.out.println("1. Add item to System"); System.out.println("2. Add item to Cart"); System.out.println("3. Display Cart"); System.out.println("4. Display System"); System.out.println("5. Remove item from Cart"); System.out.println("6. Remove item from System"); System.out.println("7. Quit"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
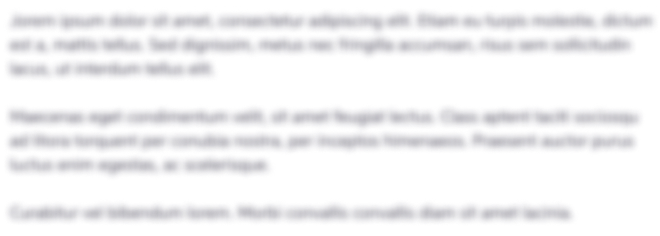
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started