Question
CashRegisterTester.java /** This program tests the CashRegister class. */ public class CashRegisterTester { public static void main(String[] args) { final double NICKEL_VALUE = 0.05; final
CashRegisterTester.java
/**
This program tests the CashRegister class.
*/
public class CashRegisterTester
{
public static void main(String[] args)
{
final double NICKEL_VALUE = 0.05;
final double DIME_VALUE = 0.1;
final double QUARTER_VALUE = 0.25;
CashRegister myRegister = new CashRegister();
myRegister.recordPurchase(0.82);
myRegister.enterPayment(3, new Coin(QUARTER_VALUE, "quarter"));
myRegister.enterPayment(2, new Coin(NICKEL_VALUE, "nickel"));
double myChange = myRegister.giveChange();
System.out.printf("Change: %.2f\n", myChange);
System.out.println("Expected:\nChange: 0.03");
}
}
CashRegister.java (has todo)
/**
A cash register totals up sales of several items purchased by a customer and computes change due.
See class CashRegisterTester to help you understand the methods in this class
*/
public class CashRegister
{
private double totalOfItemsPurchased;
private double payment;
/**
Constructs a cash register with no money in it.
*/
public CashRegister()
{
totalOfItemsPurchased = 0;
payment = 0;
}
/**
Records the sale of another item.
@param itemPrice the price of the item
*/
public void recordPurchase(double itemPrice)
{
//-----------Start below here. To do: approximate lines of code = 2
// add the price of the item to the total of items purchased so far
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
/**
Enters the payment received from the customer; should be called once
for each coin type
@param coinCount the number of coins (e.g. 3 dimes)
@param coinType the type of the coins in the payment (e.g. value of a coin)
*/
public void enterPayment(int coinCount, Coin coinType)
{
//-----------Start below here. To do: approximate lines of code = 1
// add to the payment variable the number of coins * the value of the given coins
// Hint: see class Coin
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
/**
Computes the change due and resets the cash register for the next customer.
@return the change due to the customer
*/
public double giveChange()
{
//-----------Start below here. To do: approximate lines of code = 4
// compute the change to return to the customer , then reset payment and totalOfItemsPurchased to 0 then return the change
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
}
Coin.java
/**
A coin with a monetary value.
*/
public class Coin
{
private double value;
private String name;
/**
Constructs a coin.
@param aValue the monetary value of the coin.
@param aName the name of the coin
*/
public Coin(double aValue, String aName)
{
value = aValue;
name = aName;
}
/**
Gets the coin value.
@return the value
*/
public double getValue()
{
return value;
}
/**
Gets the coin name.
@return the name
*/
public String getName()
{
return name;
}
}
A cash register totals up sales of several items purchased by a customer and computes change due. See class CashRegisterTester to help you understand the methods in this class Your job is to complete several methods in class CashRegister See the following files: * CashRegisterTester.java * CashRegister.java (has todo) * Coin.java Approximate total lines of code required: 7
Step by Step Solution
3.37 Rating (156 Votes )
There are 3 Steps involved in it
Step: 1
CashRegisterTesterjava This program tests the CashRegister class public class CashRegisterTester public static void mainString args final double NICKE...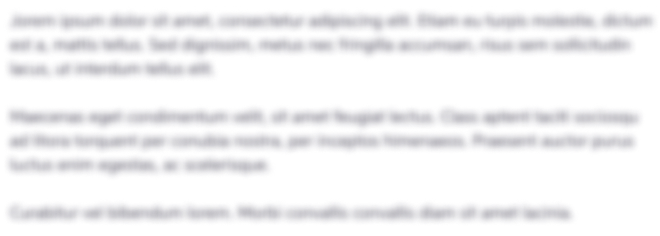
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started