Question
Challenge exercise Write a method to play every track in the track list exactly once in random order. Hint: One way to do this would
Challenge exercise Write a method to play every track in the track list exactly once in random order. Hint: One way to do this would be to shuffle the order of the tracks in the list - or, perhaps better, a copy of the list - and then play through from start to finish. Another way would be to make a copy of the list and then repeatedly choose a random track from the list, play it, and remove it from the list until the list is empty. Try to implement one of those approaches. If you try the first, how easy is it to shuffle the list so that it is genuinely in a new random order? Are there any library methods that could help with this? Please help me with the method. I have been able to write a method that plays the tracks in a random order, but I cannot get it to play each track once. I have been attempting to use an iterator. This needs to be from exercise 4.45 in Objects First with Java 6th edition. The solution in the Textbook Solutions is not correct. Below is the code that I have so far:
For the MusicOrganizer class:
import java.util.Random; import java.util.ArrayList; import java.util.*;
/** * A class to hold details of audio tracks. * Individual tracks may be played. * * @author David J. Barnes and Michael Klling * @version 2016.02.29 */ public class MusicOrganizer { // An ArrayList for storing music tracks. private ArrayList
/** * Create a MusicOrganizer */ public MusicOrganizer() { tracks = new ArrayList<>(); player = new MusicPlayer(); reader = new TrackReader(); readLibrary("../audio"); System.out.println("Music library loaded. " + getNumberOfTracks() + " tracks."); System.out.println(); }
/** * Add a track file to the collection. * @param filename The file name of the track to be added. */ public void addFile(String filename) { tracks.add(new Track(filename)); }
/** * Add a track to the collection. * @param track The track to be added. */ public void addTrack(Track track) { tracks.add(track); }
/** * Play a track in the collection. * @param index The index of the track to be played. */ public void playTrack(int index) { if(indexValid(index)) { Track track = tracks.get(index); player.startPlaying(track.getFilename()); System.out.println("Now playing: " + track.getArtist() + " - " + track.getTitle()); } }
/** * Return the number of tracks in the collection. * @return The number of tracks in the collection. */ public int getNumberOfTracks() { return tracks.size(); }
/** * List a track from the collection. * @param index The index of the track to be listed. */ public void listTrack(int index) { System.out.print("Track " + index + ": "); Track track = tracks.get(index); System.out.println(track.getDetails()); }
/** * Show a list of all the tracks in the collection. */ public void listAllTracks() { System.out.println("Track listing: ");
for(Track track : tracks) { System.out.println(track.getDetails()); } System.out.println(); } public void playRandomTrack() { Random rand = new Random(); int randInt = rand.nextInt(tracks.size()); if(indexValid(randInt)) { Track track = tracks.get(randInt); player.startPlaying(track.getFilename()); System.out.println("Now playing: " + track.getArtist() + "-" + track.getTitle()); } }
/** * List all tracks by the given artist. * @param artist The artist's name. */ public void listByArtist(String artist) { for(Track track : tracks) { if(track.getArtist().contains(artist)) { System.out.println(track.getDetails()); } } }
/** * Remove a track from the collection. * @param index The index of the track to be removed. */ public void removeTrack(int index) { if(indexValid(index)) { tracks.remove(index); } }
/** * Play the first track in the collection, if there is one. */ public void playFirst() { if(tracks.size() > 0) { player.startPlaying(tracks.get(0).getFilename()); } }
/** * Stop the player. */ public void stopPlaying() { player.stop(); }
/** * Determine whether the given index is valid for the collection. * Print an error message if it is not. * @param index The index to be checked. * @return true if the index is valid, false otherwise. */ private boolean indexValid(int index) { // The return value. // Set according to whether the index is valid or not. boolean valid;
if(index < 0) { System.out.println("Index cannot be negative: " + index); valid = false; } else if(index >= tracks.size()) { System.out.println("Index is too large: " + index); valid = false; } else { valid = true; } return valid; }
private void readLibrary(String folderName) { ArrayList
// Put all thetracks into the organizer. for(Track track : tempTracks) { addTrack(track); } } }
For the Track class:
/** * Store the details of a music track, * such as the artist, title, and file name. * * @author David J. Barnes and Michael Klling * @version 2016.02.29 */ public class Track { // The artist. private String artist; // The track's title. private String title; // Where the track is stored. private String filename; /** * Constructor for objects of class Track. * @param artist The track's artist. * @param title The track's title. * @param filename The track file. */ public Track(String artist, String title, String filename) { setDetails(artist, title, filename); } /** * Constructor for objects of class Track. * It is assumed that the file name cannot be * decoded to extract artist and title details. * @param filename The track file. */ public Track(String filename) { setDetails("unknown", "unknown", filename); } /** * Return the artist. * @return The artist. */ public String getArtist() { return artist; } /** * Return the title. * @return The title. */ public String getTitle() { return title; } /** * Return the file name. * @return The file name. */ public String getFilename() { return filename; } /** * Return details of the track: artist, title and file name. * @return The track's details. */ public String getDetails() { return artist + ": " + title + " (file: " + filename + ")"; } /** * Set details of the track. * @param artist The track's artist. * @param title The track's title. * @param filename The track file. */ private void setDetails(String artist, String title, String filename) { this.artist = artist; this.title = title; this.filename = filename; } }
For the TrackReader class
import java.io.File; import java.io.FilenameFilter; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.stream.Collectors;
/** * A helper class for our music application. This class can read files from the file system * from a given folder with a specified suffix. It will interpret the file name as artist/ * track title information. * * It is expected that file names of music tracks follow a standard format of artist name * and track name, separated by a dash. For example: TheBeatles-HereComesTheSun.mp3 * * @author David J. Barnes and Michael Klling * @version 2016.02.29 */ public class TrackReader { /** * Create the track reader, ready to read tracks from the music library folder. */ public TrackReader() { // Nothing to do here. } /** * Read music files from the given library folder * with the given suffix. * @param folder The folder to look for files. * @param suffix The suffix of the audio type. */ public ArrayList
/** * Try to decode details of the artist and the title * from the file name. * It is assumed that the details are in the form: * artist-title.mp3 * @param file The track file. * @return A Track containing the details. */ private Track decodeDetails(File file) { // The information needed. String artist = "unknown"; String title = "unknown"; String filename = file.getPath(); // Look for artist and title in the name of the file. String details = file.getName(); String[] parts = details.split("-"); if(parts.length == 2) { artist = parts[0]; String titlePart = parts[1]; // Remove a file-type suffix. parts = titlePart.split("\\."); if(parts.length >= 1) { title = parts[0]; } else { title = titlePart; } } return new Track(artist, title, filename); } }
For the MusicPlayer class:
import java.io.BufferedInputStream; import java.io.FileInputStream; import java.io.InputStream; import java.io.IOException; import javazoom.jl.decoder.JavaLayerException; import javazoom.jl.player.AudioDevice; import javazoom.jl.player.FactoryRegistry; import javazoom.jl.player.advanced.AdvancedPlayer;
/** * Provide basic playing of MP3 files via the javazoom library. * See http://www.javazoom.net/ * * @author David J. Barnes and Michael Klling. * @version 2016.02.29 */ public class MusicPlayer { // The current player. It might be null. private AdvancedPlayer player; /** * Constructor for objects of class MusicFilePlayer */ public MusicPlayer() { player = null; } /** * Play a part of the given file. * The method returns once it has finished playing. * @param filename The file to be played. */ public void playSample(String filename) { try { setupPlayer(filename); player.play(500); } catch(JavaLayerException e) { reportProblem(filename); } finally { killPlayer(); } } /** * Start playing the given audio file. * The method returns once the playing has been started. * @param filename The file to be played. */ public void startPlaying(final String filename) { try { setupPlayer(filename); Thread playerThread = new Thread() { public void run() { try { player.play(5000); } catch(JavaLayerException e) { reportProblem(filename); } finally { killPlayer(); } } }; playerThread.start(); } catch (Exception ex) { reportProblem(filename); } } public void stop() { killPlayer(); } /** * Set up the player ready to play the given file. * @param filename The name of the file to play. */ private void setupPlayer(String filename) { try { InputStream is = getInputStream(filename); player = new AdvancedPlayer(is, createAudioDevice()); } catch (IOException e) { reportProblem(filename); killPlayer(); } catch(JavaLayerException e) { reportProblem(filename); killPlayer(); } }
/** * Return an InputStream for the given file. * @param filename The file to be opened. * @throws IOException If the file cannot be opened. * @return An input stream for the file. */ private InputStream getInputStream(String filename) throws IOException { return new BufferedInputStream( new FileInputStream(filename)); }
/** * Create an audio device. * @throws JavaLayerException if the device cannot be created. * @return An audio device. */ private AudioDevice createAudioDevice() throws JavaLayerException { return FactoryRegistry.systemRegistry().createAudioDevice(); }
/** * Terminate the player, if there is one. */ private void killPlayer() { synchronized(this) { if(player != null) { player.stop(); player = null; } } } /** * Report a problem playing the given file. * @param filename The file being played. */ private void reportProblem(String filename) { System.out.println("There was a problem playing: " + filename); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
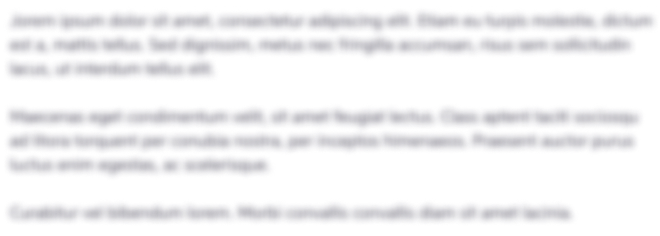
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started