Question
Changing People The file ChangingPeople.java contains a program that illustrates parameter passing. The program uses Person objects defined in the file Person.java. Do the following:
Changing People
The file ChangingPeople.java contains a program that illustrates parameter passing. The program uses Person objects defined in the file Person.java. Do the following:
Trace the execution of the program. Also show what is printed by the program.
Compile and run the program to see if your trace was correct.
Modify the changePeople method so that it does what the documentation says it does, that is, the two Person objects passed in as actual parameters are actually changed.
// *****************************************************************
// ChangingPeople.java
//
// Demonstrates parameter passing -- contains a method that should
// change to Person objects.
// ******************************************************************
public class ChangingPeople
{
// ---------------------------------------------------------
// Sets up two person objects, one integer, and one String
// object. These are sent to a method that should make
// some changes.
// ---------------------------------------------------------
public static void main (String[] args)
{
Person person1 = new Person ("Sally", 13);
Person person2 = new Person ("Sam", 15);
int age = 21;
String name = "Jill";
System.out.println (" Parameter Passing... Original values...");
System.out.println ("person1: " + person1);
System.out.println ("person2: " + person2);
System.out.println ("age: " + age + "\tname: " + name + " ");
changePeople (person1, person2, age, name);
System.out.println (" Values after calling changePeople...");
System.out.println ("person1: " + person1);
System.out.println ("person2: " + person2);
System.out.println ("age: " + age + "\tname: " + name + " ");
}
// -------------------------------------------------------------------
// Change the first actual parameter to "Jack - Age 101" and change
// the second actual parameter to be a person with the age and
// name given in the third and fourth parameters.
// -------------------------------------------------------------------
public static void changePeople (Person p1, Person p2, int age, String name)
{
System.out.println (" Inside changePeople... Original parameters...");
System.out.println ("person1: " + p1);
System.out.println ("person2: " + p2);
System.out.println ("age: " + age + "\tname: " + name + " ");
// Make changes
Person p3 = new Person (name, age);
p2 = p3;
name = "Jack";
age = 101;
p1.changeName (name);
p1.changeAge (age);
// Print changes
System.out.println (" Inside changePeople... Changed values...");
System.out.println ("person1: " + p1);
System.out.println ("person2: " + p2);
System.out.println ("age: " + age + "\tname: " + name + " ");
}
}
// ************************************************************
// Person.java
//
// A simple class representing a person.
// ************************************************************
public class Person
{
private String name;
private int age;
// ----------------------------------------------------------
// Sets up a Person object with the given name and age.
// ----------------------------------------------------------
public Person (String name, int age)
{
this.name = name;
this.age = age;
}
// ----------------------------------------------------------
// Changes the name of the Person to the parameter newName.
// ----------------------------------------------------------
public void changeName(String newName)
{
name = newName;
}
// ----------------------------------------------------------
// Changes the age of the Person to the parameter newAge.
// ----------------------------------------------------------
public void changeAge (int newAge)
{
age = newAge;
}
// ----------------------------------------------------------
// Returns the person's name and age as a string.
// ----------------------------------------------------------
public String toString()
{
return name + " - Age " + age;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
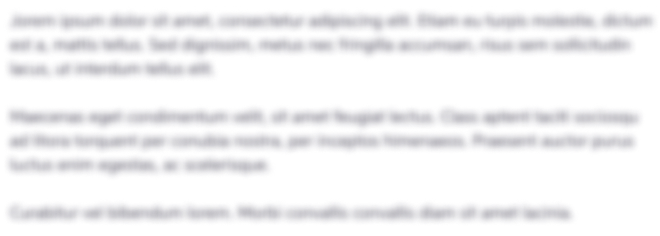
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started