Question
CHARACTER STRINGS Write a C program that will calculate the gross pay of a set of employees. The program should prompt the user to enter
CHARACTER STRINGS
Write a C program that will calculate the gross pay of a set of employees.
The program should prompt the user to enter the number of hours each employee worked. When prompted, key in the hours shown below.
The program determines the overtime hours (anything over 40 hours), the gross pay and then outputs a table in the following format.
Column alignment, leading zeros in Clock#, and zero suppression in float fields is important.
Continue to incorporate functions into your program design.
Use 1.5 as the overtime pay factor.
This week, adding a Total and Average row is no longer optional, its required for this assignment:
a) Add aTotalrow at the end to sum up the wage, hours,ot, and gross columns
b) Add anAveragerow to print out the average of the wage, hours,ot, and gross columns
--------------------------------------------------------- Name Clock# Wage Hours OT Gross --------------------------------------------------------- Connie Cobol 098401 10.60 51.0 11.0 598.90 Mary Apl 526488 9.75 42.5 2.5 426.56 Frank Fortran 765349 10.50 37.0 0.0 388.50 Jeff Ada 034645 12.25 45.0 5.0 581.88 Anton Pascal 127615 10.00 40.0 0.0 400.00 --------------------------------------------------------- Total: 53.10 215.5 18.5 2395.84 Average: 10.62 43.1 3.7 479.17
You should implement this program using a structure similar to the suggested one below to store the information for each employee. Feel free to tweak it if you wish. For example, its OK to have a first and last name member instead of just a name member, and if you want to use different types, that is OK as well.
struct employee { char name [20]; long id_number; float wage; float hours; float overtime; float gross; };
Use the following information to initialize your data.
Connie Cobol 98401 10.60 Mary Apl 526488 9.75 Frank Fortran 765349 10.50 Jeff Ada 34645 12.25 Anton Pascal 127615 10.00
Create an array of structures with 5 elements, each being of typestructemployee.
Initialize the array with the data provided and reference the elements of the array with the appropriate subscripts.
IntermediateOptionalChallenge
Add two more rows to calculate theminimumandmaximumvalues:
a) Calculate and print theminimumwage, hours,ot, and gross values
b) Calculate and print themaximumwage, hours,ot, and gross values
--------------------------------------------------------- Name Clock# Wage Hours OT Gross --------------------------------------------------------- Connie Cobol 098401 10.60 51.0 11.0 598.90 Mary Apl 526488 9.75 42.5 2.5 426.56 Frank Fortran 765349 10.50 37.0 0.0 388.50 Jeff Ada 034645 12.25 45.0 5.0 581.88 Anton Pascal 127615 10.00 40.0 0.0 400.00 --------------------------------------------------------- Total: 53.10 215.5 18.5 2395.84 Average: 10.62 43.1 3.7 479.17 Minimum: 9.75 37.0 0.0 388.50 Maximum: 12.25 51.0 11.0 598.90
AdvancedOptionalChallenge
For those of you that want the ultimate challenge this week, do the intermediate challengeandadd the following advancedchallenge below:
Define a structure type calledstructnameto store the first name, middleinitial, and last name of each employee instead of storing it all in one string.Youremployee structurewill not look something like this:
struct employee { struct name employee_name; long id_number; float wage; float hours; float overtime; float gross; };
You are welcome to addadditional membersto your employee structure type, such as those suggested in homework assignment 5.Do not initialize your array of structures, insteadpromptfor the following information as input to your program:
Connie J Cobol 98401 10.60 Mary P Apl 526488 9.75 Frank K Fortran 765349 10.50 Jeff B Ada 34645 12.25 Anton T Pascal 127615 10.00
When printing, print just like you did with the intermediate challenge, but display employee names in the following format:
<Last Name>, <First Name> <Middle Initial>
Your output would now look like this:
--------------------------------------------------------- Name Clock# Wage Hours OT Gross ---------------------------------------------------------- Cobol, Connie J 098401 10.60 51.0 11.0 598.90 Apl, Mary P 526488 9.75 42.5 2.5 426.56 Fortran, Frank K 765349 10.50 37.0 0.0 388.50 Ada, Jeff B 034645 12.25 45.0 5.0 581.88 Pascal, Anton T 127615 10.00 40.0 0.0 400.00 ---------------------------------------------------------- Total: 53.10 215.5 18.5 2395.84 Average: 10.62 43.1 3.7 479.17 Minimum: 9.75 37.0 0.0 388.50 Maximum: 12.25 51.0 11.0 598.90
Do this using the program below to start:
- #include
- /* constants to use */
- #define SIZE 5 /* number of employees to process */
- #define OT_RATE 1.5
- #define STD_HOURS 40
- /*Global Structure Declaration template */
- struct employee
- {
- int id_number; /* or just int id_number; */
- float wage;
- float hours;
- float overtime;
- float gross;
- };
- int main()
- {
- struct employee Emp[SIZE];
- /*Initializing number for variables named given employee by Emp*/
- /*Static way of defining both clock Number and Wages
- Initialized the clock and wage values in array declaration */
- Emp[0].id_number = 98401;
- Emp[1].id_number = 526488;
- Emp[2].id_number = 765349;
- Emp[3].id_number = 34645;
- Emp[4].id_number = 127615;
- Emp[0].wage = 10.60;
- Emp[1].wage = 9.75;
- Emp[2].wage = 10.50;
- Emp[3].wage = 12.25;
- Emp[4].wage = 8.35;
- float standard_pay; /*standard_pay = (hours*wage)*/
- float overtime_pay; /* ovetime_pay = overtimehours*((1.5)*(wage))*/
- int count; /* loop index */
- for (count = 0; count < SIZE; count++) /* Process each employee one at a time */
- {
- /* Read in hours worked for employee */
- printf("%d - Enter the number of hours each employee worked: ", count+1);
- scanf("%f",&Emp[count].hours);
- /* calculate overtime hours and gross pay for employee */
- if (Emp[count].hours <= STD_HOURS && Emp[count].hours >= 0)
- {
- standard_pay = (Emp[count].hours*Emp[count].wage);
- overtime_pay=0;
- Emp[count].overtime = 0;
- Emp[count].gross = (standard_pay + overtime_pay);
- }
- else
- {
- Emp[count].overtime = Emp[count].hours - STD_HOURS;
- overtime_pay = (Emp[count].wage * OT_RATE) * Emp[count].overtime;
- standard_pay = (Emp[count].wage * STD_HOURS);
- Emp[count].gross = (standard_pay + overtime_pay);
- }
- }
- /* Print a nice table header */
- //printf (" Leonardo Gutierrez, C Programming, Homework Assignment 5 ");
- //printf("---------------------------------------------------------------");
- printf(" Clock #\t| Wage\t| Hours\t| OT\t| Gross ");
- printf("--------|-------|-------|-------|-------- ");
- /* Access each employee and print to screen or file */
- for (count = 0; count < SIZE; count++)
- {
- /* print employee information from your arrays */
- printf("|%06d\t| %5.2f\t|%5.1f\t| %.1f\t|%7.2f ", Emp[count].id_number,
- Emp[count].wage, Emp[count].hours, Emp[count].overtime, Emp[count].gross);
- }
- printf("--------|-------|-------|-------|-------- ");
- return 0;
- }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
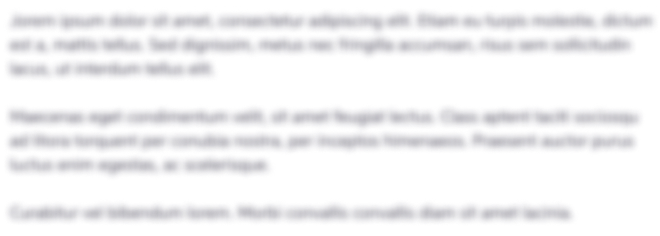
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started