Question
cheating hang man c++ The game hangman is a word guessing game. A word is selected and a hint is constructed from the word by
cheating hang man c++
The game hangman is a word guessing game. A word is selected and a hint is constructed from the word by replacing all non-guessed letters with underscores. At each turn the player guesses a letter. If that letter is present in the word, the letters that match it in the word are revealed. If not, then the number of incorrect guesses increases.
A player may only guess a single letter at a time, and can not repeat a guess.
The game ends by two conditions. First, when the number of incorrect guesses is at least 5, the player loses. Second, when the player has guessed all of the letters in the word, in which case the player wins.
The twist
While you will be writing a program to play Hangman, we want to make it cheat as much as possible, while still presenting only correct information. Instead of selecting a word to start, we will have a list of words. Whenever the player guesses a word, the list of remaining words is partitioned based upon the information that would be revealed about the word. Then the partition with the maximum size is selected as our new word list. By doing this we ensure that at each step there is a maximal amount of possible words that the player needs to guess from.
The code
You are strongly encouraged to leverage the C++ Standard Library as much as possible. Part of the point of this assignment is to learn some of the containers provided by the library which in turn make writing a program in C++ faster.
Three containers that will prove very useful for this assignment are std::map (Links to an external site.), std::string (Links to an external site.), and std::vector (Links to an external site.).
In particular the std::map provides a key, value interface to the data which allows us to partition a word list. In this program, you likely want a std::map
You should use as a source of words, a word list. This should be a file that simply contains a long list of words. If you search around for word lists, they are not hard to find, for example one can be here (Links to an external site.). If you are on Linux or MacOS, you can find a list at /usr/share/dict/words. When testing, you probably do not want to use the whole word list as it will be very difficult to verify correct behavior. Instead create words lists of your own that contain the patterns you are looking for, don't concern yourself with them being actual words.
You should also have a class to contain the current word list, guessed letters, and partitioning algorithm.
The general flow of your program should be:
- Read in the dictionary file and filter for a specific word length
- Prompt the user for a guess
- Partition the word list based upon the guessed letters so far, revealing the mask that has the most ambiguity.
- Prompt the user for another guess and repeat.
Testing
Testing your code is important! Some example cases you may use include:
Masking:
If you have the word zymurgy and the letters guessed are "rstne", its masked form would be _______. If the letters guessed were "ym", its masked form would be _ym___y.
Partitioning:
If you start with the word list {abcd, abce, abdg}, have already guessed ab, leading to the revealed ab__ and then guess c, the resulting partitions should be abc_ : {abcd, abce} and ab__ : {abdg}. The decision should then be to reveal abc_ and make the word list become {abcd, abce}. Note that to collect all the words for a given mask together is where the std::map
Sample Output
User input is in bold
What word length do you want? (<1 or non-numeric initiates self-tests) 5 There are 43 words of length 5 ------------------------------------------ The possible words are: aping awash based beers beige bided blued bossy chose cubit dirks fains false fence fishy fours gowns inked khaki knoll knows mains moody noise pepsi serfs shied skits snowy spent sunny swirl taffy timid twerp until viler wayne wheel where writs yelps zeros There are 43 words still possible. You have 5 incorrect guesses left. Current hint: _____ Guessed letters: What letter do you want to guess? a You have guessed 'a' The partite sets from your guess are: _____: beers, beige, bided, blued, bossy, chose, cubit, dirks, fence, fishy, fours, gowns, inked, knoll, knows, moody, noise, pepsi, serfs, shied, skits, snowy, spent, sunny, swirl, timid, twerp, until, viler, wheel, where, writs, yelps, zeros, __a__: khaki, _a___: based, fains, false, mains, taffy, wayne, a____: aping, a_a__: awash, Unfortunately 'a' is not in the word ------------------------------------------ The possible words are: beers beige bided blued bossy chose cubit dirks fence fishy fours gowns inked knoll knows moody noise pepsi serfs shied skits snowy spent sunny swirl timid twerp until viler wheel where writs yelps zeros There are 34 words still possible. You have 4 incorrect guesses left. Current hint: _____ Guessed letters: a What letter do you want to guess? e You have guessed 'e' The partite sets from your guess are: _____: bossy, cubit, dirks, fishy, fours, gowns, knoll, knows, moody, skits, snowy, sunny, swirl, timid, until, writs, ____e: chose, noise, ___e_: bided, blued, inked, shied, viler, __e__: spent, twerp, __e_e: where, __ee_: wheel, _e___: pepsi, serfs, yelps, zeros, _e__e: beige, fence, _ee__: beers, Unfortunately 'e' is not in the word ------------------------------------------ The possible words are: bossy cubit dirks fishy fours gowns knoll knows moody skits snowy sunny swirl timid until writs There are 16 words still possible. You have 3 incorrect guesses left. Current hint: _____ Guessed letters: ae What letter do you want to guess? i You have guessed 'i' The partite sets from your guess are: _____: bossy, fours, gowns, knoll, knows, moody, snowy, sunny, ___i_: cubit, until, __i__: skits, swirl, writs, _i___: dirks, fishy, _i_i_: timid, Unfortunately 'i' is not in the word ------------------------------------------ The possible words are: bossy fours gowns knoll knows moody snowy sunny There are 8 words still possible. You have 2 incorrect guesses left. Current hint: _____ Guessed letters: aei What letter do you want to guess? o You have guessed 'o' The partite sets from your guess are: _____: sunny, __o__: knoll, knows, snowy, _o___: bossy, fours, gowns, _oo__: moody, You have guessed correctly! ------------------------------------------ The possible words are: knoll knows snowy There are 3 words still possible. You have 2 incorrect guesses left. Current hint: __o__ Guessed letters: aeio What letter do you want to guess? k You have guessed 'k' The partite sets from your guess are: __o__: snowy, k_o__: knoll, knows, You have guessed correctly! ------------------------------------------ The possible words are: knoll knows There are 2 words still possible. You have 2 incorrect guesses left. Current hint: k_o__ Guessed letters: aeiok What letter do you want to guess? n You have guessed 'n' The partite sets from your guess are: kno__: knoll, knows, You have guessed correctly! ------------------------------------------ The possible words are: knoll knows There are 2 words still possible. You have 2 incorrect guesses left. Current hint: kno__ Guessed letters: aeiokn What letter do you want to guess? l You have guessed 'l' The partite sets from your guess are: kno__: knows, knoll: knoll, Unfortunately 'l' is not in the word ------------------------------------------ The possible words are: knows There are 1 words still possible. You have 1 incorrect guesses left. Current hint: kno__ Guessed letters: aeioknl What letter do you want to guess? w You have guessed 'w' The partite sets from your guess are: know_: knows, You have guessed correctly! ------------------------------------------ The possible words are: knows There are 1 words still possible. You have 1 incorrect guesses left. Current hint: know_ Guessed letters: aeioknlw What letter do you want to guess? s You have guessed 's' The partite sets from your guess are: knows: knows, You have guessed correctly! You win! The word was knows
Tips
- The exact formatting of the game is not important, but it is worthwhile to build in support to show the current word list, and partitions to understand how the game is being played.
- When creating your test cases, feel free to use fake words. It is much easier to type characters that have the patterns you are looking for than it is to find words with those patterns.
PreviousNext
Step by Step Solution
There are 3 Steps involved in it
Step: 1
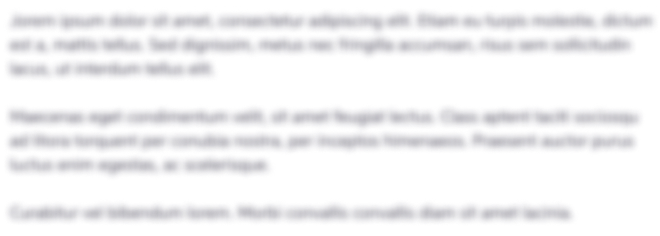
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started