Objective Create a calculator for positive, single-digit integers for basic math operations: add, subtract, multiply, divide How to use command line arguments. How to use
Objective
Create a calculator for positive, single-digit integers for basic math operations: add, subtract, multiply, divide
How to use command line arguments.
How to use an array of pointers to functions.
Related SLO
Develop properly structured multifile programs with automatic compilation.
Implement arrays and pointers in C.
InstructionsCreate a makefile named makefile that will automatically compile, link, and create an executable for this assignment.
This assignment will not require any external files such as getdouble.c, getdouble.h
Create a program named LastnameFirstname14.c, that does the following:Create the following 4 custom functions given their prototypes and descriptions (they are short and simple):
Declare function prototypes above main and place function definitions below main.int add(int, int);
Return the addition of the parameters: first parameter + second parameter
int sub(int, int);
Return the subtraction of the parameters: first parameter - second parameter
int mul(int, int);
Return the multiplication of the parameters: first parameter * second parameter
int div2(int, int);
Return the division of the parameters: first parameter / second parameter
The function is named div2 as to not conflict with the div() function already defined in stdlib.h
Ensure the main() function definition allows the use of command line arguments.
See the main() function definition in the example program: command.c
All input will come from command line arguments. The program will not ask the user for the digits. Do NOT use scanf(), getchar(), or getdouble() or any other function to get user input while the program is running.
Perform the following error checks on the command line arguments.
Note #1: The 0th command line argument is the name of the executable such as ./program
Note #2: All command line arguments are stored as chars, similar to how Java stores arguments as Strings.
Ensure there are exactly 4 command line arguments. If not, print an error message and end the program.
Ensure the 1st command line argument is a character between '0' and '9', inclusive. If not, print an error message and end the program.
That is, argv[1][0] is a character between '0' and '9', inclusive.
Ensure the 3rd command line argument is a character between '0' and '9', inclusive. If not, print an error message and end the program.
That is, argv[3][0] is a character between '0' and '9', inclusive.
Ensure the 2nd command line argument is a valid math operation symbol. If not, print an error message and end the program.
Note: The asterisk '*' character has a special use in Unix/Linux systems as the wildcard, therefore we will use the period '.' instead to represent multiplication.
Valid math operators are the characters: '+', '-', '.', '/'
Ensure that argv[2][0] is a plus, minus, period, or slash character.
If there was anything wrong with the program arguments, you will need to ensure the program ends. If you do not ensure the program ends, then the program may crash/seg. fault later on.
To end the program, you may return 1; or exit(1);
The exit function is defined in be sure to include it at the top if you plan to use it.
Because there are multiple program arguments, you must tell the user specifically which argument was wrong by printing it to the user, see Example Output. Do NOT just print a generic message such as "Error, not an integer" or "Error, invalid symbol".
After performing the error checks, convert the two digit characters from the 1st and 3rd command line arguments to integers.
To convert, subtract '0' and store the result in an integer variable.
For example: int number1 = argv[1][0] - '0';
Declare an array of functions so that the 0th element is the add function, the 1st element is NULL, the 2nd is the sub function, the 3rd is mul, and the 4th is div2.
You may use the following:
int (*arithmeticOperations[5])(int, int) = {add, NULL, sub, mul, div2};
Although the program only performs 4 math operations, the array will have 5 indexes with [1] being NULL. This is to be clever with array access and avoid comparisons.
Examples of array of functions: functionpointer.c and menu.c
Convert the math operation character from the command line arguments to an integer. The integer will be the index in arithmeticOperations.
To convert, subtract the plus '+' character from the command line argument.
For example:
int index = argv[2][0] - '+';
Using an ASCII table, you will find the following:
When the operation is '+' the index will be 0
'-' will give you an index of 2
'.' will give you an index of 3
'/' will give you an index of 4
Given this information, you can construct the array of functions.
Use a single printf() statement to output the computation and result of the math operation. Use the array of functions and calculated index to call each function. The arguments to the function call are the two numbers converted from the command line arguments.
Do NOT use if-statements or a switch-case to determine which math operation to call. The math operation to call in the array of functions is the calculated index.
Use the Example Output to format your output the same way.
All output should clearly display the operands, operator, and result.
Be sure to have a program description at the top and in-line comments.
Be clear with your comments and output to the user, so I can understand what you're program is doing.
Be sure to have read through the assignment policy for this class.
Example Output
% make % ./programError: 4 command line arguments are expected, 1 present. % ./program 2Error: 4 command line arguments are expected, 2 present.% ./program 2 +Error: 4 command line arguments are expected, 3 present.% ./program B + 3Error: 'B' is not an integer between 0 - 9. % ./program 9 ! 3Error: '!' is not a valid math operation. Use: + - . / % ./program 9 + wError: 'w' is not an integer between 0 - 9. % ./program 9 + 9 9Error: 4 command line arguments are expected, 5 present. % ./program 9 + 59 + 5 = 14 % ./program 9 . 59 . 5 = 45 % ./program 9 - 59 - 5 = 4 % ./program 8 / 38 / 3 = 2
Notes
This calculator is for single digits only, using numbers that are 2 or more digits will not work properly.
/* Function Prototypes */
int add(int, int);
int sub(int, int);
int mul(int, int);
int div2(int, int);
Step by Step Solution
3.58 Rating (180 Votes )
There are 3 Steps involved in it
Step: 1
Following are the steps to implement the required calculator 1Define four functions to perform arithmetic operations addition subtraction multiplicati...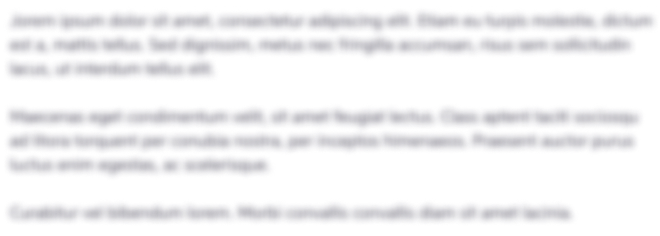
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started