Question
Check the sample output to know how the results need to be printed. Read every instruction carefully and follow them strictly. Do not change the
- Check the sample output to know how the results need to be printed.
- Read every instruction carefully and follow them strictly.
- Do not change the name of the attributes, and methods given below.
- You are required to validate several values that user will enter through the console. Write your code in the Driver class in such a way that it passes all the possible test cases.
- Mobile provider names should be a string. Three provider names: AT&T, TMOBILE, and VERIZON
- Users can't be zero or between 2 and 5 in case of several users.
- User cannot be a member of the (1) Mobile Alliance, (2) First Time User and (3) has Coupon at a time. You can ONLY have discount (1), (2) or (3).
- Attributes are given in this table. You must use the names given here, with the same capitalization as shown here. All attributes must be private.
Attribute | Type | Attribute Description |
providerName | String | Provider Name |
numOfUsers | int | Number of users |
isMemberOfMobileAlliance | Boolean | Does the user have a membership with the mobile alliance? |
isFirstTimeUser | char | Is the first time? "Y" or "N" (upper-case) |
hasCoupon | String | Does the user have a coupon? "YES", or "No" (upper-case, lower-case or any combination of upper-case and lower-case) |
COUPON | double | Must be declared as a constant and initialized with 5.00 ($5 coupon) |
SALETAXES | double | Must be declared as a constant and initialized with 7.50 (7.5% sales tax) |
- You can refer to the below tables for the information about the providers and discounts
Service Provider Category | No. of users = 1 | No. of users = 2 | No. of Items between 3 and 5. |
AT&T | $30.99 per month | $52.99 | 20.99 per user |
T-MOBILE | $39.99 per month | $70.99 | 29.99 per user |
VERIZON | $43.99 per month | $75.99 | 33.99 per user |
Membership Discount | 7.5% | 6.5% | 8.5% |
First Time User Discount | 10% | 5% | 4% |
Coupon Discount | $5 | $5 | $5 |
Sales Tax | 7.5% | 7.5% | 7.5% |
- Constructor: There will be only one constructor. It has the following signature:
public ProviderCalculator (String ProviderName, int numOfUser, boolean isMemberOfMobileAlliance, Char isFirstTimeUser, String hasCoupon) {..........}
- Methods:
You need to write the following methods, which are described below.
- Write getter and setter methods for all private instance variables.
- Write toString method which should be used to display the values for each attribute. This method should return a String. Please see the sample output for knowing the pattern.
- checkProviderName( ). This method accepts the name of the provider entered by the user and returns a Boolean whether the name is correct or no. Note.Use switch
- calcProviderPrice ( ): This method is private of return type double.It returns the provider price based on the provider's name and number of users. Refer the second table for more details.
Note: Use if-else conditions
- calcMembershipDiscount( ):This method is private of return type double and returns the membership discount based on the number of users if the user has membership. Otherwise, return 0.0. Refer the second table for more details.
- calcFirstTimeUserDiscount( ):This method is private of return type double and returns the first time user discount based on the number of users if the user is a first time user. Otherwise, it returns 0.0. Refer the second table for more details.
- Constraints:A user can use ONLY one of the following as discount:
1. Membership discount
2. Coupon discount
3. First time shopper discount
Therefore write another method called chooseYourDiscount( )which acceptthree parameters, isMemberOfMobileAlliance, isFirstTimeUser, and hasCoupon. This method should return a value of type double indicating the discount that the user has chosen based on the options displayed to the user and its respective answer. Make sure to respect the constraints as stated in f).
- calcCouponDiscount(): This method is private of return type double and returns the coupon discount if user has coupon. Otherwise, return 0.0. Refer the second table for more details.
- totalPrice(): This method is private of return type double and returns the price based on the membership, first time user, and coupon. Refer the second table for more details.
- totalPriceWithSalesTax(): This method is private of return type double and returns total price with tax. Refer the second table for more details.
- printReceipt(): This method is public of return type String and returns the receipt.
- Include Javadoc comments, using the @author, @param, and @return annotations when appropriate.
- Generate documentation for your project by clicking on Run from the NetBeans menu bar and then selecting Generate Javadoc. The documentation will be placed in a javadoc subfolder of the subfolder inside your project folder. To view the documentation, open the index.html file that is created.
Driver Class
Instructions:
- Create a class named MobileDriver. This class has a method main and uses one Scanner object to read input from the console.
- Please review the sample output and print the necessary statements in the same format.
- Review the sample output and read the input values from the console appropriately.
- Prompt the user for displaying items.
Sample validations:
All the validations should be done while reading the input in Driver class
- If the provider category is other than AT&T, T-Mobile, and VERIZON
- Then print below message.
"Not a valid provider's name"
- If the number of users is zero. Then print below message.
"The number of users can't be zero."
- In case of many users: The number of users should be between 3 and 5. Otherwise Print the message.
"The numberof users should be between 3 and 5.
- Only one of the following options will be used
- Membership discount
- Coupon discount
- First time shopper discount
- If any two of the above are used, then print below message.
- "User can only choose one option:a) Membership discount, b) Coupon discount, or c) First time shopper discount.
- At the end print "Please try again" message
- Below input has two mistakes. One is user cannot be a member and first time user at a time. Another one is passenger number can't be zero. Then you should print appropriate messages. Please check the below sample output.
Note : User input is in Red
Sample#1 of input/output:
Enter User Details:
Select the provider's name: AT&T, T-Mobile, Verizon: AT&T
How many number of users? 0
Are you a member of the Mobile Alliance? true
Are you a first time user? Y
Do you have coupon? Yes
*******************************
The output should look somehow as below:
Error#1: The number of users should not be zero.
Error#2: User can only use one discount, (1) Member of the Mobile Alliance, (2) First Time User, or (3) has a coupon at a time.
You are required to choose only one option: (1), (2) or (3)
Sample#2 of input/output:
Enter User Details:
Select the provider's name: AT&T, T-Mobile, Verizon: ATT
How many number of users? 6
Are you a member of the Mobile Alliance? false
Are you a first time user? N
Do you have coupon? Yes
Error#1: The provider's name ATT does not match the providers' list
Error#2: the number of users should be between 3 and 5.
Error#3: User can only use one discount, (1) Member of the Mobile Alliance, (2) First Time User, or (3) has a coupon at a time.
You are required to choose only one option: (1), (2), or (3).
Sample#3 of input/output:
Enter User Details:
Select the provider's name: AT&T, T-Mobile, Verizon: AT&T
How many numbers of users? 1
Are you a member of the Mobile Alliance? true
Are you a first-time user? No
Do you have coupon? N
Output: Refer below sample output 3
Sample#4 of input/output:
Enter User Details:
Select the provider's name: AT&T, T-Mobile, Verizon: T-Mobile
How many numbers of users? 3
Are you a member of the Mobile Alliance? false
Are you a first-time user? Yes
Do you have coupon? N
Output: Refer below sample output 4
Sample#5 of input/output:
Enter User Details:
Select the provider's name: AT&T, T-Mobile, Verizon: Verizon
How many numbers of users? 2
Are you a member of the Mobile Alliance? false
Are you a first time user? No
Do you have coupon? Y
Output: Refer below sample output-5
- Invoke printReceipt()to print the customer invoice.
Hint: All the validations should be done while reading the input in Driver class.
Sample output 3:
Enter User Details: -> Select the provider's name: AT&T, T-Mobile, Verizon: AT&T -> How many number of users: 1 -> Are you a member of the Mobile Alliance: true -> Are you a first time user: No -> Do you have coupon: N ******************************* User Provider Details: Provider: AT&T Number of user: 1 Member of the Mobile Alliance: true first time user: No have coupon: N *******************************
|
Sample Output 4 Enter User Details: -> Select the provider's name: AT&T, T-Mobile, Verizon: T-Mobile -> How many number of users: 3 -> Are you a member of the Mobile Alliance: false -> Are you a first time user: Yes -> Do you have coupon: N ******************************* User Provider Details: Provider: T-Mobile Number of user: 3 Member of the Mobile Alliance: false first time user: Yes have coupon: N *******************************
|
Sample Output 5 Enter User Details: -> Select the provider's name: AT&T, T-Mobile, Verizon: Verizon -> How many number of users: 2 -> Are you a member of the Mobile Alliance: false -> Are you a first time user: No -> Do you have coupon: Y ******************************* User Provider Details: Provider: Verizon Number of user: 2 Member of the Mobile Alliance: false first time user: No have coupon: Y *******************************
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
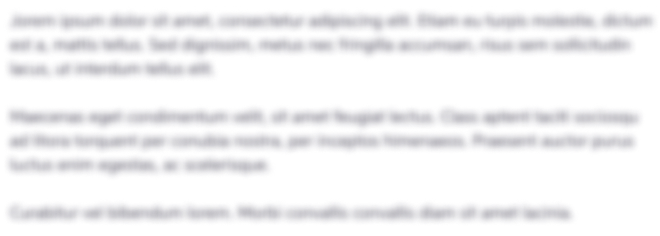
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started