Answered step by step
Verified Expert Solution
Question
1 Approved Answer
check_input.py Set of functions to validate user input. Written by: Shannon Cleary Date: Fall 2022 Functions: get_int(prompt) - returns a valid integer (positive or
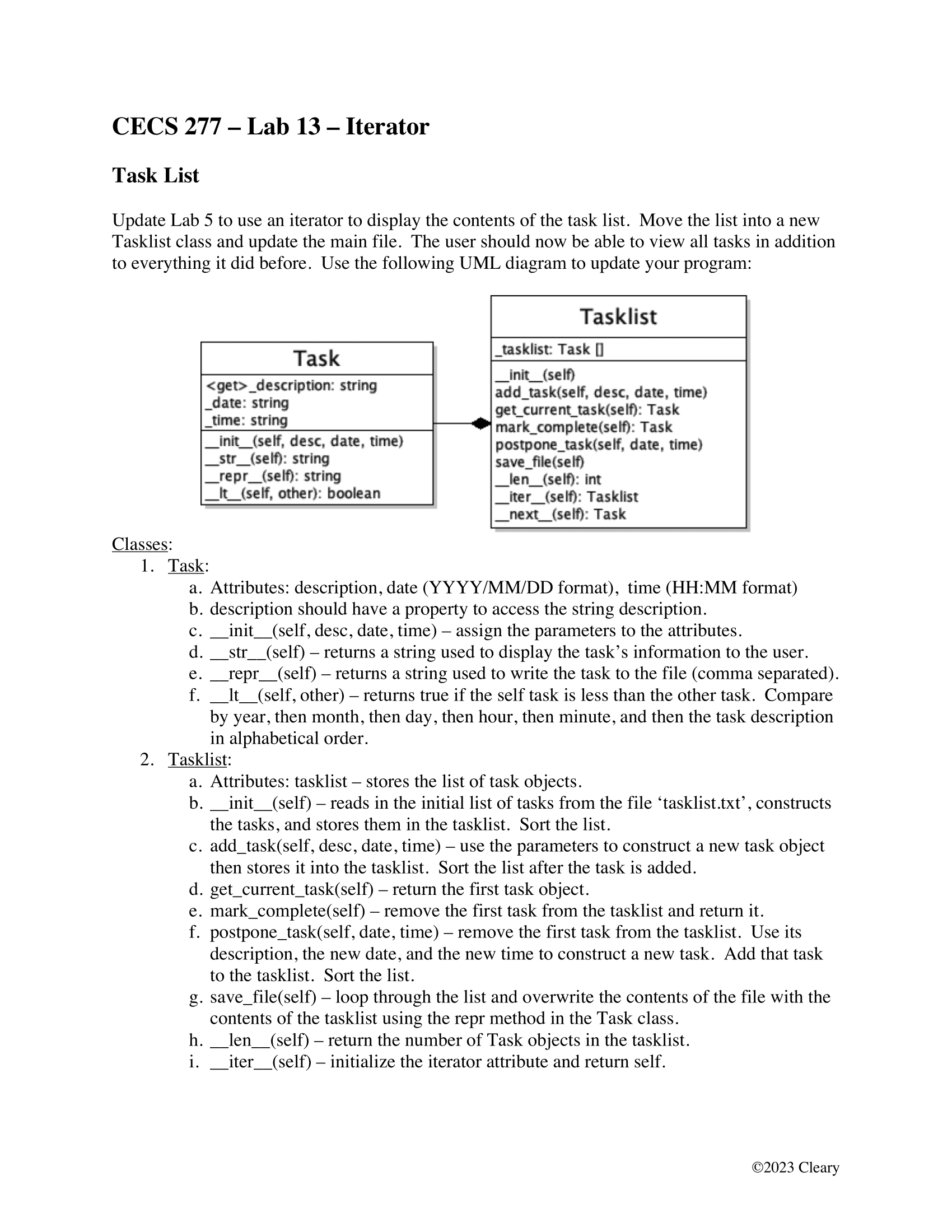
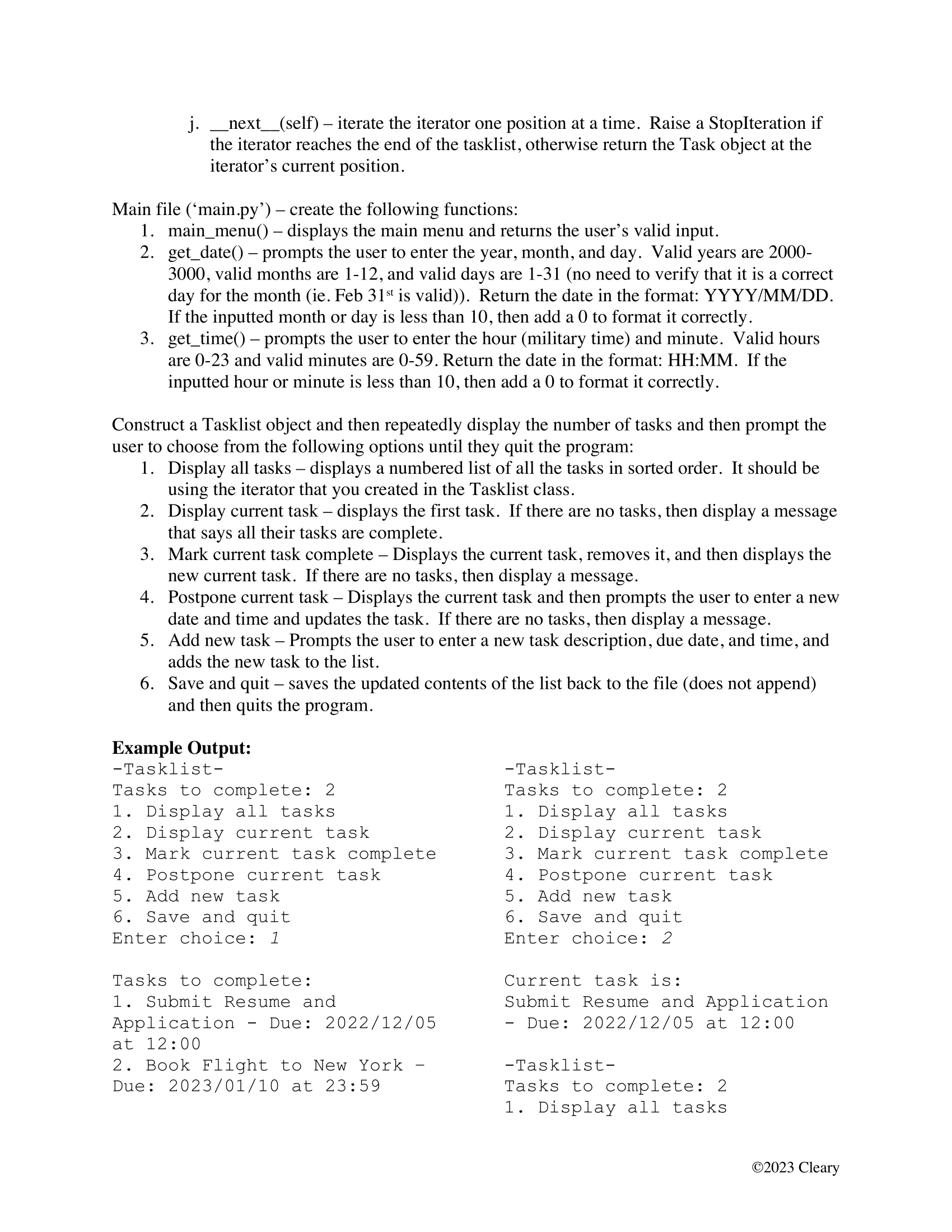
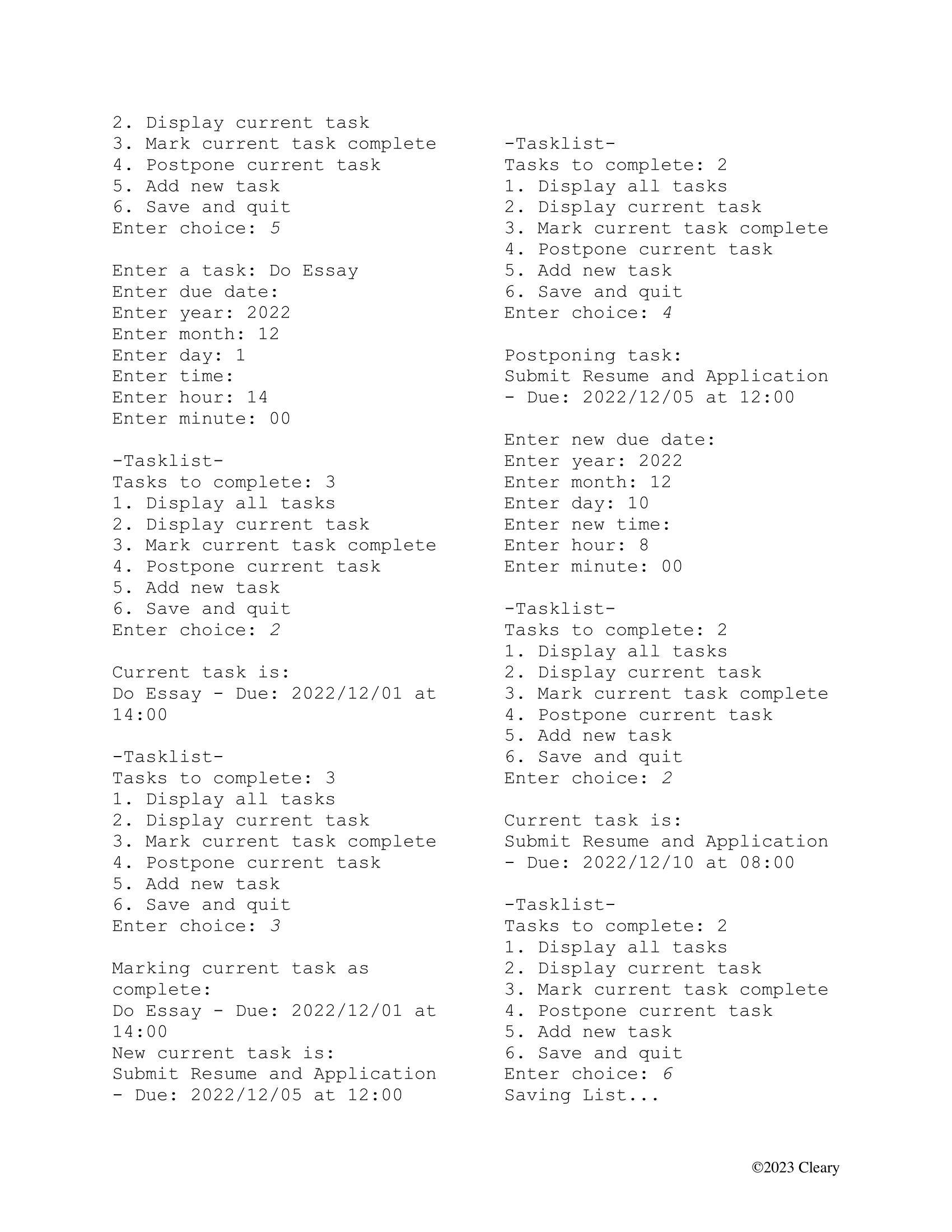
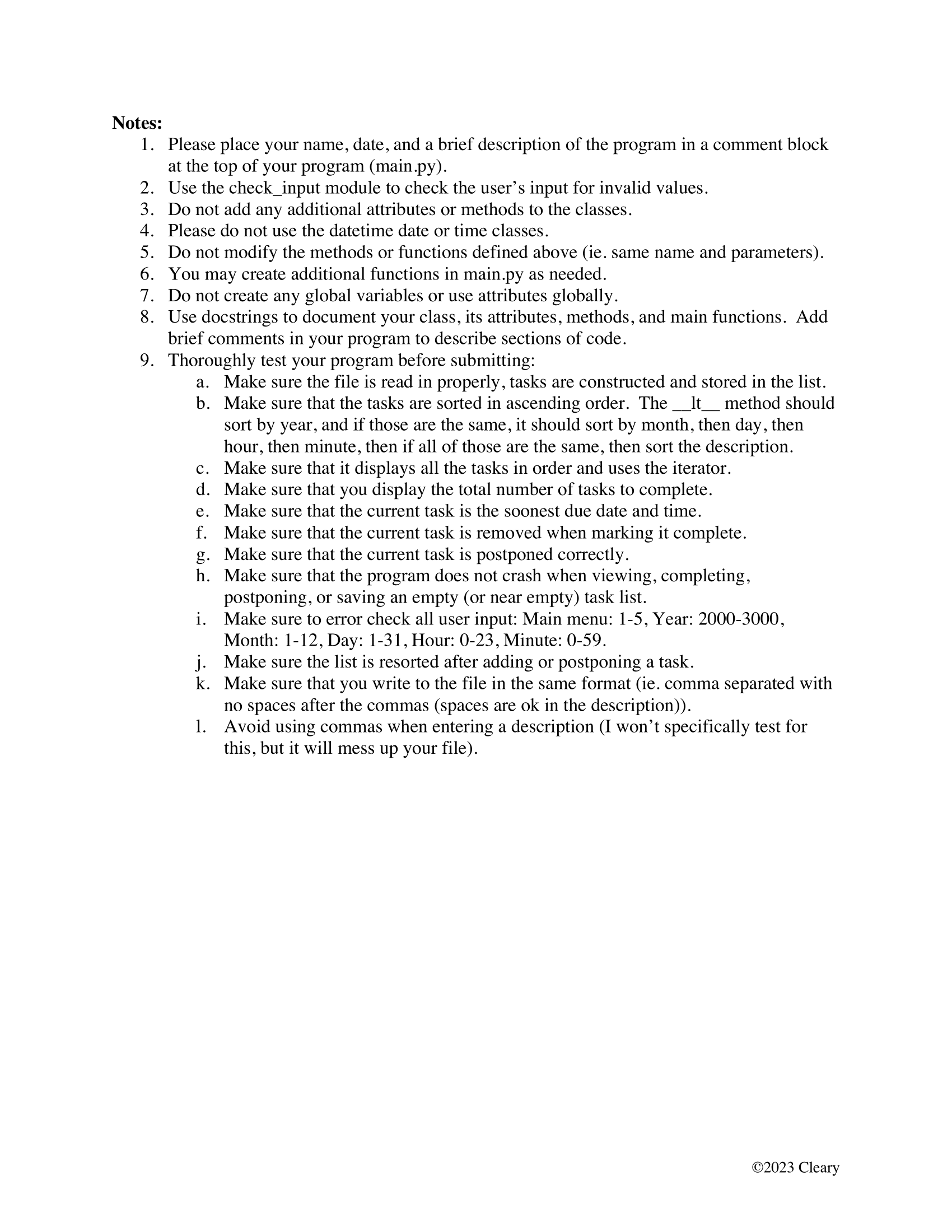
""" Set of functions to validate user input.
Written by: Shannon Cleary
Date: Fall 2022
Functions:
get_int(prompt) - returns a valid integer (positive or negative).
get_positive_int(prompt) - returns a valid positive (>=0) integer.
get_int_range(prompt, low, high) - returns a valid integer within the inclusive range.
get_float(prompt) - returns a valid decimal value.
get_yes_no(prompt) - returns a valid yes/no input.
Usage: in your program, import the check_input module. Then call one of the methods using check_input.
Example Usage:
import check_input
val = check_input.get_int("Enter #:")
print(val)
"""
def get_int(prompt):
"""Repeatedly takes in and validates user's input to ensure that it is an integer.
Args:
prompt: string to display to the user to prompt them to enter an input.
Returns:
The valid positive integer input.
"""
val = 0
valid = False
while not valid:
try:
val = int(input(prompt))
valid = True
except ValueError:
print("Invalid input - should be an integer.")
return val
def get_positive_int(prompt):
"""Repeatedly takes in and validates user's input to ensure that it is a positive (>= 0) integer.
Args:
prompt: string to display to the user to prompt them to enter an input.
Returns:
The valid integer input.
"""
val = 0
valid = False
while not valid:
try:
val = int(input(prompt))
if val >= 0:
valid = True
else:
print("Invalid input - should not be negative.")
except ValueError:
print("Invalid input - should be an integer.")
return val
def get_int_range(prompt, low, high):
"""Repeatedly takes in and validates user's input to ensure that it is an integer within the specified range.
Args:
prompt: string to display to the user to prompt them to enter an input.
low: lower bound of range (inclusive)
high: upper bound of range (inclusive)
Returns:
The valid integer input within the specified range.
"""
val = 0
valid = False
while not valid:
try:
val = int(input(prompt))
if val >= low and val <= high:
valid = True
else:
print("Invalid input - should be within range " + str(low) + "-" + str(high) + ".")
except ValueError:
print("Invalid input - should be an integer.")
return val
def get_float(prompt):
"""Repeatedly takes in and validates user's input to ensure that it is a float.
Args:
prompt: string to display to the user to prompt them to enter an input.
Returns:
The valid floating point input.
"""
val = 0
valid = False
while not valid:
try:
val = float(input(prompt))
valid = True
except ValueError:
print("Invalid input - should be a decimal value.")
return val
def get_yes_no(prompt):
"""Repeatedly takes in and validates user's input to ensure that it is a yes or a no answer.
Args:
prompt: string to display to the user to prompt them to enter an input.
Returns:
True if yes, False if no.
"""
valid = False
while not valid:
val = input(prompt).upper()
if val == "YES" or val == "Y":
return True
elif val == "NO" or val == "N":
return False
else:
print("Invalid input - should be a 'Yes' or 'No'.")
tasklist.txt
Book Flight to New York,2023/01/10,23:59
Haircut,2022/11/20,15:00
Do Math Homework,2022/11/05,12:00
Do Grocery Shopping,2022/11/21,12:00
Do English Assignment,2022/11/15,12:00
Write Email to Supervisor,2022/11/13,12:00
Clean House,2022/11/26,12:00
Read Book for English Class,2022/11/09,23:59
Babysit for Neighbor Kids,2022/11/15,20:00
Submit Resume and Application,2022/12/05,12:00
Proof code running
CECS 277 - Lab 13 Iterator Task List Update Lab 5 to use an iterator to display the contents of the task list. Move the list into a new Tasklist class and update the main file. The user should now be able to view all tasks in addition to everything it did before. Use the following UML diagram to update your program: Classes: e. f. Task _description: string _date: string _time: string _init_(self, desc, date, time) _str_(self): string _repr_(self): string _It_(self, other): boolean 2. Tasklist: Tasklist 1. Task: a. Attributes: description, date (YYYY/MM/DD format), time (HH:MM format) b. description should have a property to access the string description. C. d. _init__(self, desc, date, time) - assign the parameters to the attributes. _str__(self) returns a string used to display the task's information to the user. _repr___(self) returns a string used to write the task to the file (comma separated). _lt__(self, other) returns true if the self task is less than the other task. Compare by year, then month, then day, then hour, then minute, and then the task description in alphabetical order. _tasklist: Task [] __init__(self) add_task(self, desc, date, time) get_current_task(self): Task mark_complete(self): Task postpone_task(self, date, time) save_file(self) _len_(self): int _iter_(self): Tasklist _next_(self): Task a. Attributes: tasklist stores the list of task objects. b. _init__(self) reads in the initial list of tasks from the file 'tasklist.txt', constructs the tasks, and stores them in the tasklist. Sort the list. c. add_task(self, desc, date, time) use the parameters to construct a new task object then stores it into the tasklist. Sort the list after the task is added. d. get_current_task(self) return the first task object. e. mark_complete(self) - remove the first task from the tasklist and return it. f. postpone_task(self, date, time) - remove the first task from the tasklist. Use its description, the new date, and the new time to construct a new task. Add that task to the tasklist. Sort the list. g. save_file(self) - loop through the list and overwrite the contents of the file with the contents of the tasklist using the repr method in the Task class. h. _len___(self) return the number of Task objects in the tasklist. i. _iter___(self) initialize the iterator attribute and return self. 2023 Cleary
Step by Step Solution
There are 3 Steps involved in it
Step: 1
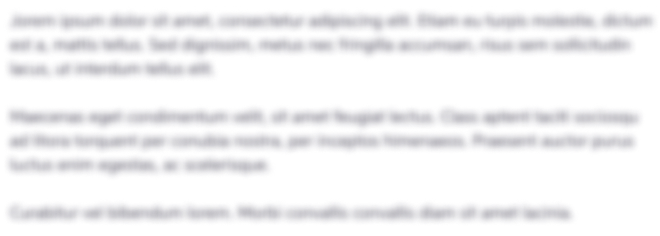
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started