Question
CHEGG ANSWER -> PLEASE READ BOTH ASSIGNMENTS AND MAKE SURE BOTH ARE ANSWERED INDEVISUALY L11a -> PLEASE USE THE FOLLOWING .in FILES BELOW TO ANSWER
CHEGG ANSWER -> PLEASE READ BOTH ASSIGNMENTS AND MAKE SURE BOTH ARE ANSWERED INDEVISUALY
L11a -> PLEASE USE THE FOLLOWING .in FILES BELOW TO ANSWER
Create the Student class derived from the Person class. A student has a studentNumber (an int). Write constructors, the accessor and modifier, a writeOutput method and an equals method. (You can call two Students equal if they have the same ID number). write a compareTo so that students are compared according to their ID number.
Create the Undergraduate class derived from the Student class. An undergraduate has one attribute called Level, an int. all other attributes are derived from Student. Write all constructors, modifier and accessor, a writeOutput. In this instance do NOT add a compareTo. You will see that by doing this you are allowing undergrads to be compared the way that Students are compared. This is because the compiler will look for a compareTo in the undergrad class, and finding none will then go to its super class and find the one in Student.
Submit your L11a project.
Here is a netBeans shell for this lab. L11aForStudents.zip
import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Collections; import java.util.Scanner;
public class InheritanceDemo {
public static void main(String[] args) { File inFile = new File("student.in"); Scanner fileInput = null; try { fileInput = new Scanner(inFile); } catch (FileNotFoundException ex) { //Logger.getLogger(Lab10.class.getName()).log(Level.SEVERE, null, ex); } //input student into an ArrayList ArrayList Student.in 9345 Joseph Smith 9274 Mary Jones 3398 Robert Hatton Jr. 4567 Leon A. White 7465 Sam Donolly undergrad.in 3333 3 John J. Porter Jr. 2846 1 Eliza Doone 3536 2 Steven Sutter 6453 4 Moses Baker 7733 2 Gerald Brooks graduate.in 4456 Masters no Julie Strange 9953 Doctorate yes Amy Burrell Fuller 6756 Masters yes John Doe 7867 Masters no Howard Long III 3322 Doctorate no Jimmy Peters L11a Rubric Correct attributes default constructor constructor with parameters Accessor Mutator WriteOutput This criterion is linked to a Learning Outcomecorrectly derive classes L11 - PLEASE USE THE FOLLOWING CODE TO ANSWER By now you have completed the following classes: Person and Employee. Create a Faculty class, and a Staff class. Both of these are derived from Employee. (This means that Employee is the super class of both Faculty and Staff). The Faculty class should have an attribute to store the Faculty's title (a String) for example "Instructor", "Assistant Professor". The Staff class should have an attribute called pay grade (an integer from 1 to 20) Write a main to fully test your classes. Since they are derived from Employee, and Employee is derived from Person, you will need to have all these classes in your project. It isn't easy to copy and paste whole files in netBeans, I have found the easiest way to get Person and Employee into this project is to create the class again so you have the correct package, and then copy the code from a previous project. Person.java Employee.java Upload ONLY the Faculty class and the Staff class to canvas. L11 Rubric Correct attributes default constructor constructor with parameters Accessor Mutator WriteOutput Correctly derive classes from Employeeclass Person { private String name; Person() { name = null; } Person(String name) { this.name = name; } public void setName(String name) { this.name = name; } public String getName() { return name; } public boolean hasSameName(Person person) { return this.name.equals(person.name); } public void writeOutput() { System.out.println( "Name: " + name); } }
class Employee extends Person { private double salary; private int hireDate; private int id; private String dept; Employee() { this.salary = 0.0; this.hireDate = 0; this.id = 0; this.dept = null; } Employee(double salary, int hireDate, int id, String dept) { this.salary = salary; this.dept = dept; this.id = id; this.hireDate = hireDate; } public void setSalary(double salary) { this.salary = salary; } public double getSalary() { return salary; } public void setHireDate(int hireDate) { this.hireDate = hireDate; } public int getHireDate() { return hireDate; } public void setID(int id) { this.id = id; } public int getID() { return id; } public void setDept(String dept) { this.dept = dept; } public String getDept() { return dept; } public boolean isSameEmployee(Employee emp) { return this.id == emp.id; } public void writeOutput() { System.out.println("Employee ID: " + id); super.writeOutput(); System.out.println("Salary: " + salary + " Hire Date: " + hireDate + " Department: " + dept); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
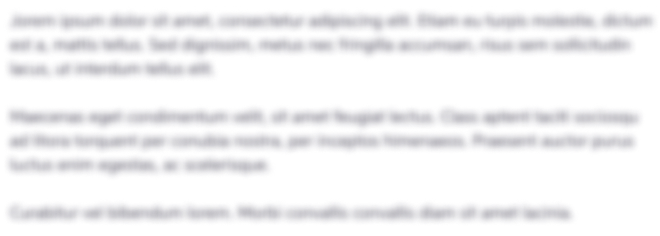
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started