Question
Circle class public class Circle { private static int instanceCnt = 0; private double radius; public static int getInstanceCnt() { return Circle.instanceCnt; } private static
Circle class
public class Circle { private static int instanceCnt = 0; private double radius;
public static int getInstanceCnt() { return Circle.instanceCnt; }
private static void setInstanceCnt(int insCnt) { Circle.instanceCnt = insCnt; }
public Circle() { }
public Circle(double newRadius) { Circle.setInstanceCnt(Circle.getInstanceCnt() + 1); this.setRadius(newRadius); }
public Circle(Circle guest) { }
public double getRadius() { return this.radius; }
private void setRadius(double radius) { if (radius >= 0) this.radius = radius; else this.radius = 0; }
public void resize(double newRadius) { this.setRadius(newRadius); }
public Circle clone() { return new Circle(this.getRadius()); }
public boolean equals(Circle guest) { return guest.getRadius() == this.getRadius(); } public void print() { System.out.print("The circle\'s radius is " + this.getRadius()); } }
Main topics:
Writing Classes Declaring / Using Instance Variables
Writing Instance Methods
Accessors and Mutators
public vs private
Constructors
Member method overloading
static vs non-static
final vs non-final
Exercise
This week you will get more practicing writing a complete class, starting from where you left off last week, which includes class and instance constants. Again careful attention to the public, private and static specifiers will be made.
Getting Started
To start this exercise, you should:
1. Open eclipse and start a new Java project named Lab04
2. Add a Class (named Circle) to this project, and copy the contents of the Circle file provided into it.
3. Add a Class (named CircleDriver) to this project, the contents of which you will be writing from scratch.
Requirements
Circle.java
A simple class which models a circle by its one defining characteristic, which is its radius. This class is moderately complete and must be modified as such:
1. Both the default and copy constructors simply call the specifying constructor, with the appropriate argument
2. Include a class constant for PI Whose value is the best approximation of PI you can store ( public or private? static or not? final or not? )
3. Include an instance constant for units Whose default value is cm ( public or private? static or not? final or not? ) 4. The print() method is modified to include the units the radius is given in
5. Include an overloaded 0-arity mutator for the radius value that sets the radius value to 0 ( where is the one and only place it make sense to call this? )
6. Include an area method that returns the area of the circle in units squared
CircleDriver.java
A simple driver class to test your additions to the Circle class. This class you are writing from scratch, but must include sufficient code to test all of the new features listed above.
________________________________________________________________
This is what I have done so far for the Circle class and I have also added comments
public class Circle
{
private static int instanceCnt = 0;
private static final double pi = 3.14;
private double radius;
private final String unit;
public static int getInstanceCnt()
{
return Circle.instanceCnt;
}
private static void setInstanceCnt(int insCnt)
{
Circle.instanceCnt = insCnt;
}
//default
public Circle()
{
this(0.0,"cm");
}
//specifying
public Circle(double newRadius, String units)
{
Circle.setInstanceCnt(Circle.getInstanceCnt() + 1);
this.setRadius(newRadius);
this.unit = units;
}
//copy
public Circle(Circle guest)
{
this(guest.getRadius(),guest.unit);
}
public double getRadius()
{
return this.radius;
}
public double getArea()
{
return (pi*radius*radius);
}
public String getUnit()
{
return this.unit;
}
/* public void unit (String unit)
* {
* this.unit.equals("cm");
* } */
private void setRadius(double radius)
{
if (radius >= 0)
this.radius = radius;
else
this.radius = 0;//create new method that sets radius to zero and call it here
}
public void resize(double newRadius)
{
this.setRadius(newRadius);
}
public Circle clone()
{
return new Circle(this.getRadius(),this.getUnit());
}
public boolean equals(Circle guest)
{
return guest.getRadius() == this.getRadius();//compare units also
}
public void print()
{
System.out.print("The circle\'s radius is " +
this.getRadius());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
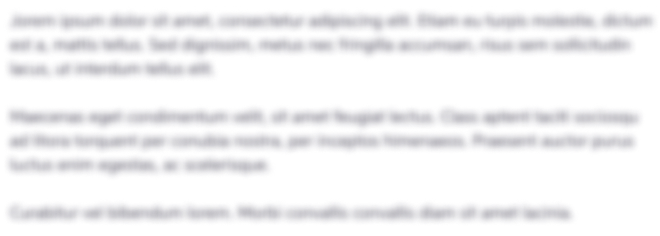
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started