Question
Circular Doubly Linked List Open Eclipse, create a new Java project, and add a new class called CircularDoublyLinkedList . This class will be based heavily
Circular Doubly Linked List
Open Eclipse, create a new Java project, and add a new class called CircularDoublyLinkedList.
This class will be based heavily on the doubly linked list written below. You can use the same node inner class, without modification, as well as the private helper methods. However, this list will NOT have a head or a tail.
Code:
public class DLList
} private static class Node
}
In a circularly linked list, the first and last element are linked together, so that the list becomes a loop. Because of this, we only need a reference to a single node, called the cursor. Thus, CircularDoublyLinkedList will only have two member variables:
cursor -- a reference to one of the nodes in the list, or null if the list is empty
size -- an integer storing the number of nodes in the list
Implement the following methods:
A constructor that creates an empty list
addAfterCursor(T value) -- adds a new node for the given value after the cursor. Be sure you handle the empty list case correctly. The cursor should not change unless the list is empty.
deleteCursor() -- remove the node pointed to by the cursor and return its value. If the list is empty, you should throw an appropriate exception. The cursor should be updated to point to the node after cursor, or to null if the list is empty after deletion.
advanceCursor(int n) -- move the cursor n elements forward. Since the list is circular, this operation will always work. Do nothing if the list is empty.
getValue() -- return the value stored at the cursor. If the list is empty, throw an appropriate exception.
Add a Driver class with a main method, and test your list by performing a "lottery" simulation:
Add the numbers 1-10 to a CircularDoublyLinkedList
While the list is not empty:
Advance the cursor a random amount
Print the element at the cursor
Delete the cursor.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
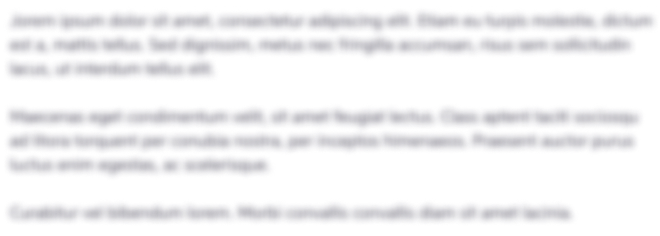
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started