Question
Circular queue array, please comment beside code the Queue array implementation could be improved to eliminate shuffling if we treated the array as if it
Circular queue array, please comment beside code
the Queue array implementation could be improved to eliminate shuffling if we treated the array as if it were circular, so that when you try to add an item past the end, you insert it at the start instead.
Produce a modified version of QueueArray.java called something such as QueueCircularArray.java. This will involve keeping track of the current head and tail and allowing them to increase continuously as items are enqueued/dequeued. These head and tail positions are virtual array indices, from which actual indices are computed using modular arithmetic. You also will have to change how isEmpty and isFull operate. When testing, make sure to enqueue/dequeue enough nodes so that it wraps around. Consider using loops in your testing.
import javax.swing.JOptionPane; public class ArrayQueue implements Queue { protected Object Q[]; // array used to implement the queue protected int rear = -1; // index for the rear of the queue protected int capacity; // The actual capacity of the queue array public static final int CAPACITY = 1000; // default array capacity public ArrayQueue() { // default constructor: creates queue with default capacity this(CAPACITY); } public ArrayQueue(int cap) { // this constructor allows you to specify capacity capacity = (cap > 0) ? cap : CAPACITY; Q = new Object[capacity]; } public void enqueue(Object n) { if (isFull()) { JOptionPane.showMessageDialog(null, "Cannot enqueue object; queue is full."); return; } rear++; Q[rear] = n; } public Object dequeue() { // Can't do anything if it's empty if (isEmpty()) return null; Object toReturn = Q[0]; // shuffle all other objects towards 0 int i = 1; while (i <= rear) { Q[i-1] = Q[i]; i++; } rear--; return toReturn; } public boolean isEmpty() { return (rear < 0); } public boolean isFull() { return (rear == capacity-1); } public Object front() { if (isEmpty()) return null; return Q[0]; } }
public interface Queue { // most important methods public void enqueue(Object n); // add an object at the rear of the queue public Object dequeue(); // remove an object from the front of the queue // others public boolean isEmpty(); // true if queue is empty public boolean isFull(); // true if queue is full (if it has limited storage) public Object front(); // examine front object on queue without removing it }
import javax.swing.JOptionPane; public class QueueTest { public static void main(String[] args) { // Create a Queue Queue q = new ArrayQueue(); // Put some strings onto the queue JOptionPane.showMessageDialog(null, "About to enqueue words onto queue: The end is nigh!"); q.enqueue("The"); q.enqueue("end"); q.enqueue("is"); q.enqueue("nigh!"); // Now dequeue them from the queue JOptionPane.showMessageDialog(null, "About to dequeue the words ..."); while(! q.isEmpty()) { String word = (String)q.dequeue(); // Note: have to cast Objects popped to String JOptionPane.showMessageDialog(null, "Word dequeued: " + word); } System.exit(0); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
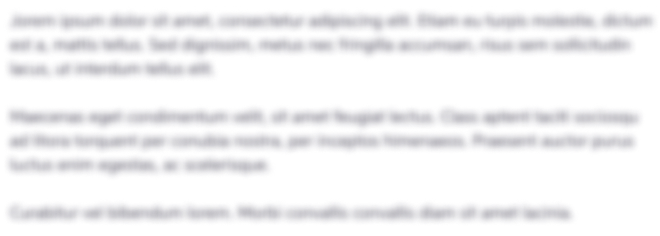
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started