Question
CIS Project which involves databases. I need to create classes that allow me to sort through a database of every country. I should be able
CIS Project which involves databases. I need to create classes that allow me to sort through a database of every country. I should be able to figure out the name of the country, the capital, population, area, gdp, etc. I'm including the outline for methods that need to be in each class. Looking for answers to steps 3-5
Step 1: Create a new project called "Project 4"
Step 2: Download data file
Step 3: Create a class called country and include the following:
Instance variable:
Name of the country (String)
Continent in which the country is located (String)
Area of the country given in square kilometers (int)
Population of the country (double)
Gross domestic product (gdp) is a monetary measure of the market value of all final goods and services produced in a period. (double)
Capital of the country (String)
Constructors:
public Country () default constructor set the name to "Default "
public Country (String name, String continent, int area, double population, double gdp, String capital) - a constructor that initializes all the instance variables to appropriate values passed as input parameters
Accessors and Mutators:
provide get methods for each one of the instance variables
provide matching set methods for each instance variable. It is good practice to provide set methods for each instance variable.
public String toString() - return a formatted String of the country.
For example: the return value for the country United States will be:
United States, Capital: Washington D.C., GDP: 16,200 billion, Per Capita GDP: 50,892
Notes: the gdp is formatted in billions. 1 billion is: 1000000000 (nine zeroes).
Use DecimalFormat to format the numeric values.
Per capita gdp is the gdp divided by the population: gdp / population
public static void main(String [] args) thoroughly test each of the methods in this class.
Step 4: Create a class called countryDatabase
Instance variables / class fields: Define an instance variable that holds an ArrayList of elements of the Country class.
Constructor
A constructor is a special method with the same name as the class and generally initializes the fields to appropriate starting values.
public CountryDatabase () - instantiate the ArrayList that will contain the countries of the world. This method will be one line of code.
Mutator Methods
Mutator methods perform tasks that may modify class fields. Methods that set a field with the parameter value often begin with the prefix set.
public void readCountriesData(String filename) - open the provided filename and read all the data. We are providing most of the code to read the file. See Background Information: Reading from a Text File on page 9.
Accessor Methods
The names for these methods, which simply return the current value of a field, often begin with the prefix get.
Note: Use the elegant for-each loop every time you need to search the ArrayList
public int countAllCountries () - return the number of items in the ArrayList using one line of code.
public ArrayList
public Country highestGdp(String continent) search the full list and return the country that has the highest gdp in the specified continent.
public Country smallestArea(String continent) search the full list and return the country that has the smallest area in the specified continent entered as parameter.
public String searchForCapital(String countryName) - search the full list and return the capital of the country entered as parameter. The search should not be case sensitive - hint: use equalsIgnoreCase to compare the names-. If the countryName entered as parameter is not found, return null.
public Country findCountryDetails(String countryName) - search the full list and return the country that matches the name entered as parameter. The search should not be case sensitive - hint: use equalsIgnoreCase to compare the names-. If the countryName entered as parameter is not found, return null.
public ArrayList
public ArrayList
Step 5: Generate a Top Ten List (10 pts)
To generate a top ten list of countries based on the gdp, your solution must be able to sort the countries in descending order by gdp. This requires small changes to the Country class and a new method in the CountryDatabase class.
Changes to the Country Class
Add two words to the end of the class header (shown below). This allows Country objects to be compared using compareTo() and therefore sorted.
public class Country implements Comparable {
Also, add the following method. This method allows two Country objects to be compared with respect to the gdp.
IMPORTANT NOTE: For the compareTo code below, we are assuming that the name of the instance variable for the gross domestic product is gdp
public int compareTo(Object other){
Country c = (Country) other;
return (int)(c.gdp gdp);
}
Changes to CountryDatabase class
NOTE: Sorting an ArrayList, called tempList, can be performed with one line of code: Collections.sort(tempList);
Now that you know how to sort search results, sort the temporary list in the searchCountriesInContinent (String continent) method before returning. You only need to add one line of code to this method.
public ArrayList
Background Information: Reading from a Text File
The data file contains information of 195 countries. A sample line is shown below. The readCountriesData() method reads six items and passes them to the Country class constructor.
"United States","North America",9826675,318320000,16244600000000,"Washington D.C."
The following method reads from a text file one line at a time. The solution is a bit more complex than an example in Zybooks section 15.5.1. Look for FIX ME comments for suggestions of improvements.
Sample Code
public void readCountriesData(String filename){
// Read the full set of data from a text file
try{
// open the text file and use a Scanner to read the text
FileInputStream fileByteStream = new FileInputStream(filename);
Scanner scnr = new Scanner(fileByteStream);
scnr.useDelimiter("[, ]+");
// keep reading as long as there is more data
while(scnr.hasNext()) {
// reading the fields from the record one at the time
String name = scnr.next();
String continent = scnr.next();
// FIX ME: capture the area, population, GDP, and capital
// instantiate a new Country with the data that was read
Country entry = new Country(name, continent, area, population,
gdp, capital)
// FIX ME: Add the Country created on previous instruction
// to the ArrayList
}
fileByteStream.close();
}
catch(IOException e) {
System.out.println("Failed to read the data file: " + filename);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
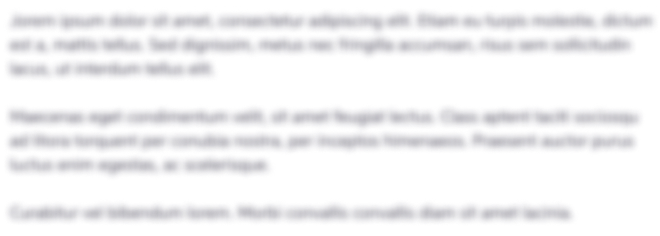
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started