Question
Clas Dimensions: The constructor needs to provide input validation. Only positive values are allowed for height, width, and depth. If one or more of the
- Clas Dimensions:
- The constructor needs to provide input validation. Only positive values are allowed for height, width, and depth. If one or more of the parameter values are zero or negative, an IllegalArgumentException should be thrown. We'll introduce exception handling in one of the upcoming modules. For now, just use the code statement provided. throw new IllegalArgumentException("The height, width, and depth of a dimension needs to be positive.");
- The method toString should return a string of the following format: ({height} x {width} x {depth}) Where {height}, {width}, and {depth} are substituted by the corresponding field values rounded to one digit after the decimal point. E.g., the dimensions 137.81 x 64 x 9.2 return: (137.8 x 64.0 x 9.2)
- Clas Phone
- The method toString should return a string of the following format: {model} {dimension} Where {model} and {dimention} are substituted by the corresponding field values E.g.: Samsung Galaxy S9 (147.7 x 68.7 x 8.5)
- Clas SmartPhone
- Analogous to class Dimension, the constructor needs to provide input validation. Only positive values are allowed for the field storage. If there is an attempt to create a new smartphone with an invalid storage value, an IllegalArgumentException should be thrown. This time the error message should be "Storage needs to be assigned a positive value."
- The method call should return the following string: Calling {number} by selecting the number Where {number} is substituted by the corresponding parameter value. E.g.: Calling 8015817200 by selecting the number
- The method browse should return the following string: Browsing the web
- The method takePicture should return one of the following two strings: Taking a horizontal picture or Taking a vertical picture Each time the method is called, one of those two strings should be chosen randomly.
- The method toString should return a string of the following format: {model} {dimension} {storage}GB Where {model}, {dimension} and {storage} are substituted by the corresponding field values and GB stands for gigabyte. E.g.: Samsung Galaxy S9 (147.7 x 68.7 x 8.5) 64GB
- Enum Voltage Voltage provides three options: V110, V220, and DUAL Those three options correlate to 110 Volt which is used in the US, 220 Volt like in Europe, and the ability to work with both 110 and 220 Volt
- Clas DeskPhone
- The constructor includes two parameters to initialize the superclass Phone and a third parameter to initialize the field voltage. However, there is no parameter to initialize the field connected. That's because a new DeskPhone out of the box is never connected.
- The method plugIn sets the field connected to true
- The method unplug sets the field connected to false
- The method isConnected returns the value of the corresponding field
- The method toString should return a string of the following format: {model} {dimension} {voltage} {connectionInfo} Where {model}, {dimension} and {voltage} are substituted by the corresponding field values and {connectionInfo} is one of the following two strings: connected if the desk phone is connected and not connected if it is not connected. E.g.: Crosley CR62-BK (127.0 x 153.0 x 127.0) DUAL connected E.g.: Opis PushMeFon (228.0 x 217.0 x 130.0) V220 not connected
- Clas RotaryPhone
- The method call should return the following string: Rotating the dial to call {number} Where {number} is substituted by the corresponding parameter value. E.g.: Rotating the dial to call 8015817200
- Clas PushButtonPhone
- The method call should return the following string: Pushing buttons to call {number} Where {number} is substituted by the corresponding parameter value. E.g.: Pushing buttons to call 8015817200
Testing code:
package phones;
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test;
/** * = = = This test class should not be modified. = = = */ class DeskPhoneTest { private DeskPhone pushButtonPhone; private DeskPhone rotaryPhone; @BeforeEach void setUp() throws Exception { pushButtonPhone = new PushButtonPhone( "JeKaVis J-P45", new Dimension(85, 85, 28), Voltage.DUAL); rotaryPhone = new RotaryPhone( "Pyle PRT45", new Dimension(205, 135, 92), Voltage.V110); } @Test void deskPhone_initializeTheFields() { DeskPhone p = new RotaryPhone( "Model 500", new Dimension(143, 208, 119), Voltage.V110); assertFalse(p.isConnected()); assertEquals("Model 500 (143.0 x 208.0 x 119.0) V110 not connected", p.toString()); }
@Test void plugIn_connectPhone() { pushButtonPhone.plugIn(); assertTrue(pushButtonPhone.isConnected()); rotaryPhone.plugIn(); assertTrue(rotaryPhone.isConnected()); }
@Test void unplug_disconnectPhone() { pushButtonPhone.unplug(); assertFalse(pushButtonPhone.isConnected()); rotaryPhone.unplug(); assertFalse(rotaryPhone.isConnected()); }
@Test void isConnected_pluggedIn_returnTrue() { pushButtonPhone.plugIn(); assertTrue(pushButtonPhone.isConnected()); }
@Test void isConnected_unplugged_returnFalse() { rotaryPhone.unplug(); assertFalse(rotaryPhone.isConnected()); } @Test void toString_returnsModelDimensionVoltageAndConnectionInfo() { assertEquals("JeKaVis J-P45 (85.0 x 85.0 x 28.0) DUAL not connected", pushButtonPhone.toString()); rotaryPhone.plugIn(); assertEquals("Pyle PRT45 (205.0 x 135.0 x 92.0) V110 connected", rotaryPhone.toString()); }
} I can upload the other testing ones in the comments, not enough space to do it here
Add one more class to the same package. It is called TestClient and it includes the main method.
- Create four variables that store the following objects:
- A RotaryPhone, model Model 500 with dimensions 143 x 208 x 119 and 110 Volt
- A PushButtonPhone, model Cortelco 2500 with dimensions 178 x 228 x 127 and the ability to connect both to 110 Volt and 220 Volt
- A SmartPhone, model Pixel3 with dimensions 145.6 x 68.2 x 7.9 and 128GB storage
- A SmartPhone, model iPhone8 with dimensions 138.4 x 67.3 x 7.3 and 64GB storage
- Print the title Various Phones: Call the toString methods of all four objects and print the results in separate lines. After that, print a single empty line to group related output.
- Plug in both desk phones. Then randomly select one of the desk phones and unplug it. This selection needs to be done dynamically (at runtime).
- Create an array of type Phone. Add the different phones we created above as elements to the array.
- Print the title Array Elements:
- Loop through all the elements in the array. For each of the array elements do the following:
- Call the method toString and print it.
- Make a call to the following number: 8019574111 and print the result
- If it is a SmartPhone, browse and take a picture. Print the results.
Various Phones: Model 500 (143.0 x 208.0 x 119.0) V110 not connected Cortelco 2500 (178.0 x 228.0 x 127.0) DUAL not connected Pixel3 (145.6 x 68.2 x 7.9) 128GB iPhone8 (138.4 x 67.3 x 7.3) 64GB Array Elements: Model 500 (143.0 x 208.0 x 119.0) V110 connected Rotating the dial to call 8019574111 Cortelco 2500 (178.0 x 228.0 x 127.0) DUAL not connected Pushing buttons to call 8019574111 Pixel3 (145.6 x 68.2 x 7.9) 128GB Calling 8019574111 by selecting the number Browsing the web Taking a vertical picture iPhone8 (138.4 x 67.3 x 7.3) 64GB Calling 8019574111 by selecting the number Browsing the web Taking a horizontal picture
UJJD1U5 dl TIULICS Phone - model : String #Phone(model : String, dimension : Dimension) + calumber: longString + toString(): String Dimension - height: double -width: double -depth : double + Dimension(height: double, width: double, depth : double) + getHeight(): double + getwidth ) : double + getDepth(): double + toString(): Sring SmartPhone - Storage: int + SmartPhone model : String, dimension : Dimension, storage: int) + call number: longi: String + browse): Sering + takePicture(): String + toString(): String Des Phone Enum Voltage V110 V220 DUAL -voltage + connected: boolean # DeskPhone model: String, dimension : Dimension, voltage: Voltage) + plugin + unplug) + is connected ) : boolean +toString): String RotaryPhone Push ButtonPhone + RotaryPhone model: String, dimension : Dimension, voltage : Voltage) + call number: longo String + Push ButtonPhone(model: String, dimension: Dimension, voltage : Votage) + callinumber: longi-Sarim Gm UJJD1U5 dl TIULICS Phone - model : String #Phone(model : String, dimension : Dimension) + calumber: longString + toString(): String Dimension - height: double -width: double -depth : double + Dimension(height: double, width: double, depth : double) + getHeight(): double + getwidth ) : double + getDepth(): double + toString(): Sring SmartPhone - Storage: int + SmartPhone model : String, dimension : Dimension, storage: int) + call number: longi: String + browse): Sering + takePicture(): String + toString(): String Des Phone Enum Voltage V110 V220 DUAL -voltage + connected: boolean # DeskPhone model: String, dimension : Dimension, voltage: Voltage) + plugin + unplug) + is connected ) : boolean +toString): String RotaryPhone Push ButtonPhone + RotaryPhone model: String, dimension : Dimension, voltage : Voltage) + call number: longo String + Push ButtonPhone(model: String, dimension: Dimension, voltage : Votage) + callinumber: longi-Sarim GmStep by Step Solution
There are 3 Steps involved in it
Step: 1
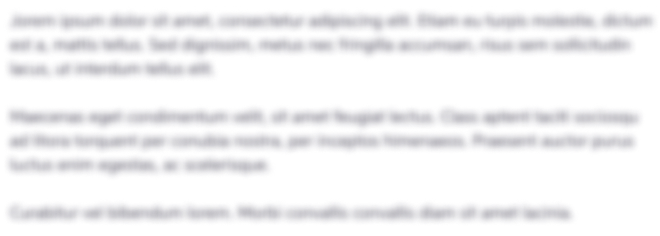
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started