Question
// class BookGenre package library.service.classes; public enum BookGenre {GENRE_HISTORY,GENRE_SCIENCE,GENRE_ENGINEERING,GENRE_LITERATURE}; // class BookRecord package library.service.classes;//set up the package import library.service.classes.BookGenre; public class BookRecord{ int recordNo; String
// class BookGenre
package library.service.classes; public enum BookGenre {GENRE_HISTORY,GENRE_SCIENCE,GENRE_ENGINEERING,GENRE_LITERATURE};
// class BookRecord
package library.service.classes;//set up the package
import library.service.classes.BookGenre;
public class BookRecord{
int recordNo;
String title;
String []authors;
BookGenre genre;
static int cnt=10000;
/on-default constructor
public BookRecord(String title, String genre, String [] name){
this.setTitle(title);
this.setGenre(genre);
this.setAuthors(name);
this.setRecordNo();
}
//accessors
public String getTitle(){
return this.title;
}
public BookGenre getGenre(){
return this.genre;
}
public String [] getAuthors(){
String []ret = new String[this.authors.length];
for(int i=0;i ret[i]=this.authors[i]; } return ret; } public String getAuthorList(){ String ret=""; for(int i=0;i ret=ret + " " +this.authors[i]; } return ret; } public int getRecordNo(){ return this.recordNo; } //mutators public void setRecordNo(){ this.recordNo=BookRecord.cnt++; } public void setTitle(String title){ this.title=title; } public void setGenre(String genre){ this.genre=BookGenre.valueOf(genre); } public void setAuthors(String []authorList){ this.authors = new String[authorList.length]; for(int i=0;i this.authors[i]=authorList[i]; } } //toString method public String toString(){ String str=""; str = str + "=================================== "; str = str + "Record No:" + this.getRecordNo() + " "; str = str + "Title:" + this.getTitle() + " "; str = str + "Genre: " + this.getGenre() + " "; str = str + "Authors: " + this.getAuthorList() + " "; str = str + "=================================== "; return str; } //toEquals method public boolean equals(BookRecord aRecord){//remember you should not compare the record no if(!this.getTitle().equals(aRecord.getTitle())) return false; if(!this.equalsHelper(aRecord)) return false; return true; } private boolean equalsHelper(BookRecord aRecord){ for(int i=0;i if(!this.authors[i].equals(aRecord.authors[i])){ //assume the authors will always be provided in the same order return false; } } return true; } } // class library package library.client.classes; import java.util.Scanner; import java.io.File; import java.io.IOException; import library.service.classes.BookGenre; import library.service.classes.BookRecord; import java.util.Vector; class library{ /*Task 1: Declare the vector of objects*/ library(){ /* Task 2: Initiliaze vector*/ /* Task 3: Print out its capacity and its size*/ } public void searchByGenre(BookGenre genre){//search the vector for records of specific genre /* Task 5: implement searchByGenre() to search for records of a particylar genre in the vector*/ } public void print(){//list the records /* Task 6: Implement the print() to list out the records*/ } public static void main(String []args){//instantiate the library //arg[0]: text file library myLib = new library(); //read the the files from text files String []authors; BookRecord aRecord; Scanner scan; String str; try { File myFile=new File(args[0]); scan=new Scanner(myFile);//each line has the format title:genre:author-name-1,author-name-2..authorname-m while(scan.hasNextLine()){ str=scan.nextLine(); String []tok=str.split(":"); authors=tok[2].split(","); aRecord = new BookRecord(tok[0],tok[1],authors); /*Task 4: Add the objects to the vector*/ } scan.close(); }catch(IOException ioe){ System.out.println("The file can not be read"); } //User interactive part String option1, option2; scan = new Scanner(System.in); option1=""; while(!option1.equals("Q")){ System.out.println("Select an option:"); System.out.println("Type \"S\" to list books of a genre"); System.out.println("Type \"P\" to print out all the book recors"); System.out.println("Type \"Q\" to Quit"); option1=scan.nextLine(); switch (option1) { case "S": System.out.println("Type a genre. The genres are:"); for (BookGenre d : BookGenre.values()) { System.out.println(d); } option2=scan.nextLine(); //assume the user will type in a valid genre myLib.searchByGenre(BookGenre.valueOf(option2));//implement this method break; case "P": myLib.print();//print the array; implement this method break; case "Q": System.out.println("Quitting interactive part"); break; default: System.out.println("Wrong option! Try again"); break; } } /*Task 7- Create 2 dimensional array using the records from the vector The array has rows for GENRE_HISTORY, GENRE_SCIENCE, GENRE_ENGINEERING, GENRE_LITERATURE*/ BookRecord [][] libAr; //declaration of the 2D array libAr = new BookRecord[4][]; for (BookGenre d : BookGenre.values()) {//for each genre count the number of records belonging to it and then intantiate its row /*add code here*/ } System.out.println("Printing out the array. Type enter to proceed"); option1=scan.nextLine(); /*Task 8: Print out the array*/ System.out.println("Removing the duplicates. Type enter to proceed"); option1=scan.nextLine(); /* Task 9: Identify and remove the duplicate records IN THE VECTOR (NOT THE ARRAY) and print out the removed records */ System.out.println("Note the record nos. of the duplicated records"); System.out.println("Printing out the array. Type enter to proceed"); option1=scan.nextLine(); /* Task 10: print out the array again; are the duplicated printed out again? */ } } //books.txt Thomas Jefferson and the Tripoli Pirates:GENRE_HISTORY:Brian Kilmeade,Don Yaeger Component-oriented programming:GENRE_ENGINEERING:C. Szyperski,J. Bosch,W. Weck Microfabricated microneedles, a novel approach to transdermal drug delivery:GENRE_ENGINEERING:S. Henry,D. V. McAllister,M. G. Allen Nikola Tesla:GENRE_HISTORY:Sean Patrick English landscaping and literature, 1660-1840:GENRE_LITERATURE:E. Malins A history and theory of informed consent:GENRE_HISTORY:R. R. Faden,T. L. Beauchamp,N. M. King The Feminist Companion to Literature in English Women Writers From the Middle Ages to the Present:GENRE_LITERATURE:V. Blain,P. Clements,I. Grundy Climate and atmospheric history of the past 420,000 years:GENRE_HISTORY:J. R. Petit, J. Jouzel,D. Raynaud,N. I. Barkov,J. M. Barnola The comparative method in evolutionary biology:GENRE_SCIENCE:P. H. Harvey,M. D. Pagel Human-computer interaction:GENRE_ENGINEERING:J. Preece,Y. Rogers,H. Sharp,D. Benyon,S. Holland Free radicals in biology and medicine:GENRE_SCIENCE:B. Halliwell,J. M. C. Gutteridge Electron transfers in chemistry and biology:GENRE_SCIENCE:R. A. Marcus,N. Sutin English landscaping and literature, 1660-1840:GENRE_LITERATURE:E. Malins Device electronics for integrated circuits:GENRE_ENGINEERING:R. S. Muller,T. I. Kamins,M. Chan,P. K. Ko An outline of English literature:GENRE_LITERATURE:G. C. Thornley,G. Roberts Gene Ontology:GENRE_SCIENCE:M. Ashburner,C. A. Ball,J. A. Blake,D. Botstein,H. Butler Nikola Tesla:GENRE_HISTORY:Sean Patrick Microfabricated microneedles, a novel approach to transdermal drug delivery:GENRE_ENGINEERING:S. Henry,D. V. McAllister,M. G. Allen
Step by Step Solution
There are 3 Steps involved in it
Step: 1
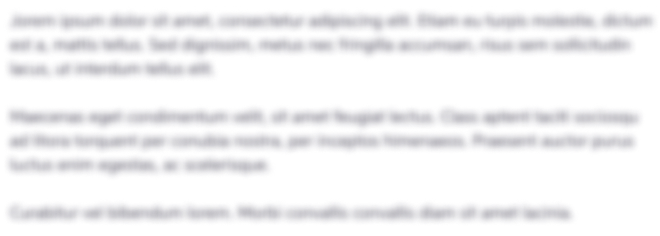
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started