Question
class MyStack { private int maxSize; // size of stack array private long [] stackArray; private int top; // top of stack public MyStack( int
class MyStack
{
private int maxSize; // size of stack array
private long[] stackArray;
private int top; // top of stack
public MyStack(int s) // constructor
{
maxSize = s; // set array size
stackArray = new long[maxSize]; // create array
top = -1; // no items yet
}
public void push(long j) // put item on top of stack
{
stackArray[++top] = j; // increment top, insert item
}
public long pop() // take item from top of stack
{
return stackArray[top--]; // access item, decrement top
}
public long peek() // peek at top of stack
{
return stackArray[top];
}
public boolean isEmpty() // true if stack is empty
{
return (top == -1);
}
public boolean isFull() // true if stack is full
{
return (top == maxSize-1);
}
public long getBottom()
{
if(!isEmpty())
{
return stackArray[0];
}
else {
return -1;
}
}
public MyStack stackStack(MyStack T)
{
MyStack newStack=new MyStack(T.maxSize+maxSize);
while(!isEmpty())
{
newStack.push(pop());
}
while(!newStack.isEmpty())
{
T.push(newStack.pop());
}
return T;
}
}
/////////////////////////////////////////////////////////////////////////////
public static void main(String[] args)
{
MyStack theStack = new MyStack(10);
// make new stack
theStack.push(20); // push items onto stack
theStack.push(40);
theStack.push(60);
theStack.push(80);
MyStack secondStack= new MyStack(10);
secondStack.push(1);
secondStack.push(7);
secondStack.push(3);
MyStack stackStack=new MyStack(10);
stackStack=theStack.stackStack(secondStack);
while(!theStack.isEmpty())
{
long value= theStack.pop();
System.out.print(value);
System.out.print(" ");
}
System.out.println("");
}
}
As you can see from above I wrote a code to solve these two problems, however, it does not work, Can someone tell me exactly what I did wrong and how to fix it? Thanks
2) For our MyStack class we created methods emptyCheck() which returns True if the stack becomes empty; top), that returns the value of the top element in the stack; push(a1) that places the value of al onto the top of the stack; and popi) that removes the top element of the stack, if any. To process any stack, you may only use those routines and some primitive data variables to temporarily store a value or two. If necessary, you can temporarily create a second stack to store values. a) Design a method in MyStack called getBottomValue() that returns the values at the bottom of the stack. Design a method in MyStack called stackStack(MyStack T) that pushes the values from stack T, in the correct order. When the routine is done, the top element in the ("this") stack should be the top element from T. T should also be restored to its original condition. b) Illustration Stack T 6 The ('this") Stack Resulting "this" Stack
Step by Step Solution
There are 3 Steps involved in it
Step: 1
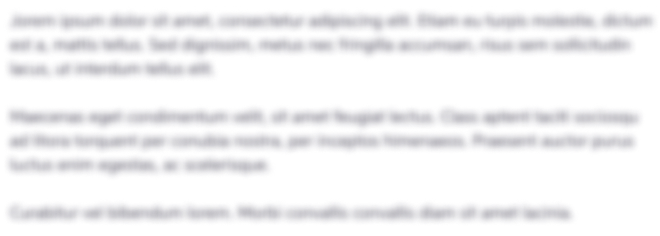
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started