Answered step by step
Verified Expert Solution
Question
1 Approved Answer
class Node : def __init__ ( self , value) : self .value = value self . next = None def __str__ ( self ) :
class Node: def __init__(self, value): self.value = value self.next = None def __str__(self): return "Node({})".format(self.value) __repr__ = __str__ class SortedLinkedList: ''' >>> x=SortedLinkedList() >>> x.add(8.76) >>> x.add(1) >>> x.add(1) >>> x.add(1) >>> x.add(5) >>> x.add(3) >>> x.add(-7.5) >>> x.add(4) >>> x.add(9.78) >>> x.add(4) >>> x Head:Node(-7.5) Tail:Node(9.78) List:-7.5 -> 1 -> 1 -> 1 -> 3 -> 4 -> 4 -> 5 -> 8.76 -> 9.78 >>> x.replicate() Head:Node(-7.5) Tail:Node(9.78) List:-7.5 -> 1 -> 1 -> 1 -> 3 -> 3 -> 3 -> 4 -> 4 -> 4 -> 4 -> 4 -> 4 -> 4 -> 4 -> 5 -> 5 -> 5 -> 5 -> 5 -> 8.76 -> 9.78 >>> x Head:Node(-7.5) Tail:Node(9.78) List:-7.5 -> 1 -> 1 -> 1 -> 3 -> 4 -> 4 -> 5 -> 8.76 -> 9.78 >>> x.removeDuplicates() >>> x Head:Node(-7.5) Tail:Node(9.78) List:-7.5 -> 1 -> 3 -> 4 -> 5 -> 8.76 -> 9.78 ''' def __init__(self): # You are not allowed to modify the constructor self.head=None self.tail=None def __str__(self): # You are not allowed to modify this method temp=self.head out=[] while temp: out.append(str(temp.value)) temp=temp.next out=' -> '.join(out) return 'Head:{} Tail:{} List:{}'.format(self.head,self.tail,out) __repr__=__str__ def isEmpty(self): return self.head == None def __len__(self): count=0 current=self.head while current: current=current.next count+=1 return countdef replicate(self): # --- YOUR CODE STARTS HERE passdef removeDuplicates(self): # --- YOUR CODE STARTS HERE pass
question:
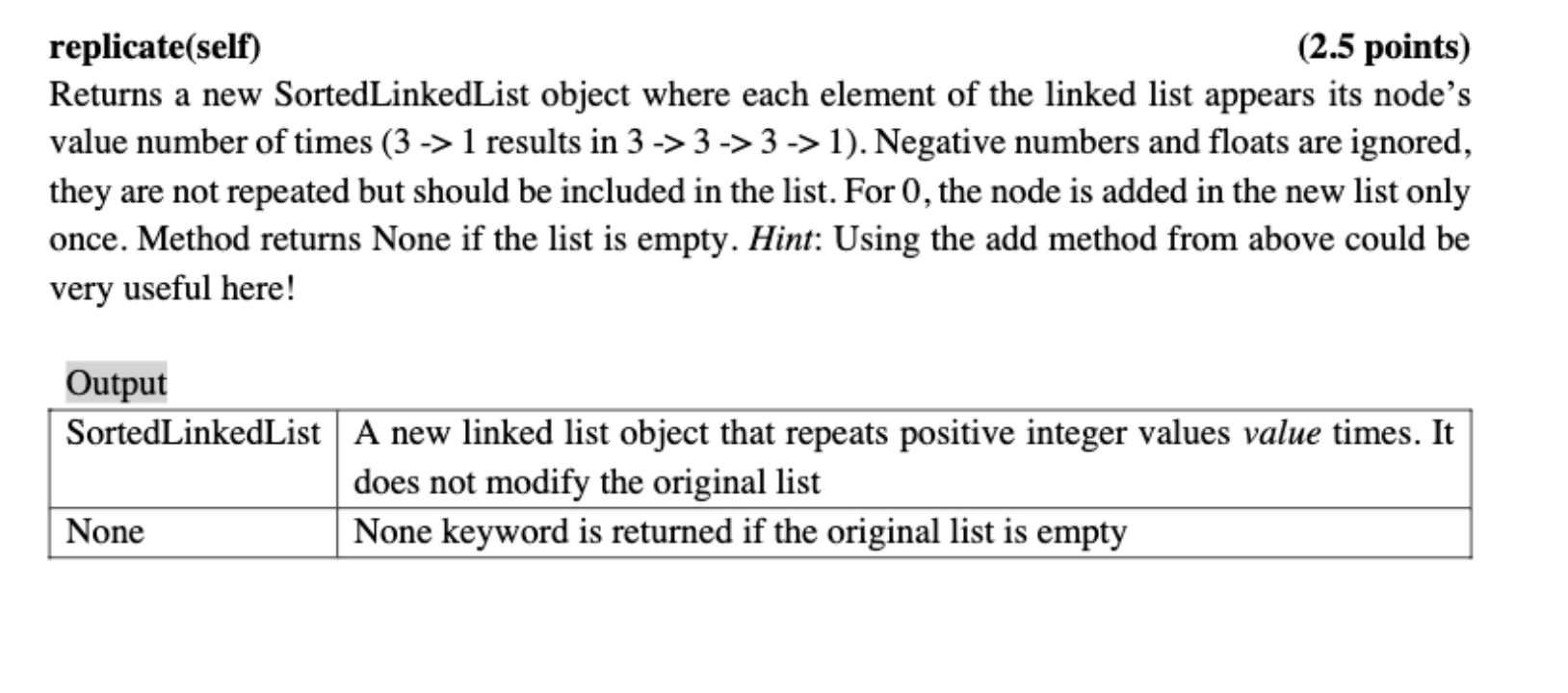
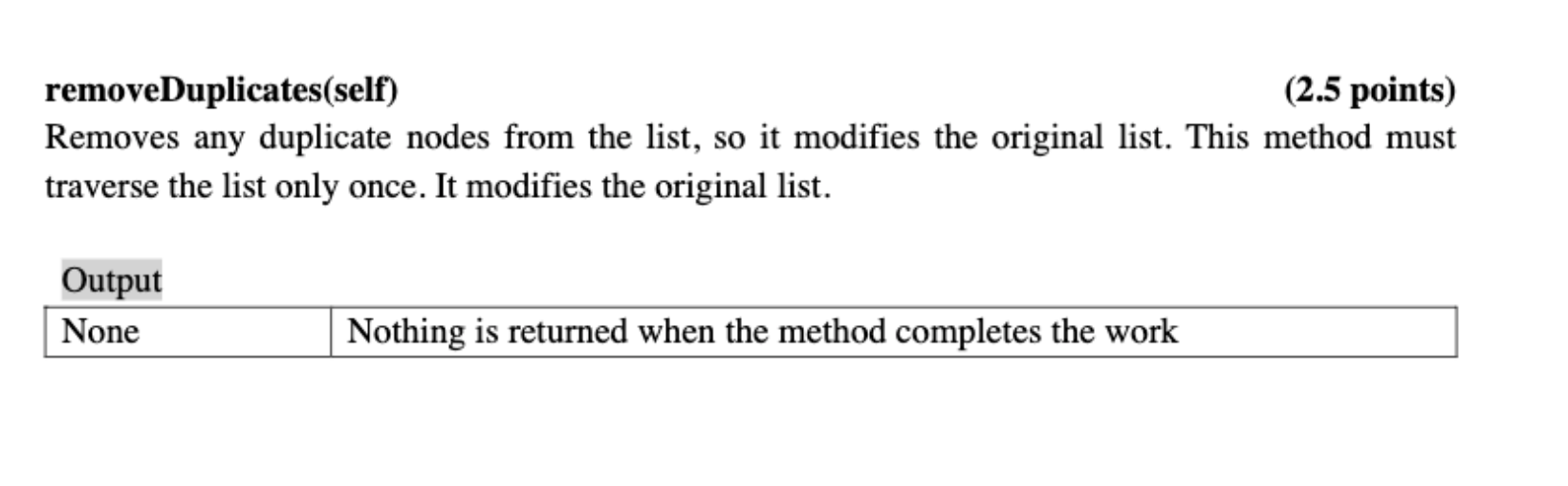
Step by Step Solution
There are 3 Steps involved in it
Step: 1
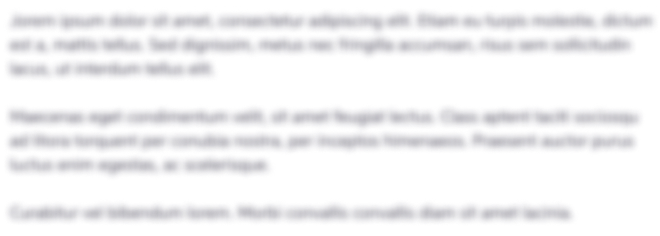
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started