Question
class Point2D { public: /* * Default Constructor * Places point at (0, 0) */ Point2D() { x = 0; y = 0; } /*
class Point2D { public: /* * Default Constructor * Places point at (0, 0) */ Point2D() { x = 0; y = 0; }
/* * Constructor * Places point at (xCoord, yCoord) */ Point2D(int xCoord, int yCoord) { x = xCoord; y = yCoord; }
int getX() { return x; }
int getY() { return y; }
void setX(int xCoord) { x = xCoord; }
void setY(int yCoord) { y = yCoord; }
void moveHorizontally(int distance) { x+=distance; }
void moveVertically(int distance) { y+=distance; }
void moveToOrigin() { x = y = 0; }
/* * Prints the current location of the point * */ void printLocation() { cout<<"Point located at ("< private: int x; int y; }; double dist(const Point2D& p1, const Point2D& p2) { return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2)); } Part 1: 1) Make a new class Point3D which inherits from the Point2D class. The class has one more data member for the z coordinate. There are two constructors, a default one and one with 3 coordinates. Add the new member functions getZ, setZ and moveAlongZ. Override moveToOrigin and printLocation to do the right thing for all 3 coordinates. Make a few Point3D objects and exercise their functionality. 2) Add a new data member, string color to your Point2D class and a new getter and setter function for color. Create a Point2D object and set its color. Then create a Point3D object and try to set its color. Is the setColor behavior available for a Point3D class? Why or why not? 3) Make a Point2D* pointer variable and point it to a newly created Point2D object. Make a Point3D* pointer variable and point it to a newly created Point3D object. Use the pointer variables to move the points and print their locations. 4) Point a Point2D pointer to a Point3D object. Does this work? Why or why not? Use the Point2D pointer to print the location of the Point3D object. What does it print? Is that what you expected for the location of a 3D point? 5) Modify the member functions so that exercise 5 behaves as expected for a Point3D 6) Explain briefly what polymorphism is and give a short example. Part 2: 1) Write a code snippet which asks the user to enter city names until "DONE" is entered and stores the cities entered in a string vector. Then print the cities that were entered but in reverse order. 2) Write a function printAll which takes as a parameter a vector of Point2D objects and prints all their locations. The function returns nothing. 3) Write a function moveAll that takes as a parameter a vector of Point2D objects, and two int parameters moveX and moveY and moves all the points by moveX and moveY. The function returns nothing. Create a vector of points in the main and add 3 points to it. Call the function to move all the points by -2, 5. Then, call the printAll function and verify that they have moved. 4) Write a function combine which takes two vectors of student names, group1 and group2 and returns a vector containing all the students in both groups. Call your function from the main with two groups you create and then print the result returned from the function. 5) Describe briefly how vectors are like arrays and how they are more powerful than arrays. Part 3: 1) Describe briefly what exceptions are and what their purpose is. What is the try block? What is the catch block? What happens with the flow of control if the catch block does not catch a thrown exception? Give a simple example. 2) Describe briefly what templates are and what their purpose is. 3) Write one line of code for each line below. What is the template type in each case? - Create a vector to store student IDs - Create a vector to store student names - Create a vector to store Point2D points - Create a set to store unique (no duplicates) student names - Create a set to store unique (no duplicates) student IDs - Create a map that maps student IDs to their score - Create a map that maps student IDs to their name - Create a map that maps student names to the name of their lab partner
Step by Step Solution
There are 3 Steps involved in it
Step: 1
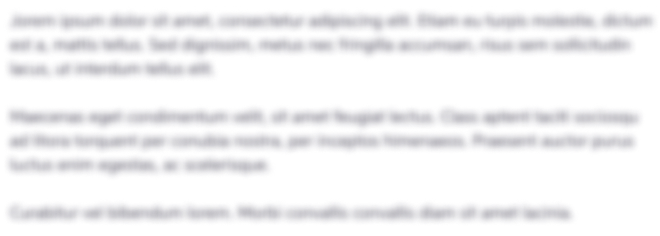
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started