Question
Code for Question #1: #include #include using namespace std; template class stackADT { public: virtual void initializeStack() = 0; //Method to initialize the stack to
Code for Question #1:
#include
#include
using namespace std;
template
class stackADT
{
public:
virtual void initializeStack() = 0;
//Method to initialize the stack to an empty state.
//Postcondition: Stack is empty
virtual bool isEmptyStack() const = 0;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty,
// otherwise returns false.
virtual bool isFullStack() const = 0;
//Function to determine whether the stack is full.
//Postcondition: Returns true if the stack is full,
// otherwise returns false.
virtual void push(const Type& newItem) = 0;
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
// is added to the top of the stack.
virtual Type top() const = 0;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element
// of the stack is returned.
virtual void pop() = 0;
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
// element is removed from the stack.
};
// -- stackType -------------------
template
class stackType: public stackADT
{
public:
const stackType
//Overload the assignment operator.
void initializeStack();
//Function to initialize the stack to an empty state.
//Postcondition: stackTop = 0
bool isEmptyStack() const;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty,
// otherwise returns false.
bool isFullStack() const;
//Function to determine whether the stack is full.
//Postcondition: Returns true if the stack is full,
// otherwise returns false.
void push(const Type& newItem);
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
// is added to the top of the stack.
Type top() const;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element
// of the stack is returned.
void pop();
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
// element is removed from the stack.
stackType(int stackSize = 100);
//constructor
//Create an array of the size stackSize to hold
//the stack elements. The default stack size is 100.
//Postcondition: The variable list contains the base
// address of the array, stackTop = 0, and
// maxStackSize = stackSize.
stackType(const stackType
//copy constructor
~stackType();
//destructor
//Remove all the elements from the stack.
//Postcondition: The array (list) holding the stack
// elements is deleted.
bool operator==(const stackType
private:
int maxStackSize; //variable to store the maximum stack size
int stackTop; //variable to point to the top of the stack
Type *list; //pointer to the array that holds the
//stack elements
void copyStack(const stackType
//Function to make a copy of otherStack.
//Postcondition: A copy of otherStack is created and
// assigned to this stack.
};
template
void stackType
{
stackTop = 0;
}//end initializeStack
template
bool stackType
{
return (stackTop == 0);
}//end isEmptyStack
template
bool stackType
{
return (stackTop == maxStackSize);
} //end isFullStack
template
void stackType
{
if (!isFullStack())
{
list[stackTop] = newItem; //add newItem to the
//top of the stack
stackTop++; //increment stackTop
}
else
cout
}//end push
template
Type stackType
{
assert(stackTop != 0); //if stack is empty,
//terminate the program
return list[stackTop - 1]; //return the element of the
//stack indicated by
//stackTop - 1
}//end top
template
void stackType
{
if (!isEmptyStack())
stackTop--; //decrement stackTop
else
cout
}//end pop
template
stackType
{
if (stackSize
{
cout
cout
maxStackSize = 100;
}
else
maxStackSize = stackSize; //set the stack size to
//the value specified by
//the parameter stackSize
stackTop = 0; //set stackTop to 0
list = new Type[maxStackSize]; //create the array to
//hold the stack elements
}//end constructor
template
stackType
{
delete [] list; //deallocate the memory occupied
//by the array
}//end destructor
template
void stackType
(const stackType
{
delete [] list;
maxStackSize = otherStack.maxStackSize;
stackTop = otherStack.stackTop;
list = new Type[maxStackSize];
//copy otherStack into this stack
for (int j = 0; j
list[j] = otherStack.list[j];
} //end copyStack
template
stackType
{
list = nullptr;
copyStack(otherStack);
}//end copy constructor
template
const stackType
(const stackType
{
if (this != &otherStack) //avoid self-copy
copyStack(otherStack);
return *this;
} //end operator=
template
bool stackType
(const stackType
otherStack) const
{
if (this == &otherStack)
return true; //same object being tested
else {
// test stackTop value
//return false if top value differs
if (stackTop != otherStack.stackTop)
return false;
//falls thru here if two stack has same length
//for loop
//return false if any value differs
for(int i = 0; i
if (list[i] != otherStack.list[i])
return false;
}
return true;
}
}
int main() {
stackType
stackType
stack1.initializeStack();
stack2.initializeStack();
stack1.push(23);
stack1.push(45);
stack1.push(38);
stack1.push(32);
stack2.push(23);
stack2.push(45);
stack2.push(38);
stack2.push(32);
if (stack1 == stack2)
cout
else
cout
cout
return 0;
}
Question #2:
Pageof 6
ZOOM
#include
#include
using namespace std;
template
class stackADT
{
public:
virtual void initializeStack() = 0;
//Method to initialize the stack to an empty state.
//Postcondition: Stack is empty
virtual bool isEmptyStack() const = 0;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty,
// otherwise returns false.
virtual bool isFullStack() const = 0;
//Function to determine whether the stack is full.
//Postcondition: Returns true if the stack is full,
// otherwise returns false.
virtual void push(const Type& newItem) = 0;
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
// is added to the top of the stack.
virtual Type top() const = 0;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element
// of the stack is returned.
virtual void pop() = 0;
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
// element is removed from the stack.
};
//Definition of the node
template
struct nodeType
{
Type info;
nodeType
};
template
class linkedStackType: public stackADT
{
public:
const linkedStackType
(const linkedStackType
//Overload the assignment operator.
bool isEmptyStack() const;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty;
// otherwise returns false.
bool isFullStack() const;
//Function to determine whether the stack is full.
//Postcondition: Returns false.
void initializeStack();
//Function to initialize the stack to an empty state.
//Postcondition: The stack elements are removed;
// stackTop = nullptr;
void push(const Type& newItem);
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
// is added to the top of the stack.
Type top() const;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
// terminates; otherwise, the top
// element of the stack is returned.
void pop();
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
// element is removed from the stack.
linkedStackType();
//Default constructor
//Postcondition: stackTop = nullptr;
linkedStackType(const linkedStackType
//Copy constructor
~linkedStackType();
//Destructor
//Postcondition: All the elements of the stack are
// removed from the stack.
private:
nodeType
void copyStack(const linkedStackType
//Function to make a copy of otherStack.
//Postcondition: A copy of otherStack is created and
// assigned to this stack.
};
//Default constructor
template
linkedStackType
{
stackTop = nullptr;
}
template
bool linkedStackType
{
return(stackTop == nullptr);
} //end isEmptyStack
template
bool linkedStackType
{
return false;
} //end isFullStack
template
void linkedStackType
{
nodeType
while (stackTop != nullptr) //while there are elements in
//the stack
{
temp = stackTop; //set temp to point to the
//current node
stackTop = stackTop->link; //advance stackTop to the
/ext node
delete temp; //deallocate memory occupied by temp
}
} //end initializeStack
template
void linkedStackType
{
nodeType
newNode = new nodeType
newNode->info = newElement; //store newElement in the node
newNode->link = stackTop; //insert newNode before stackTop
stackTop = newNode; //set stackTop to point to the
//top node
} //end push
template
Type linkedStackType
{
assert(stackTop != nullptr); //if stack is empty,
//terminate the program
return stackTop->info; //return the top element
}//end top
template
void linkedStackType
{
nodeType
if (stackTop != nullptr)
{
temp = stackTop; //set temp to point to the top node
stackTop = stackTop->link; //advance stackTop to the
/ext node
delete temp; //delete the top node
}
else
cout
}//end pop
template
void linkedStackType
(const linkedStackType
{
nodeType
if (stackTop != nullptr) //if stack is nonempty, make it empty
initializeStack();
if (otherStack.stackTop == nullptr)
stackTop = nullptr;
else
{
current = otherStack.stackTop; //set current to point
//to the stack to be copied
//copy the stackTop element of the stack
stackTop = new nodeType
stackTop->info = current->info; //copy the info
stackTop->link = nullptr; //set the link field of the
/ode to nullptr
last = stackTop; //set last to point to the node
current = current->link; //set current to point to
//the next node
//copy the remaining stack
while (current != nullptr)
{
newNode = new nodeType
newNode->info = current->info;
newNode->link = nullptr;
last->link = newNode;
last = newNode;
current = current->link;
}//end while
}//end else
} //end copyStack
//copy constructor
template
linkedStackType
const linkedStackType
{
stackTop = nullptr;
copyStack(otherStack);
}//end copy constructor
//destructor
template
linkedStackType
{
initializeStack();
}//end destructor
//overloading the assignment operator
template
const linkedStackType
(const linkedStackType
otherStack)
{
if (this != &otherStack) //avoid self-copy
copyStack(otherStack);
return *this;
}//end operator=
int main() {
linkedStackType
//stack1.initializeStack();
stack1.push(23);
stack1.push(45);
stack1.push(38);
stack1.push(32);
while(!stack1.isEmptyStack()) {
cout
stack1.pop();
}
cout
return 0;
}
Ouestion 1 Overload theoperator for the class stackType that returns true if two stacks of the same type are the same or returns false otherwise. Write test code to test your added functions nd implementation of the overload of-and source only the test code a also the output in Canvas Question 2 Repeat same work in Question 1 but this time for the linkedStackType that returns true if two stacks of the same type are the same or returns false otherwise. Write test code to test your added functions and source only the test code and implementation of the overload of also the output in CanvasStep by Step Solution
There are 3 Steps involved in it
Step: 1
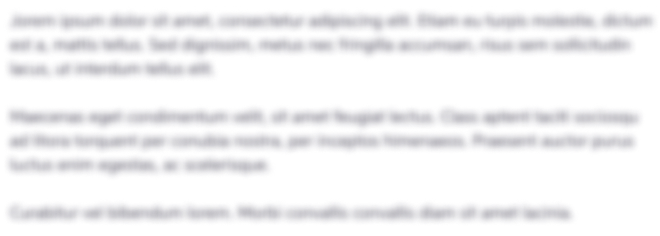
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started