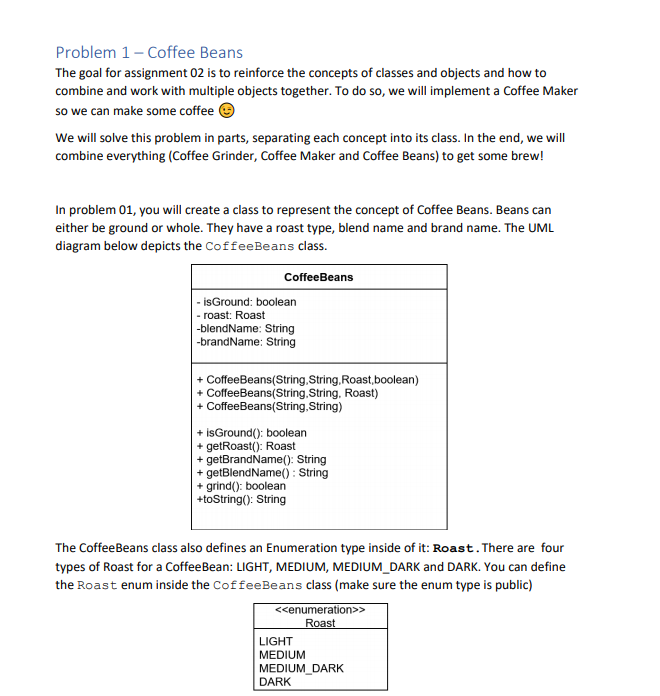
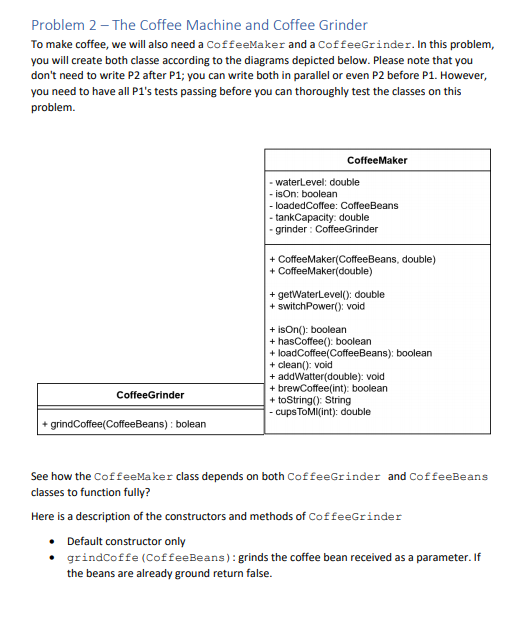
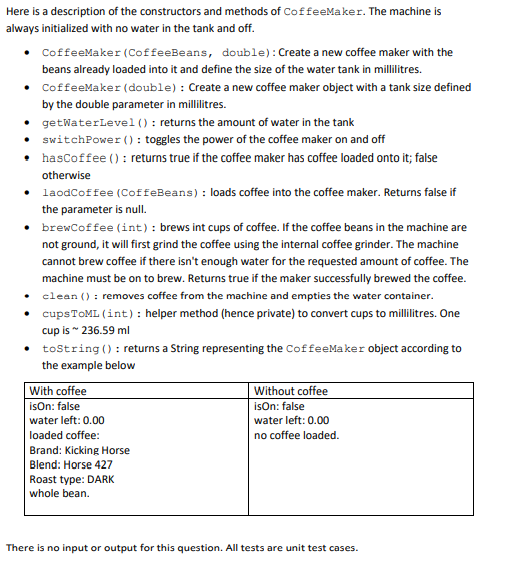
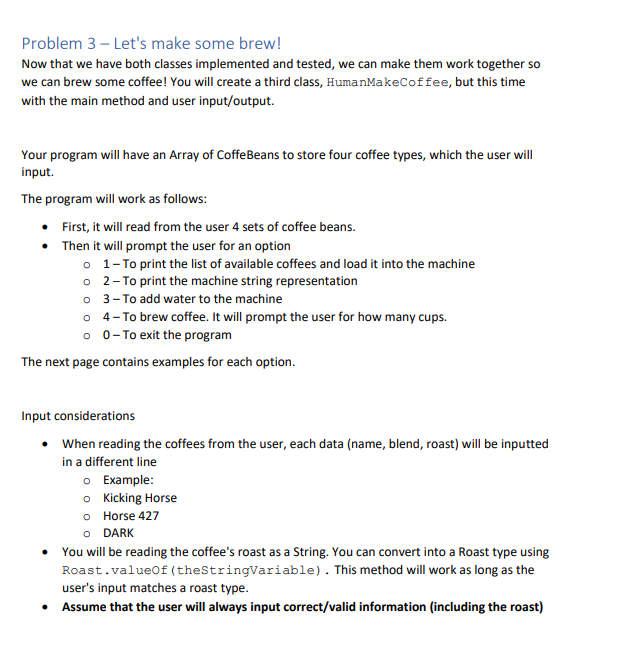
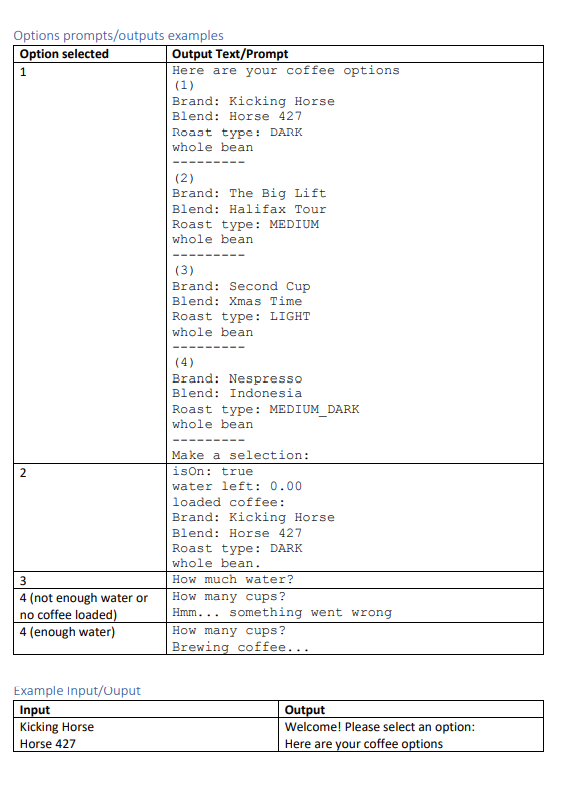
code for question 2
*///CoffeeGrinder.java////////////////// public class CoffeeGrinder { public boolean grindCoffee(CoffeeBeans beans) { return beans.grind(); } } //end of CoffeeGrinder.java/////////////
//CoffeeMaker.java//////////////////// public class CoffeeMaker { private double waterLevel; private boolean isOn; private CoffeeBeans loadedCoffee; private double tankCapacity; private CoffeeGrinder grinder; public CoffeeMaker(CoffeeBeans loadedCoffee,double tankCapacity) { this.loadedCoffee=loadedCoffee; this.tankCapacity=tankCapacity; waterLevel=0; isOn=false; grinder=new CoffeeGrinder(); } public CoffeeMaker(double tankCapacity) { this.tankCapacity=tankCapacity; this.loadedCoffee=null; waterLevel=0; isOn=false; grinder=new CoffeeGrinder(); } public double getWaterLevel() { return waterLevel; } public void switchPower() { isOn=!isOn; } public boolean isOn() { return isOn; } public boolean hasCoffee() { return loadedCoffee!=null; } public boolean loadCoffee(CoffeeBeans beans) { if(beans==null) return false; this.loadedCoffee=beans; return true; } public void clean() { if(isOn()) switchPower(); loadedCoffee=null; waterLevel=0; } public boolean brewCoffee(int cups) { if(!hasCoffee()) return false; if(loadedCoffee.isGround()==false) grinder.grindCoffee(loadedCoffee); if(getWaterLevel()
code for question1
//CoffeeBeans.java////////////////// enum Roast { LIGHT,MEDIUM,MEDIUM_DARK,DARK } //_____________________________ public class CoffeeBeans { private boolean isGround; private Roast roast; private String blendName; private String brandName; public CoffeeBeans(String brandName,String blendName,Roast roast,boolean isGround) { this.blendName=blendName; this.brandName=brandName; this.roast=roast; this.isGround=isGround; } public CoffeeBeans(String brandName,String blendName,Roast roast) { this.blendName=blendName; this.brandName=brandName; this.roast=roast; this.isGround=false; } public CoffeeBeans(String brandName,String blendName) { this.blendName=blendName; this.brandName=brandName; this.roast=Roast.MEDIUM; this.isGround=false; } public boolean isGround() { return isGround; } public Roast getRoast() { return roast; } public String getBlendName() { return blendName; } public String getBrandName() { return brandName; } public boolean grind() { if(isGround) return false; isGround=true; return true; } public String toString() { String str="Brand: "+this.brandName+" "; str+="Blend: "+this.blendName+" "; str+="Roast type: "+this.roast+" "; if(isGround) str+="ground bean"; else str+="whole bean"; return str; }
} //end of CoffeeBeans.java//////////////////
Problem 1 - Coffee Beans The goal for assignment 02 is to reinforce the concepts of classes and objects and how to combine and work with multiple objects together. To do so, we will implement a Coffee Maker so we can make some coffee We will solve this problem in parts, separating each concept into its class. In the end, we will combine everything (Coffee Grinder, Coffee Maker and Coffee Beans) to get some brew! In problem 01, you will create a class to represent the concept of Coffee Beans. Beans can either be ground or whole. They have a roast type, blend name and brand name. The UML diagram below depicts the Coffee Beans class. CoffeeBeans isGround: boolean - roast: Roast -blendName: String -brandName: String + CoffeeBeans(String, String, Roast, boolean) + CoffeeBeans(String, String, Roast) + Coffee Beans(String, String) + isGround(): boolean + getRoast(): Roast + getBrandName(): String + getBlendName(): String + grind(): boolean +toString(): String The Coffee Beans class also defines an Enumeration type inside of it: Roast. There are four types of Roast for a CoffeeBean: LIGHT, MEDIUM, MEDIUM_DARK and DARK. You can define the Roast enum inside the CoffeeBeans class (make sure the enum type is public) > Roast LIGHT MEDIUM MEDIUM DARK DARK Problem 2 - The Coffee Machine and Coffee Grinder To make coffee, we will also need a CoffeeMaker and a CoffeeGrinder. In this problem, you will create both classe according to the diagrams depicted below. Please note that you don't need to write P2 after P1; you can write both in parallel or even P2 before P1. However, you need to have all P1's tests passing before you can thoroughly test the classes on this problem. Coffee Maker - WaterLevel: double - isOn: boolean - loadedCoffee: Coffee Beans - tank Capacity: double - grinder Coffee Grinder + Coffee Maker(CoffeeBeans, double) + Coffee Maker(double) + getWaterLevel(): double + switchPower(); void + isOn(): boolean + hasCoffee(): boolean + loadCoffee(Coffee Beans): boolean + clean(): void + addWatter(double): void + brewCoffee(int): boolean + toString(): String - cups TOMI(int): double Coffee Grinder + grind Coffee Coffee Beans): bolean See how the coffeeMaker class depends on both CoffeeGrinder and CoffeeBeans classes to function fully? Here is a description of the constructors and methods of CoffeeGrinder Default constructor only grindcoffe (CoffeeBeans): grinds the coffee bean received as a parameter. If the beans are already ground return false. Here is a description of the constructors and methods of CoffeeMaker. The machine is always initialized with no water in the tank and off. CoffeeMaker (CoffeeBeans, double): Create a new coffee maker with the beans already loaded into it and define the size of the water tank in millilitres. CoffeeMaker (double): Create a new coffee maker object with a tank size defined by the double parameter in millilitres. getWaterLevel(): returns the amount of water in the tank switchPower(): toggles the power of the coffee maker on and off hasCoffee(): returns true if the coffee maker has coffee loaded onto it; false otherwise laodCoffee (CoffeBeans): loads coffee into the coffee maker. Returns false if the parameter is null. brewCoffee (int): brews int cups of coffee. If the coffee beans in the machine are not ground, it will first grind the coffee using the internal coffee grinder. The machine cannot brew coffee if there isn't enough water for the requested amount of coffee. The machine must be on to brew. Returns true if the maker successfully brewed the coffee. clean() : removes coffee from the machine and empties the water container. cupsToML (int): helper method (hence private) to convert cups to mililitres. One cup is 236.59 ml toString(): returns a String representing the CoffeeMaker object according to the example below With coffee Without coffee isOn: false isOn: false water left: 0.00 water left: 0.00 loaded coffee: no coffee loaded. Brand: Kicking Horse Blend: Horse 427 Roast type: DARK whole bean. There is no input or output for this question. All tests are unit test cases. Problem 3 - Let's make some brew! Now that we have both classes implemented and tested, we can make them work together so we can brew some coffee! You will create a third class, HumanMakeCoffee, but this time with the main method and user input/output. Your program will have an Array of CoffeBeans to store four coffee types, which the user will input. The program will work as follows: First, it will read from the user 4 sets of coffee beans. Then it will prompt the user for an option o 1 - To print the list of available coffees and load it into the machine 2- To print the machine string representation o 3 - To add water to the machine 4- To brew coffee. It will prompt the user for how many cups. 0 0- To exit the program The next page contains examples for each option. Input considerations When reading the coffees from the user, each data (name, blend, roast) will be inputted in a different line Example: o Kicking Horse o Horse 427 O DARK You will be reading the coffee's roast as a String. You can convert into a Roast type using Roast.valueof (theStringVariable). This method will work as long as the user's input matches a roast type. Assume that the user will always input correct/valid information (including the roast) 1 Options prompts/outputs examples Option selected Output Text/Prompt Here are your coffee options (1) Brand: Kicking Horse Blend: Horse 427 Roast type: DARK whole bean (2) Brand: The Big Lift Blend: Halifax Tour Roast type: MEDIUM whole bean (3) Brand: Second Cup Blend: Xmas Time Roast type: LIGHT whole bean (4) Brand: Nespresso Blend: Indonesia Roast type: MEDIUM_DARK whole bean 2 Make a selection: isOn: true water left: 0.00 loaded coffee : Brand: Kicking Horse Blend: Horse 427 Roast type: DARK whole bean. How much water? How many cups? Hmm... something went wrong How many cups? Brewing coffee... 3 4 (not enough water or no coffee loaded) 4 (enough water) Example Input/Ouput Input Kicking Horse Horse 427 Output Welcome! Please select an option: Here are your coffee options