Question
code : import java.util.ArrayList; import java.util.Collections; import java.util.List; public class PrintBinaryTree { public static void main(String[] args) { TreeNode root = new TreeNode (10); TreeNode
code :
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class PrintBinaryTree {
public static void main(String[] args) {
TreeNode
TreeNode
TreeNode
TreeNode
TreeNode
TreeNode
TreeNode
root.left = node11;
root.right = node12;
node11.left = node21;
node11.right = node22;
node12.left = node23;
node12.right = node24;
printTree(root);
}
public static
int treeMaxLevel = treeMaxLevel(root);
printNode(Collections.singletonList(root), 1, treeMaxLevel);
}
private static
if (nodes.isEmpty() || isElementsNull(nodes))
return;
int egdeLines = (int) Math.pow(2, (Math.max(treeMaxLevel - level - 1, 0)));
int spaces = (int) Math.pow(2, (treeMaxLevel - level)) - 1;
int middleSpaces = (int) Math.pow(2, (treeMaxLevel - level + 1)) - 1;
printSpaces(spaces);
List
for (TreeNode
if (treeNode != null) {
System.out.print(treeNode.value);
newNodes.add(treeNode.left);
newNodes.add(treeNode.right);
} else {
newNodes.add(null);
newNodes.add(null);
System.out.print(" ");
}
printSpaces(middleSpaces);
}
System.out.println("");
for (int i = 1; i
for (int j = 0; j
printSpaces(spaces - i);
if (nodes.get(j) == null) {
printSpaces(egdeLines + egdeLines + i + 1);
continue;
}
if (nodes.get(j).left != null)
System.out.print("/");
else
printSpaces(1);
printSpaces(i + i - 1);
if (nodes.get(j).right != null)
System.out.print("\\");
else
printSpaces(1);
printSpaces(egdeLines + egdeLines - i);
}
System.out.println("");
}
printNode(newNodes, level + 1, treeMaxLevel);
}
private static void printSpaces(int count) {
for (int i = 0; i
System.out.print(" ");
}
private static
if (treeNode == null)
return 0;
return Math.max(treeMaxLevel(treeNode.left), treeMaxLevel(treeNode.right)) + 1;
}
//to check all the elements are null
private static
for (Object object : list) {
if (object != null)
return false;
}
return true;
}
}
class TreeNode
TreeNode
T value;
public TreeNode(T value) {
this.value = value;
}
}
Aurinn w Lab Arraylsts and Trees - Word Search Xoral Xdial Design Layout References Malines Review View Help Picture Format Share Comments Fle Home Insert A Xcut Un copy Paste Format Panter A 21 Calibri Body 11 AA A A A A A BI, XA2-A- A Anbcc A:BCOCCEDE AaBbcc ABCD AaBbCal. Aalbce AaBb BbDABbcc Aabbcc 1 Console. 1 Lab Higu. Labrigu.. 1lab Goal 1 Lab Goal. 1 Lab Hub Lab Rub.. 1 Lab Rub. 1Lab Solu. 1 Lab Solu. Labak Find - 4. - Sensitivi Idhor Clipboard Paragraph Editing Voir Editar Task 5. Pre-order and post-order traversal (10 points) Implement the textbook's algorithm not the Java implementation) for pre-order and post-order traversal. To "process" ar "visit' a node means to print the data at that node. Algorithm preorder(p): perform the visit" action for position p { this happens before any recursion) for each child c in children(p) do preorderic {recursively traverse the subtree rooted at e Code Fragment 8.12: Algorithm preorder for performing the preorder traversal of a subtree rooted at position p of a tree. Algorithm postorder(p): for each child in childrent p) do postorderc) recursively traverse the subtree rooted alle perform the visit" action for position p this happens after any recursion Code Fragment 8.13: Algorithm postorder for performing the postorder traversal of a subtree rooted at position pof a tree. Thanks to Goodrich, Algorithms and Data Structures in 1,6 Edition Feel free to reuse the tree and code from Task 2 as a test case . The tree from Task 2: Pre-order traversal output: 10 6 4 8 18 15 21 Post-order traversal output: 4 8 6 15 21 18 19 Additionally, create and traverse the following trees: root right? Root edu com Type here to search O I ENG 10:35 PM 2/14/201 Aurinn w Lab Arraylsts and Trees - Word Xoral Xdial Design Layout References Malines Review View Help Picture Format Share Comments Fle Home Insert A Xcut Un copy Paste Format Panter St A T Calibri Body 11 AA A A A AA BI, XA2-A- A 2 Find - 4. Aaabcc 4:3bCocaCD. AaBbcc AoB6CD AaBbci AaBbc Aab AaEbDc AaBbccc Aabbcc 1 Console. 1 Lab Higu. Labrigu.. 1lab Goal 1 Lab Goal. 1 Lab Hub Lab Rub.. 1 Lab Rub. 1Lab Solu. 1 Lab Solu. Labak E == - Senti dhor Clipboard PASTA Editing Warn Editor Pre-order traversal output: 10 6 4 8 10 15 21 Post-order treversal output: 4 3 6 15 21 10 18 Additionally, create and traverse the following trees: root Root (1 edu com yahoo AS O google med maps Tracht THEY CIS 143-Winter 2021 Lab 6 Page 7 of 11 L Rubric Student name and today's date is a comment in the first line of the programs: -10 points if fails Screenshot and program code: -10 points if fails All lines of program output shown: 10 if fails Tree creation for two additional trees: 1 points Pre order traversal and output for all three trees: 3 points Post-order traversal and output for all three trees: 3 points Type here to search T AI 10 ENG 10:35 PM 2/14/2001 Aurinn w Lab Arraylsts and Trees - Word Search Xoral Xdial Design Layout References Malines Review View Help Picture Format Share Comments Fle Home Insert A Xcut Un copy Paste Format Panter A 21 Calibri Body 11 AA A A A A A BI, XA2-A- A Anbcc A:BCOCCEDE AaBbcc ABCD AaBbCal. Aalbce AaBb BbDABbcc Aabbcc 1 Console. 1 Lab Higu. Labrigu.. 1lab Goal 1 Lab Goal. 1 Lab Hub Lab Rub.. 1 Lab Rub. 1Lab Solu. 1 Lab Solu. Labak Find - 4. - Sensitivi Idhor Clipboard Paragraph Editing Voir Editar Task 5. Pre-order and post-order traversal (10 points) Implement the textbook's algorithm not the Java implementation) for pre-order and post-order traversal. To "process" ar "visit' a node means to print the data at that node. Algorithm preorder(p): perform the visit" action for position p { this happens before any recursion) for each child c in children(p) do preorderic {recursively traverse the subtree rooted at e Code Fragment 8.12: Algorithm preorder for performing the preorder traversal of a subtree rooted at position p of a tree. Algorithm postorder(p): for each child in childrent p) do postorderc) recursively traverse the subtree rooted alle perform the visit" action for position p this happens after any recursion Code Fragment 8.13: Algorithm postorder for performing the postorder traversal of a subtree rooted at position pof a tree. Thanks to Goodrich, Algorithms and Data Structures in 1,6 Edition Feel free to reuse the tree and code from Task 2 as a test case . The tree from Task 2: Pre-order traversal output: 10 6 4 8 18 15 21 Post-order traversal output: 4 8 6 15 21 18 19 Additionally, create and traverse the following trees: root right? Root edu com Type here to search O I ENG 10:35 PM 2/14/201 Aurinn w Lab Arraylsts and Trees - Word Xoral Xdial Design Layout References Malines Review View Help Picture Format Share Comments Fle Home Insert A Xcut Un copy Paste Format Panter St A T Calibri Body 11 AA A A A AA BI, XA2-A- A 2 Find - 4. Aaabcc 4:3bCocaCD. AaBbcc AoB6CD AaBbci AaBbc Aab AaEbDc AaBbccc Aabbcc 1 Console. 1 Lab Higu. Labrigu.. 1lab Goal 1 Lab Goal. 1 Lab Hub Lab Rub.. 1 Lab Rub. 1Lab Solu. 1 Lab Solu. Labak E == - Senti dhor Clipboard PASTA Editing Warn Editor Pre-order traversal output: 10 6 4 8 10 15 21 Post-order treversal output: 4 3 6 15 21 10 18 Additionally, create and traverse the following trees: root Root (1 edu com yahoo AS O google med maps Tracht THEY CIS 143-Winter 2021 Lab 6 Page 7 of 11 L Rubric Student name and today's date is a comment in the first line of the programs: -10 points if fails Screenshot and program code: -10 points if fails All lines of program output shown: 10 if fails Tree creation for two additional trees: 1 points Pre order traversal and output for all three trees: 3 points Post-order traversal and output for all three trees: 3 points Type here to search T AI 10 ENG 10:35 PM 2/14/2001Step by Step Solution
There are 3 Steps involved in it
Step: 1
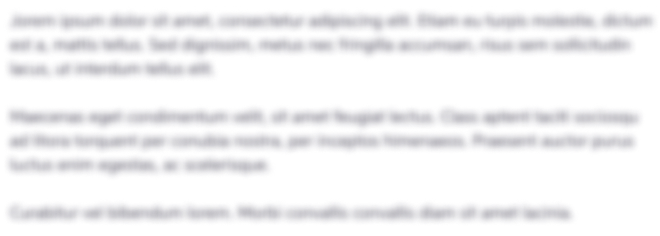
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started