Question
CODE IN C++ First modify UnsortedType based upon the following detailed instructions: Modify the UnsortedType class to use a dynamically allocated array to store the
CODE IN C++
First modify UnsortedType based upon the following detailed instructions: Modify the UnsortedType class to use a dynamically allocated array to store the items,you need to add an int field to remember the size of the array. Modify zero-parameter constructor so that it allocates an array of size 10 (default size) Add a constructor that takes an int as parameter, specifying the size of array to allocate Add a destructor for the class (why do you need to do so?) Modify IsFull( ):
It will return true, if and only if, the array is filled and an attempt to allocate larger array fails. This means that if array if not full, or if array is full but we could allocate a larger array, then the method return false.
Overload index operator (i.e., []) to return the reference of i-th element in the list Modify PutItem( ) so that it can be called to put an item into a full list:
1. Allocate a larger array (which can be double of current size) 2. Copy current array elements to the new array 3. Delete current array 4. insert item into new array
5. set info points to new array
CODE TO MODIFY:
UnsortedType::UnsortedType() { length = 0; } bool UnsortedType::IsFull() const { return (length == MAX_ITEMS); } int UnsortedType::GetLength() const { return length; } ItemType UnsortedType::GetItem(ItemType item, bool& found) { bool moreToSearch; int location = 0; found = false; moreToSearch = (location < length); while (moreToSearch && !found) { switch (item.ComparedTo(info[location])) { case LESS : case GREATER : location++; moreToSearch = (location < length); break; case EQUAL : found = true; item = info[location]; break; } } return item; } void UnsortedType::MakeEmpty() { length = 0; } void UnsortedType::PutItem(ItemType item) { info[length] = item; length++; } void UnsortedType::DeleteItem(ItemType item) { int location = 0; while (item.ComparedTo(info[location]) != EQUAL) location++; info[location] = info[length - 1]; length--; } void UnsortedType::ResetList() { currentPos = -1; } ItemType UnsortedType::GetNextItem() { currentPos++; return info[currentPos]; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
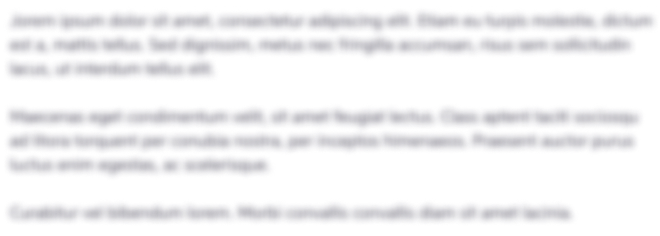
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started