Question
Code in c++. please provide a bug free easy to understand code. please write comments. CS1337 Homework #9 Assigned April 4, due April 11 at
Code in c++. please provide a bug free easy to understand code. please write comments.
CS1337
Homework #9
Assigned April 4, due April 11 at 11:59 PM
This homework assignment gives you the opportunity to practice structures, classes, dynamic array creation, use of overloaded operators.
HW9 (Graded out of 100)
Your program will read from an input file that contains account records. Each account record starts with a delimiter, which is the '#' character, followed by the account number, followed by the number of owners, followed by one or more owner records (there is one record for each owner), followed by the number of transactions, followed by one or more transaction records (there is one record for each transaction). An owner record consists of the owner's name followed by the owner's DOB in mm/dd/yyyy format, followed the owner's address. A transaction record consists of the transaction date, followed by the transaction type (1 for account creation, 2 for deposit, and 3 for withdrawal), followed by the amount. The various fields are separated by a white space, except for the owner's name and address, which are surrounded by a new line. Below is an example of account record. The // comments are added for clarification, but are not part of the file content.
#
1200 // account number
2 // number of owners
Kirk // owner's name
02/12/2200 // owner's DOB
Enterprise // owner's address
Mr. Spock // owner's name
03/13/2300 // owner's DOB
Galaxy Way // owner's address
2 // number of transactions
02/15/2320 3 100 // first transaction record
02/11/2320 1 200 // second transaction record
Your program should determine the number of account records in the file, dynamically create an array of Account of size equal to the number of account records (note this is an array of Account, not an array of pointers to Account), and populate the array with the data read from the file. Finally it should display for each account, the owner's data and the transactions data sorted according to their dates (the oldest transaction should be listed first).
Your program should implement the following:
Date class
This class is as defined in HW8, implement in-line in Date.h
Account class
This class is adapted from HW7. The additions with respect to HW7 are underlined. Implement in-line in Account.h
struct Transaction
Date transactionDate; int type; double amount;
};
struct Person {
string name; Date DOB; string address;
};
The class Account has the following private member variables:
accountNumber of type int numOwners of type int ownerPtr of type Person * balance of type double
numTransactions of type int // Number of transactions for the account
transacPtr of type Transaction * // Pointer to an array that holds the Transactions
and the following public member functions:
void setOwner(int, Person): as in HW7 Person getOwner(int) const: as in HW7 int getAccountNumber() const: as in HW7 double getBalance() const: as in HW7 int getNumOwners() const: as in HW7
The public member functions new with respect to HW7 are defined as follows.
void set(int accountNumber , int numOwners ) : mutator that sets the accountNumber and numOwners, dynamically creates an array of Person of size numOwners, and assign to ownerPtr. Sets balance to zero.
void setTransacPtr(Transaction * transacPtr ) : mutator that sets transacPtr to transacPtr int getNumTransactions() const: accessor for numTransactions
void setNumTransactions(int numTransactions ) : mutator that sets numTransactions to numTransactions
Transaction getTransaction(int ind) const: accessor that returns the Transaction at index ind of the transacPtr array
Stand-alone Functions (in main.cpp)
You should implement the following stand-alone functions.
/* This function takes as argument a string representing a date in the mm/dd/yyyy format and returns the month as an int
The argument string is assumed properly formatted and no input validation is required */
int getMonth(string d)
{
// Function body
}
/* This function takes as argument a string representing a date in the mm/dd/yyyy format and returns the day as an int
The argument string is assumed properly formatted and no input validation is required */
int getDay(string d)
{
// Function body
}
/* This function takes as argument a string representing a date in the mm/dd/yyyy format and returns the year as an int
The argument string is assumed properly formatted and no input validation is required */
int getYear(string d)
{
// Function body
}
/* This function reads from an account file and populates an array of Account objects (not an array of pointers to Account) with all the owners and transactions data read from the file. The owner data is the owner's name, DOB and address. The transaction data is the transaction date, transaction type (account creation, deposit, withdrawal), and transaction amount. For each account, the function should dynamically allocate an array of Transaction of size equal to the number of transactions, assign the array's address to transacPtr, and populate the array with the transaction data. In the array, the transactions should be sorted by date (oldest first).
It takes as arguments the file name, the array and the size of the array.
It returns true if file open is successful, false otherwise. It should close the file when done */
bool readAccounts(string fname, Account accntPtr [], int numAccnts)
{
// Function body
}
/* This function reads from a file to determine the number of accounts It returns -1 if file open is unsuccessful, else it returns the number of accounts */
int determineNumAccounts(string fname)
{
// Function body
}
Outline of main
Call determineNumAccounts to determine the number of account records in the file
Dynamically create an array of Account of size equal to the number of accounts, to hold the Account objects (note this is an array of Account, not an array of pointers to Account),
Call readAccounts to read data from the file and populate the array of Accounts.
Read the populated array of Accounts to display for each account the account number, along with the data for all the owners, and the data for all the transactions. The owner data is the owner's name, DOB and address. The transaction data is the transaction date, transaction type (account creation, deposit, withdrawal), and transaction amount. Additionally, the new balance resulting from each transaction is displayed. The transactions should be listed by date, the oldest transaction being listed first.
Additional Requirements
What to turn in
main.cpp
Date.h - All the member functions of Date are in line
Account.h - All the member functions of Account are in line
Style
Make sure you follow the style requirements, especially regarding the comment header for functions, to avoid losing points.
Suggestions for implementation
a) Read data from file
You may assume the data records in the file are properly formatted (no need to do input validation)
Assuming inFile is your file stream object, inFile >> .... will read the date like cin >> ....That is, it will leave a left over new line character in the buffer. To flush the left over character from the buffer, you can use inFile. ignore () , which works similarly to cin.ignore() .
getline(inFile, ... ) will read the data like getline(cin, ... ). That is, it will read the whole line (including spaces) until a new line is found.
You may assume the transactions in the file are a log of past transactions which have been actually executed, and for which there was no error such as excessive withdrawal amount, negative deposit amount, incorrect account number, etc.
b) Sorting of Transaction
You will need to sort the Transaction according to their dates. You may adapt the bubble or insertion sort algorithms, and use the overloaded < operator for Date.
Expected Output
Use the "f.txt" file to test your program.
Enter the account file name: f.txt There are 3 accounts in the file
Account Number: 1100
Name: Scotty, DOB: January 11, 2100, Address: Enterprise
Transaction history:
Transaction #1
Date: January 11, 2120, type: account creation, amount: 100, new balance: 100 Transaction #2
Date: January 15, 2120, type: withdrawal, amount: 50, new balance: 50 Transaction #3
Date: January 21, 2120, type: deposit, amount: 200, new balance: 250 Account Number: 1200
Name: Kirk , DOB: February 12, 2200, Address: Enterprise
Name: Mr. Spock , DOB: March 13, 2300, Address: Galaxy Way Transaction history:
Transaction #1
Date: February 11, 2320, type: account creation, amount: 200, new balance: 200 Transaction #2
Date: February 15, 2320, type: withdrawal, amount: 100, new balance: 100 Account Number: 1300
Name: H Solo , DOB: April 14, 2400, Address: Millenium Falcon Name: Chewbacca , DOB: May 15, 2500, Address: Chewys home Name: C-3P0 , DOB: June 16, 2600, Address: Milky Way
Transaction history:
Transaction #1
Date: March 11, 2620, type: account creation, amount: 300, new balance: 300 Transaction #2
Date: March 12, 2620, type: withdrawal, amount: 200, new balance: 100 Transaction #3
Date: March 16, 2630, type: deposit, amount: 500, new balance: 600
Process returned 0 (0x0) execution time : 2.584 s Press any key to continue.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
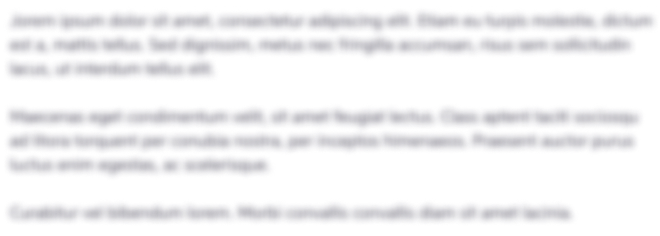
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started