Question
Code in JAVA In this part, students are required to implement a password salt verification system. A salt is stored in the database and added
Code in JAVA
In this part, students are required to implement a password salt verification system. A salt is stored in the database and added to the hashing process to force the uniqueness of the password, which is easy to verify and can increase the complexity without increasing user requirements. The salt does not need to be kept secret and the extra security which comes in with the salt is that it even maps same passwords to different hashes depending on the salt. This renders the dictionary attacks useless which are otherwise very effective in password cracking.
Q-3) Given UID.txt and Hash.txt files, Students need to implement the Verification system, such that the given example of the password and salt can match with the hash value in the Hash.txt file.
For example, in your UID.txt file, the first UID is 001, and in your Password.txt file, the password is 0599, and in your Salt.txt file, the salt associated with the first UID is 054. When applying the MD5 Hash Function with the encode format as utf- 8 as shown in the figure, the expected output should be 4a1d6f102cd95fac33853e4d72fe1dc5 (See the first line of your hash.txt file). It is worth to mention that, the concatenation between password and salt needs to be in the format of (password||salt). For example, with the aforementioned input, the concatenation result will be 0599054. Note that, 0 should not be omitted
Requirements for the Design of Verification System:
def computeMD5hash(my_string): m = hashlib.md5() m.update(my_string.encode('utf-8')) return m.hexdigest()
A) The designed verification system should be able to correctly verify the example shown above. When the input is correct, the system will output a String The input password and salt matches the hash value in the database. Otherwise, the output should be The input password and salt does not match the hash value in the database
Q-4) Implementation of Brute Force Cracker
To reduce the complexity for cracking the password and salt, the passwords are randomly set in the range of [0000, 1000], while the salt is randomly set in the range of [000,100] for each UID. One easy idea to implement a cracker system is to brute-forcely try all possible combinations of password and salt for one UID. As the Hash.txt and UID.txt files are given, students are requested to implement a cracker system which could find the correct password and salt for a specific UID.
Requirements for the Design of Cracker System :
A) For a specific UID, the cracker system can output the correct password and salt value. For example, when input the UID as 001, the output should be password: 0599; salt: 054
UID.txt
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100
Salt.txt
054 027 079 001 049 030 008 006 080 091 047 086 058 084 055 089 049 013 053 044 019 011 084 054 050 082 056 037 089 022 046 082 069 052 044 094 054 010 073 094 100 090 086 020 056 094 028 004 064 039 096 018 041 027 022 003 024 067 077 009 095 001 015 074 038 052 006 058 043 072 099 060 025 047 076 056 013 046 026 021 024 037 066 018 098 026 015 079 078 080 044 081 081 088 045 051 060 026 063 065
Password.txt
0599 0973 0242 0771 0192 0187 0937 0825 0324 0258 0818 0111 0459 0519 0914 0200 0816 0219 0894 0087 0322 0589 0253 0604 0290 0936 0685 0319 0705 0186 0528 0227 0135 0861 0010 0799 0986 0798 0976 0635 0025 0576 0759 0808 0138 0825 0482 0856 0648 0247 0683 0898 0147 0184 0773 0637 0894 0791 0726 0027 0848 0846 0157 0656 0875 0688 0318 0768 0681 0020 0555 0679 0721 0178 0993 0741 0569 0609 0085 0766 0897 0983 0469 0790 0066 0962 0837 0367 0366 0690 0791 0455 0699 0868 0892 0497 0358 0379 0555 0821
Hash.txt
4a1d6f102cd95fac33853e4d72fe1dc5 e8e7d67256aedc225a072540540d910c 0c6a7629e1fe2eab887de89dd65072d9 0e8b4ee66ad464aee37a934d74088613 6261a6ddd461304eaed4090348d8d117 cfa0000941daff46ebf0ef1950c86db0 e09a3a07abbaa5bf3170e6d297dff065 11dcc98c009eb5b2a9449d05ea8bb381 dfbcb13e80aa4cfb872f987b17879ec8 db8a21330a299c4fcae3534cc7f1e01b 8ec4312533793e33d092978b2c847330 5ac048a9173c4d6257c05cc1a26e1d06 5cfdff9a6b2859ed5aec0808dd50524e 83936eca815f642eb11970208520e2a3 6948e63fc20c099f4837d25a061439bc aa058ea92bebaa364f0153383c202c27 f48edac418318d0580653ed629069a24 6555bd5e2356ae34906cc724994a4b07 a9154393f28581eff2ca3b84b44f8702 89bf097de1f883368ef00e652fa2ae8d 04117ec99be1bcbd9f42da3228685f26 a5eec6aa303a169368cdb7a1b7818be8 e307b0acf8b5896c62ec0372ed3b7a38 594a723309b9b0bf3ca081ef4008c709 d303dd9e216a31f4f3422877b25012c6 fc97bd45eed421fd859d0a57d3c9168c 5689bc7ae71a56ca02f4a6fbeb221a65 ecf00e96b47634d0edd411a3ecd16d45 e2e9fbc8ee758be468a2f3e8164d5995 3f34c28d953d4279e7fec04cddf69d61 7b86f90ffebabff0bc29ed460da38414 9e537436c7751a0f75acee353f4e7fa3 e8e3742add907f94d2534e6c1431c021 74f4d9a79a5a4f17e231008269d8f126 9d016be6b4b7a8e0abbd7e81d48d54ad 926183d9745a0ad9531e339cecc27c32 b7321bde18b5bcabab68dcb2a3b59d8b 89f18f046ac58603900a73c34f5c88ac c9781a494696f39198bd87f863ffd2f1 cba36fe41dd35e38934109092111cb94 8c0f135b3e798427eee61cd59a377895 4d4ab45bff506e6f8b2530a60e4fbc84 093c57314f26e564f1edb847edf691f4 6ca04238450d2d76affc85441bfba832 e05b02d5732d420bb62c3175413b8f45 ecf7f3478a26f387d045956adf3b048b 1d706464afbf3138772167e9cd5ac648 2dfb337ff8cde7515ea3d99d169b6ab3 9c45c74b86973fb81748008496e643ea 2e86861267dfd51df069325ef8586eb1 814a5a817f7cecf59321d7b1b59512eb 760d293372257cd0af2c83d33a69e992 1ad2721523dcc547cbee3d4823c5f9cc 55df2796fee19ddd3860b950350fad28 cf855b8878ca23707b21abb06e6e9bfe 0460cf935180a5fb1bee794d2166dcc8 6ee6e1f98bb64faecf719fdec5935285 711a31e3af8b42f1af1dc30b747a4043 1de0c17431522b41496f4617b34ccbb6 c6ccbdd6e0e99d15738141c0882fc703 21542a4afaa446283d9dac62816b6f4f fe3d4de9a42fc0fbc0c7c7702149ddac f8c74793ea1534a510cd4c43c6724f98 3911e13c8f3345418ec1e756e0cc2325 170df1ce6c4cf82375cdf5751324666f 06c47b7fcf6fac367bd36a833a9ac627 eb42658c3b74e64470f1ce96dca09a97 8ac8b25c4ffdd19f40be9e9f121a8400 864287113da1db156d23553e91af2bca 8a1e10f94d0478895afc263478f5367f 8d361f8aa92bd601f06a0f050533edab f0ef3ea13c7f4d2a21a59d3daae7b73e 2ead2c8aec52e72dc872df8a9989517c 35b9853368c995b693cf0d0bafed0a03 8b981b46577e36238d238c88fd5502af e5c6d48896c5b988be046f8a48951f83 49868b6ce89f70b6b9b9e8c7cc1999c2 a288f9ce0700f58d67f8ad727fd6d7e8 5c3c31e58e5cbeabd7985226cf121152 986c5cbeb003ffa0751fcaacf650794c 766d04efe8bfbfbc59bd5dd3be786450 8016329915a96453c55c92f7ee06498f ea3a2dd7805e8ba5b7a3935971d37b48 5f6930065352317503ed73554b4270aa 6c2a30349ab3254936aa5eb587706a7f a52703e5c0850231e1b3f357a3b2eb11 380b6a7fc5116344ded301fe43add105 74140677f7e93c0faf8a40c21ac21d77 69a3a2c54b7c26c51b58983d092debf8 189c892a88ad58ed15210ee2168a2d77 690b0614d891d57c4300cba80e85a234 00662a81551bacf3f8dd738e2f429eef 9774c80bb94fa9dd404519895403e113 e8294e389ce622c139aa4d7c498763de 7a59c3ad66de26084c3085d98b8393f8 cf0bfe66bc5e6fc77a9db06699a8d6c0 6a2c733c6cc3fc8a548386d9daac24d2 8e7f3ac790fbdc624e01d9ec50071752 3b3542579462ba4654040def945b11ce 0105db564c086d336422b4a033862018
Step by Step Solution
There are 3 Steps involved in it
Step: 1
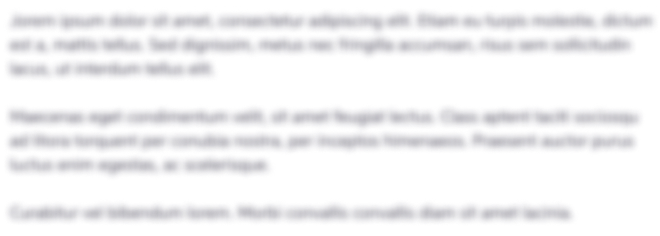
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started