Question
code in java public class Bill { /* * Instance Variables all set to private */ private String biller; private String billAcct; private double billAmtDue;
public class Bill { /* * Instance Variables all set to private */ private String biller; private String billAcct; private double billAmtDue; private String billDueDate; private String billDesc; private String billPaidDate; /* * Constructors in class Bill will contain a null constructor and a * full constructor */ public Bill( ) { // Null Constructor empty } // end of null constructor public Bill ( String owedTo, String owedFor, double owedAmt, String owedWhen, String owedDesc, String owedPaidDate ) // constructor calling set method for each instance variable { setBiller( owedTo ); setBillAcct( owedFor ); setBillAmtDue( owedAmt ); setBillDueDate( owedWhen ); setBillDesc( owedDesc ); setBillPaidDate( owedPaidDate ); } // End Full Constructor /* * declaring set Methods for class variables */ public final void setBiller( String owedTo ) { biller = owedTo; } // end setBiller public final void setBillAcct( String owedFor ) { billAcct = owedFor; } // end setBillAcct public final void setBillAmtDue ( double owedAmt ) { billAmtDue = owedAmt; } // end setBillAmtDue public final void setBillDueDate ( String owedWhen ) { billDueDate = owedWhen; } // end setBillDueDate public final void setBillDesc ( String owedDesc ) { billDesc = owedDesc; } // end setBillDesc public final void setBillPaidDate ( String owedPaidDate ) { billPaidDate = owedPaidDate; } // end setBillPaidDate /* * Declaring get Methods for class variables * */ public final String getBiller ( ) { return biller; } // end getBiller public final String getBillAcct ( ) { return billAcct; } // end getBillAcct public final double getBillAmtDue ( ) { return billAmtDue; } // end getBillAmtDue public final String getBillDueDate ( ) { return billDueDate; } // end getBillDueDate public final String getBillDesc ( ) { return billDesc; } // end getBillDesc public final String getBillPaidDate ( ) { return billPaidDate; } // end getBillPaidDate // END GET METHODS // Insering toString method and declaring it private public String toString ( ) { String time; time = String.format(\"%n%s is owed $%.2f on account %s by %s for %s. %n%s\", getBiller (), getBillAmtDue (), getBillAcct (), getBillDueDate (), getBillDesc (), getBillPaidDate () ); // if else statement to see if bill has been paid if(time.equals(\"\")) { time += \"The bill has not yet been paid.\"; } else { time += \"The bill was paid on \" +billPaidDate; } //end if else statement return time; } // END toString } //end Bill class
/*
* Lab 11
*
* This code will read in a \"flat\" file containing data items for use in instantiating Bill objects.
* A variety of Bill objects will be instantiated based on the input values.
* Those objects will be added to a (globally declared) generic collection for holding/serialization
* Once all objects have been instantiated, the (globally declared) collection will be output
* as an XML-serialized document.
*/
// ---------------------- IMPORTS for File Handling/Management ------------------------------
// -------------------- IMPORTS for Collection Handling/Management ---------------------------
import java.util.List; // Interface provides methods for manipulating objects by index
import java.util.ArrayList; // Class implements interfaces List & Collection
// --------------------------- IMPORT for XML Serialization ---------------------------------
public class CreateBillObjects_Batch
{
/*
* Declared globally, as it will be needed in both sub-methods. This avoids having to pass the reference
* among methods.
*/
private static List billsCollection = new ArrayList( );
public static void main( String[] args )
{
readInput_BuildBills( );
serializeBills( );
} // end main
private static void readInput_BuildBills( )
{
/*
* 1. Set up \"holding spaces\" for various objects and variables we will use in the loop
* 2. Declare and instantiate an object for input of the data \"flat file\" inputBills.txt
* 3. Read each line from the input file, and using the data provided, instantiate a Bill object
* 4. Add the new Bill object to the billsCollection (for later serialization)
* 5. When all input has been read, remember to close the input file!!
*/
Bill newBill; // This is a \"holding space\" for the Bill objects we instantiate
try // Because \"stuff\" can happen during I/O...
{
/*
* Declare and instantiate a Scanner object to read the input file.
*/
// Modify Scanner object to use a comma (,) as the delimiter between inputs
inputFile.useDelimiter( \",\" );
while( inputFile.hasNext( ) )
{
/*
* Read input items from the file, and use that data to instantiate a new Bill object
*/
newBill = new Bill( inputFile.next( ),
inputFile.next( ),
inputFile.nextDouble( ),
inputFile.next( ),
inputFile.next( ),
inputFile.next( ) );
// Now that the new Bill object has been instantiated, add it to the (global) collection
billsCollection.add( newBill );
} // end while loop
/*
* ALWAYS CLOSE your file!
*/
} // end try block
catch( IOException xcptn )
{
System.err.printf( \"There was an error involving the INPUT file. Refer to exception type & message for info.%n%s%n%s%n\",
xcptn.getClass( ).getName( ),
xcptn.getMessage( ) );
xcptn.printStackTrace( );
} // end catch IOException
} // end readInput_BuildBills
private static void serializeBills( )
{
/*
* Complete the XML Serialization process using the billsCollection
*
* 1. Instantiate a local copy of the \"helper\" code - this is necessary for the XML serialization
* to complete properly.
* 2. Declare and instantiate an output file
* 3. Serialize the collection
*/
/*
* Declare and instantiate a local copy of the \"helper\" code here
*/
try // Because \"stuff\" can happen during I/O...
{
/*
* Declare and instantiate a BufferedWriter object for output. Use file name \"outputBills.xml\"
*/
/*
* Copy the Bill object references from the (globally declared) collection to the collection in the
* \"helper\" code. NOTE: This does not duplicate Bill objects, but simply duplicates references to
* those objects.
*/
bills.getBills( ).addAll( billsCollection );
/*
* Execute the actual serialization process using local copy of the \"helper\" code instantiated above
* and the the BufferedWriter object declare/instantiated above
*/
} // end try
catch( IOException xcptn )
{
System.err.printf( \"There was an error involving the OUTPUT file. Refer to exception type & message for info.%n%s%n%s%n\",
xcptn.getClass( ).getName( ),
xcptn.getMessage( ) );
xcptn.printStackTrace( );
} // end catch IOException
} // end serializeBills
} // end CreateBillObjects_Batch.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
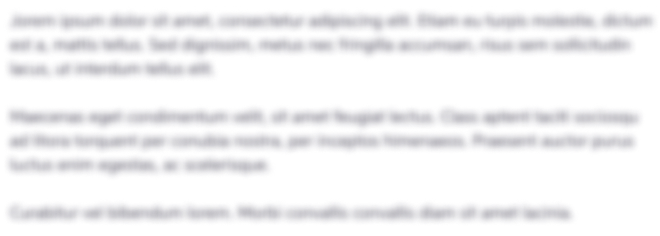
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started