Question
CODE TEMPLATE HERE #include #include #include #include using namespace std; class Time { private: int hours; int minutes; int seconds; string twoDigits(const int num) const;
#include #include #include #include
using namespace std;
class Time { private: int hours; int minutes; int seconds; string twoDigits(const int num) const;
public: void setHours(const int hrs); void setMinutes(const int mins); void setSeconds(const int secs); int getHours() const; int getMinutes() const; int getSeconds() const; string toString() const; };
class Order { private: double price; int quantity; string id; Time time; public: void setPrice(const double cost); void setQuantity(const int quant); void setID(const string ident); void setTime(const Time t); double getPrice() const; int getQuantity() const; string getID() const; Time getTime() const; void display() const; };
// FUNCTION PROTOTYPES GO HERE:
int main() { // Declare constant
vector orders;
// Call the function in TASK 6 // Write code to call the functions in TASK 13 and TASK 14 return 0; } // CLASS MEMBER FUNCTION DEFINITIONS GO HERE:
void Time::setHours(const int hrs) { hours = hrs; }
void Time::setMinutes(const int mins) { minutes = mins; }
void Time::setSeconds(const int secs) { seconds = secs; }
int Time::getHours() const { return hours; }
int Time::getMinutes() const { return minutes; }
int Time::getSeconds() const { return seconds; }
string Time::twoDigits(const int num) const { /* INSERT YOUR CODE */ }
string Time::toString() const { /* INSERT YOUR CODE */ } void Order::setPrice(const double cost) { /* INSERT YOUR CODE */ }
double Order::getPrice() const { /* INSERT YOUR CODE */ } void Order::setQuantity(const int quant) { /* INSERT YOUR CODE */ }
int Order::getQuantity() const { /* INSERT YOUR CODE */ } void Order::setID(const string ident) { /* INSERT YOUR CODE */ }
string Order::getID() const { /* INSERT YOUR CODE */ } void Order::setTime(const Time t) { /* INSERT YOUR CODE */ }
Time Order::getTime() const { /* INSERT YOUR CODE */ } void Order::display() const { /* INSERT YOUR CODE */ }
// FUNCTION DEFINITIONS GO HERE:
TEST CASE
Time time;
Order order;
time.setHours(3);
time.setMinutes(29);
time.setSeconds(9);
order.setID(\"aa\");
order.setPrice(5.5);
order.setQuantity(10);
order.setTime(time);
order.display();
Step by Step Solution
There are 3 Steps involved in it
Step: 1
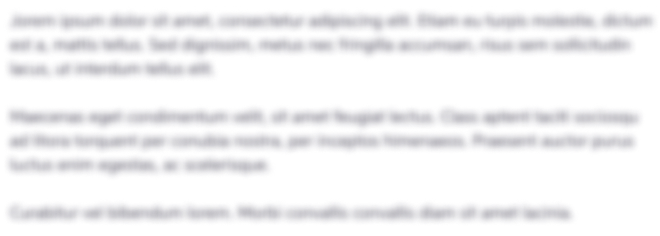
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started