Question
Code the attached Flowers Express program in C#, incorporating the modifications below. Modifications: In the code give, the Flowers Express program allows the user to
Code the attached Flowers Express program in C#, incorporating the modifications below.
Modifications: In the code give, the Flowers Express program allows the user to add records to the sales.txt file and also displays the total of the sales amounts stored in the file. Modify the program so that it also allows the user to display the contents of the sales.txt file on the computer screen (hint - add a displayFile() method to the program and to the menu choices).
#include
#include
#include
#include
#include
using namespace std;
//function prototypes
int displayMenu();
void addRecords();
void displayTotal();
void displayFile();
void deleteRecord();
int main()
{
int menuChoice = 0;
//display menu and get choice
menuChoice = displayMenu();
//call appropriate function
//or display error message
while (menuChoice != 5)
{
switch(menuChoice)
{
case 1:
addRecords();
break;
case 2:
displayTotal();
break;
case 3:
displayFile();
break;
case 4:
deleteRecord();
break;
default:
cout << "Invalid menu choice" << endl;
break;
}//end switch
//display menu and get choice
menuChoice = displayMenu();
} //end while
return 0;
} //end of main function
//*****function definitions*****
int displayMenu()
{
//displays a menu and then gets
//and returns the user's choice
int choice = 0;
cout << "Options" << endl;
cout << "1 Add Records" << endl;
cout << "2 Calculate and Display Total Balance" << endl;
cout << "3 Display File" << endl;
cout << "4 Delete Record" << endl;
cout << "5 Exit Program" << endl;
cout << "Enter menu option: ";
cin >> choice;
cin.ignore(100, ' ');
return choice;
} //end of displayMenu function
void addRecords()
{
//writes records to a sequential access file
string custID = "";
double balance = 0.0;
string status = "";
//create file object and open the file
ofstream outFile;
outFile.open("customer.txt", ios::app);
//determine whether the file was opened
if (outFile.is_open())
{
//get the name
cout << "Enter customer ID (X to stop): ";
getline(cin, custID);
transform(custID.begin(), custID.end(), custID.begin(), toupper);
while (custID != "X")
{
//get the sales amount
cout << "Enter customer balance: ";
cin >> balance;
cin.ignore(100, ' ');
cout << "Enter account status - A = active; I = inactive: ";
getline(cin, status);
transform(status.begin(), status.end(), status.begin(), toupper);
//write the record
outFile << custID << '#' << balance << '#' << status << endl;
//get another name
cout << "Enter customer ID (X to stop): ";
getline(cin, custID);
transform(custID.begin(), custID.end(), custID.begin(), toupper);
} //end while
//close the file
outFile.close();
}
else
cout << "File could not be opened." << endl;
//end if
} //end of addRecords function
void displayTotal()
{
//reads records from a file and calculates the
//total sales amount, then displays the total
//sales amount
string custID = "";
double balance = 0.0;
string status = "";
double total = 0.0;
//create file object and open the file
ifstream inFile;
inFile.open("customer.txt", ios::in);
//determine whether the file was opened
if (inFile.is_open())
{
//read a record
getline(inFile, custID, '#');
inFile >> balance;
inFile.ignore();
getline(inFile, status);
while (!inFile.eof())
{
//accumulate the balance amount for active accounts
if (status == "A" || status == "a")
{
total = total + balance;
}
//read another record
getline(inFile, custID, '#');
inFile >> balance;
inFile.ignore();
getline(inFile, status);
} //end while
//close the file
inFile.close();
//display the total sales amount
cout << setiosflags(ios::fixed) << setprecision(2);
cout << endl << "Total balance of active accounts $" << total << endl << endl;
}
else
cout << "File could not be opened." << endl;
//end if
} //end of displayTotal function
void displayFile()
{
//displays the contents of the sales.txt file
string record = "";
//create file object and open the file
ifstream inFile;
inFile.open("customer.txt", ios::in);
//determine whether the file was opened
if (inFile.is_open())
{
cout << endl;
//read a line from the file
getline(inFile, record);
while (!inFile.eof())
{
//dislay the line
cout << setiosflags(ios::fixed) << setprecision(2);
cout << record << endl;
//read another line from the file
getline(inFile, record);
} //end while
cout << endl;
//close the file
inFile.close();
}
else
cout << "File could not be opened." << endl;
//end if
} //end of displayFile function
void deleteRecord()
{
//reads records from a file, loads into an array,
//locates the record to be deleted in array,
//then rewrites all records to the file
//except the record be be deleted
string custID = "";
double balance = 0.0;
string status = "";
string recordArray[100];
string record;
int recordCount = 0;
//create file object and open the file
ifstream inFile;
inFile.open("customer.txt", ios::in);
//determine whether the file was opened
if (inFile.is_open())
{
while (!inFile.eof())
{
getline(inFile, record);
recordArray[recordCount] = record;
recordCount++;
}//end while
for (int x = 0; x < recordCount; x++)
{
cout << recordArray[x] << endl;
}
//close the input file
inFile.close();
}
//create file object for output and open the file - this overwrites the original file
ofstream outFile;
outFile.open("customer.txt");
string delCustID = "";
cout << "Enter the customer ID of the record to be deleted: " << endl;
getline(cin, delCustID);
transform(delCustID.begin(), delCustID.end(), delCustID.begin(), toupper);
cout << endl;
for (int x = 0; x < recordCount; x++)
{
//split record into fields using delimiter
string record = recordArray[x];
int delimiterPos = record.find("#", 0);
custID = record.substr(0, delimiterPos);
if (delCustID == custID)
{
cout << "Record being deleted: " << recordArray[x] << endl;
recordCount--;
}
else
{
//create file object for output file and open the file
//re-write the record
cout << "Record being written: " << recordArray[x] << endl;
outFile << recordArray[x] << endl;
}//end if
}//end for
cout << endl;
//close the output file
outFile.close();
}//end deleteRecord function
Step by Step Solution
There are 3 Steps involved in it
Step: 1
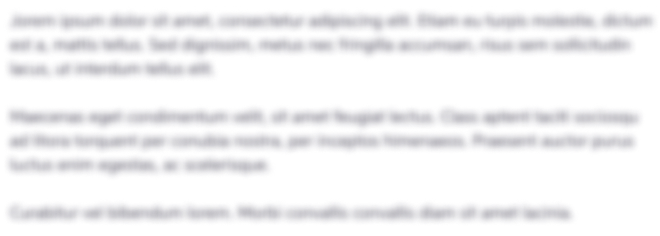
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started