Question
Coding language is Java. import imagePackage.RasterImage; import java.awt.BasicStroke; import java.awt.Color; import java.awt.Dimension; import java.awt.Graphics2D; import java.awt.Toolkit; import java.awt.geom.*; import java.awt.geom.Point2D; import java.awt.geom.Rectangle2D; import java.io.PrintStream; //
Coding language is Java.
import imagePackage.RasterImage;
import java.awt.BasicStroke; import java.awt.Color; import java.awt.Dimension; import java.awt.Graphics2D; import java.awt.Toolkit; import java.awt.geom.*; import java.awt.geom.Point2D; import java.awt.geom.Rectangle2D; import java.io.PrintStream;
// ALIEN is a COMPOSITION of a Point2D // ALIEN is an AGGREGATION of a Color
public class Alien { // functionality to draw an Alien, given a position and Graphics2D object // The expected class variables are given below private Point2D.Double pos; private Color col; private double width; private double height; private Alien() { // Restrict public use of default constructor (keep this empty, only use custom ctor's) } // custom constructors (remember to initialize ALL fields in each constructor) public Alien(Point2D.Double pos) { // create new Point2D.Double object before assigning to this.pos } public Alien(Point2D.Double pos, double width, double height, Color col) { // create new Point2D.Double object before assigning to this.pos } // copy constructor - this is necessary to create new Alien objects as duplicates of existing Alien objects // you may need this when you create aliens for the Battlefield class in Exercise 05). public Alien(Alien other) { } // mutators public void setColor(Color col) { } public void setPos(Point2D.Double pos) { } // draw method public void draw(Graphics2D gfx) { // method to draw an Alien object } @Override public String toString() { String result = "Alien: pos= " + this.pos + ", size= " + this.width + ", " + this.height; return result; }
public static void main(String[] args) { /* * Sample main method to instantiate 3 Alien objects (as per the diagram in lab2 pdf) * * The main then draws each of these in a new position, the look and feel of the Alien * object (its shape) will depend on how you define your draw method * */ PrintStream out = System.out; // HERE IS A CONVENIENT WAY TO GET ACCESS TO THE SCREEN SIZE!! // You may query the Dimension object "screen", to access the screen width and height (device space, not user space) Dimension screen = Toolkit.getDefaultToolkit().getScreenSize(); RasterImage gameBoard = new RasterImage(640,480); gameBoard.show(); Graphics2D g = gameBoard.getGraphics2D(); Alien a1 = new Alien(new Point2D.Double(100, 100)); Alien a2 = new Alien(new Point2D.Double(200, 100),100,150,Color.RED); Alien a3 = new Alien(a1); a3.setColor(Color.ORANGE); a3.setPos(new Point2D.Double(100, 300)); a1.draw(g); a2.draw(g); a3.draw(g); } }
Exercises 04 (Alien class): Alien.java In this task, using a similar methodology to that used in Exercises 01-03, create an Alien class that represents one of the attacking aliens. You have some freedom over its geometry. An Alien object should be initialized by default with a BLACK color object, and have its position defined as a Point 2D.Double object (rather than using x.y as in the Hero classes) Point2D.Double can be found in java.awt. geom. Point2D). The Alien must have at least: one custom constructor (notice that the default constructor is private, so only custom constructors) may be used to instantiate) the ability to be instantiated with an alternative color to black one "draw" method (as with the Hero classes). The draw method will display a unique shape for the Alien object (up to you to define), but the shape should be based on the position, height and width of the Alien object. The constructor for the Alien class should generate a new Point2D.Double object, and a Color object (see diagram below). An Alien only needs to be coloured a single colour (for the purpose of this lab), though you are free to make it multi-coloured if you like. See the video link at the top of this lab document for some inspiration on Alien slapes - but keep it simple. You have creative control over how you shape the geometry for your Alien. Alternatively, you can create an Alien similar to the example on the next page). Create a full UML class diagram of your Alien class (showing class variables, constructors and methods) and submit this file (as per submission at the end of this document). Alien // class variables // must have a Point2D.Double object for position Il constructors // relevant accessor/mutator methods // must have draw method // (this defines the look and shape of your Alien) Example of a simple Alien (instantiated 3 times with different size and color arguments, & drawn using shell code in Alien.java) Exercises 04 (Alien class): Alien.java In this task, using a similar methodology to that used in Exercises 01-03, create an Alien class that represents one of the attacking aliens. You have some freedom over its geometry. An Alien object should be initialized by default with a BLACK color object, and have its position defined as a Point 2D.Double object (rather than using x.y as in the Hero classes) Point2D.Double can be found in java.awt. geom. Point2D). The Alien must have at least: one custom constructor (notice that the default constructor is private, so only custom constructors) may be used to instantiate) the ability to be instantiated with an alternative color to black one "draw" method (as with the Hero classes). The draw method will display a unique shape for the Alien object (up to you to define), but the shape should be based on the position, height and width of the Alien object. The constructor for the Alien class should generate a new Point2D.Double object, and a Color object (see diagram below). An Alien only needs to be coloured a single colour (for the purpose of this lab), though you are free to make it multi-coloured if you like. See the video link at the top of this lab document for some inspiration on Alien slapes - but keep it simple. You have creative control over how you shape the geometry for your Alien. Alternatively, you can create an Alien similar to the example on the next page). Create a full UML class diagram of your Alien class (showing class variables, constructors and methods) and submit this file (as per submission at the end of this document). Alien // class variables // must have a Point2D.Double object for position Il constructors // relevant accessor/mutator methods // must have draw method // (this defines the look and shape of your Alien) Example of a simple Alien (instantiated 3 times with different size and color arguments, & drawn using shell code in Alien.java)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
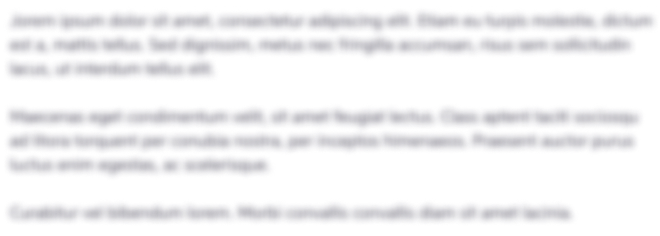
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started