Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Coding project 6 . Simulate an oversimplified predator - prey ecosystem. The goal for this programming project is to simulate an oversimplified predator - prey
Coding project Simulate an oversimplified predatorprey ecosystem.
The goal for this programming project is to simulate an oversimplified predatorprey ecosystem. Specifically, this ecosystem contains only two organisms, one being the prey and the other the predator. For the ease of reference, I will name the preys Zoomies and predators Swoopies. Note that you can name them differently in your project.
Specifically, Zoomies and Swoopies live in a world composed of a x grid of cells. Only one critter may occupy a cell at a time. The grid is enclosed, so a critter is not allowed to move off the edges of the world. Time is simulated in time steps. Each critter performs some action at every time step.
The Zoomies behave according to the following model:
Move. At every time step, randomly try to move up down, left, or right. If the neighboring cell in the selected direction is occupied or would
move the Zoomie off the grid, then the Zoomie stays in the current cell.
Breed. If a Zoomie survives for three time steps, then at the end of the time step that is after moving the Zoomie will breed. This is simulated by creating a new Zoomie in an adjacent up down, left, or right cell that is empty. If there is no empty cell available, then no breeding occurs. Once an offspring is produced, a Zoomie cannot produce an offspring until three more time steps have elapsed.
The Swoopies behave according to the following model:
Move. Every time step, if there is an adjacent Zoomie up down, left, or right then the Swoopie will move to that cell and eat the Zoomie. Otherwise, the Swoopie moves according to the same rules as the Zoomies. Note that a Swoopie cannot eat other Swoopies.
Breed. If a Swoopie survives for eight time steps, then at the end of the time step it will spawn off a new Swoopie in the same manner as the Zoomie.
Starve. If a Swoopie has not eaten a Zoomie within the last three time steps, then at the end of the third time step it will starve and die. The
Swoopie should then be removed from the grid of cells.
During one turn, all the Swoopies should move before the Zoomis do
Write a program to implement this simulation and draw the world using two different ASCII characters, for example, an o for a Zoomie and an x for a Swoopie. Create a class hierarchy with the base class Organism shown below and two derived classes Zoomie and Swoopie. The World class sets up this small ecosystem.
class Organism
friend class World; Allow world to affect organism
public:
Organism;
OrganismWorld world int x int y;
~Organism; Ask yourself: should this function be declared virtual?
virtual void breed; Whether or not to breed
virtual void move; Rules to move the critter
virtual int getType; Return if Swoopie or Zoomie
virtual bool starve; Determine if organism starves
protected:
int xy; Position in the world
bool moved; Bool to indicate if moved this turn
int breedTicks; Number of ticks since breeding
World world;
;
class World
friend class Organism; Allow Organism to access grid
friend class Swoopie; Allow Organism to access grid
friend class Zoomie; Allow Organism to access grid
public:
World;
~World;
Organism getAtint x int y; return a pointer to the critter at position xy
void setAtint x int y Organism org;
void Display; display the grid
void SimulateOneStep; simulate one step
private:
Organism gridWORLDSIZEWORLDSIZE; WORLDSIZE
;
Initialize the small world with Swoopies and Zoomies. After each time step, prompt the user to press Enter to move to the next time step. You should be able to observe a cyclical pattern between the population of predators and prey, although random perturbations may lead to the elimination of one or both species.
Demonstration:
Each learner will demonstrate your project by first compile it and then run it for time steps.
Submission: concatenate the following files into one PDF file.
DesignDoc: describe the algorithm of your World::SimulateOneStep function.
Organism.h
Organism.cpp
world.h
world.cpp
Zoomie.h
Zoomie.cpp
Swoopie.h
Swoopie.cpp
main.cpp
Can you please write a code for me for each of above mentioned files.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
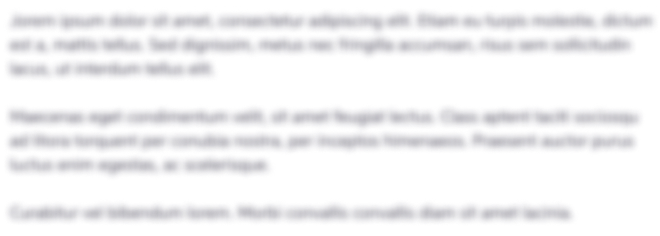
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started