Question
COMP2340: Computer Organization Lab 4 Higher level programming In this lab we will explore how the functionality implemented at the lower levels of the computer
COMP2340: Computer Organization Lab 4 Higher level programming In this lab we will explore how the functionality implemented at the lower levels of the computer system become exposed to the higher level languages that are common among most programmers today. We will also use the opportunity to experience the interface of an alternative operating system to the primary one that we have been using for most of the previous labs (if you have been using windows). The tool that we will primarily use is gcc, a C compiler that is available under most versions of linux. One of the main purported strengths of C is that it is a powerful, small language. Because of its size, it was easy to learn, and could run well on small systems. In recent versions, numerous versions of C have appeared, each with libraries included designed to solve specific problems. This introduction is therefore aimed at providing an overview of some of the key features of the language platform in a simple way. Part 1 (Introduction , making the link to the assembly)[5 marks] 1. Log on to linux, and in your home directory, make a folder called cprog (using mkdir). 2. Use a text editor to type in the following program, (substituting your name where indicated); #include ; void main() { printf("My name is and this is my first C program "); } 3. Save the program as First.C in the folder that you just created 4. Open a terminal window and navigate to the folder that you created 5. Compile the program that you just wrote by using the command gcc o first first.c (Note that without the o option, the program would have created a file named a.out, if any other executable name is required, it should be explicitly specified) 6. Run the executable by typing ./first 7. View the assembly language generated by the program by issuing the command objdump d first > out.txt a. (The objdump d command allows you to disassemble a compiled object file the redirection operator , >, sends output to a file instead of standard output (which is the screen by default) ) 8. From the dissembled file, identify the address range that the program will occupy when it is running. a. Can you determine the amount of memory required by the program from that information? (you should be able to.) 9. Execute the command ls al, and record the file size of program first. 10. Is the program size that you observe on disk the same as that you identified in question? If your answer is no, provide a justification. Part 2. Control structures and simple IO using C[10 marks] As discussed in the presentation on C programming , the control structures in C are very similar to those in java (perhaps we should be saying that the control structures in java are very similar to those in C, since C was around first). Getchar() and putchar() allow characters to be read from the keyboard and put to the screen respectively, and printf and scanf allow formatted input and output with the aid of format strings. When used for output, format strings will consist of the literal data to printed to the screen, and format codes as placeholders for variables that are to be printed. For this exercise, you will add watches to the program CALC.C (listed below, but included in the Lab4 folder) and trace it from a strategically placed breakpoint, and then make a functional change to the program. 1. Compile the program to and object named calc, ensuring that the g flag is used to allow the debugger, gdb, to work 2. Start debugging calc by issuing the command gdb calc 3. Insert a breakpoint at line 6 (by issing break 6) 4. Step through the program line by line, observing the value of the variable num by printing it at each line. 5. Modify the program CALC.C, allowing it to accept another option for the exponent to the nth power, ie , to evaluate nn. PART 3 (File access).[35 marks] The file CODER.C contains a partially implemented solution of a system that is aimed at opening a data file, and encoding it, based on a built in encryption key, and then saving the file. The file is currently missing some declarations. You will make the corrections to the file to ensure that it compiles, and then subsequently ensure that all features listed below are implemented. 1. In C, Functions can be called before they are declared. If that happens however, it is expected that the definition of the function, (formally called the prototype) will be placed before the function call. The prototype consists of the type and name of the function, as well as the types of all the arguments. Two of the needed prototypes have been included for you. Include the remaining prototypes. 2. There are a few global integer variables that need to be declared before CODER.C will successfully compile. Identify and declare them. Initialize all the newly declared variables to 0. 3. Implement the save functionality.
LAB 4 FILES.
Calc.c
#include
int main()
{
int num,answer;
unsigned int n;
char ch;
printf("Please enter a number:");
scanf("%d",&n);
printf("Enter [S] to calculate the square of the number or [F] to calculate its factorial:");
scanf("%c",&ch); /*read past the newline character from the previous entry*/
scanf("%c",&ch);
answer =1;
num = n;
if (ch=='F')
{
while (num>=1)
{
answer = answer * num;
num = num - 1;
}
printf("The factorial of %d is %d ",n, answer);
}
else
{
if (ch=='S')
{
answer = num * num;
printf("The square of %d is %d ",num, answer);
}
else
printf("Neither F nor S was entered");
}
getchar();
return 0;
}
Coder.c
#include
#include
void loadFile();
FILE * open_file(char *, char *);
char txtfile[512];
int recordsize=22;
char id[9];
char inFileName[12];
char outFileName[12];
int c;
int main ()
{
char option = '0';
id[0]= '7';
id[1]='6';
id[2]='4';
id[3]='5';
id[4]='8';
id[5]='2';
id[6]='5';
id[7]='1';
id[8]='2';
id[9]=0;
while (option !='X')
{
//system("cls");
printf("File Accessed:");
if (fileLoaded==1)
printf("%s ",inFileName);
else
printf("None ");
printf("[L]oad a file ");
if (fileLoaded==1)
{
printf("[V]iew contents ");
if (fileEncrypted==0)
printf("[E]ncrypt file ");
else
printf("[D]ecrypt file ");
printf("[S]ave file ");
}
printf("E[X]it ");
option =getchar();
switch(option)
{
case 'L':{loadFile();
break;
}
case 'V':{viewContents();
break;
}
case 'E':{processFile(1);
break;
}
case 'D':{processFile(0);
break;
}
case 'S':{saveFile();
break;
}
}
}
/*end of while statement*/
printf("End of program ");
} /*end main*/
void loadFile()
{
int ch=0;
int filepos = 0;
FILE *in;
printf("Please input file name:");
scanf("%s",inFileName);
numrecords =1;
in = open_file(inFileName, "r" );
while((ch=fgetc(in))!=EOF)
{ txtfile[filepos]=ch;
if (ch==' ')
numrecords++;
filepos++;
}
fclose ( in );
fileLoaded = 1;
printf("File has been loaded ");
// printf("Hit the enter key to continue ");
// while (!(kbhit()));
//while ((c=getchar())!=13);
}
/*end of loadfile*/
FILE *open_file ( char *file, char *mode )
{
FILE *fp = fopen ( file, mode );
if ( fp == NULL ) {
perror ( "Unable to open file" );
exit ( EXIT_FAILURE );
}
return fp;
}
void viewContents()
{
int total=0,num;
char strint[3];
/* for(currentrec=0;currentrec<=numrecords;currentrec++)*/
currentrec = 0;
printf("=========================================== ");
printf("\tFile Contents\t\t ");
printf("=========================================== ");
while (currentrec < numrecords)
{ printf("%.*s ",recordsize, txtfile+(currentrec*(recordsize+1)));
strint[0]= txtfile[20+(currentrec*(recordsize+1))];
strint[1]=txtfile[21+(currentrec*(recordsize+1))];
strint[2]=0;
// num=atoi(strint);
total+=num;
currentrec=currentrec+1;
}
if (fileEncrypted==0)
{
printf("================================================ ");
printf("File Totals\t:");
printf("%d ",total);
printf("================================================ ");
}
/*print contents, 22 characters at a time*/
/*for each row, convert last two digits to integer*/
/*print footer at the end*/
printf("Hit the enter key to continue ");
//while (!(kbhit()));
// while ((c=getchar())!=0);
}
void processFile(int mode)
{
int i,shiftval;
currentrec = 0;
while (currentrec < numrecords)
{
for ( i= 0; i <= recordsize-1;i++)
{
shiftval = id[i % 9]-48;
txtfile[i + currentrec*(recordsize+1)]= txtfile[i + currentrec*(recordsize+1)] - shiftval;
}
currentrec++;
}
fileEncrypted= 1;
printf("File contents have been encrypted ");
printf("Hit the enter key to continue ");
//while (!(kbhit()));
// while ((c=getchar())!=0);
}
void saveFile()
{
printf("Enter code that will save the file ");
printf("Hit the enter key to continue ");
// while (!(kbhit()));
//
while ((c=getchar())!=0);
}
Input.txt
John Tom :12 Jack Harris :06 Jill Hill :59 Henry teller :27 rick James :23 Amy rose :10
Step by Step Solution
There are 3 Steps involved in it
Step: 1
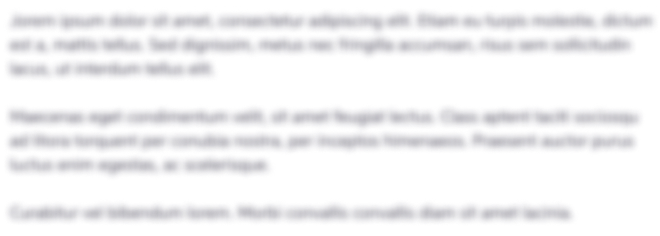
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started