Question
Complete a partial C++ program that uses Priority Queue ADT in which the internal representation of the priority queue are double-linked series of dynamically allocated
Complete a partial C++ program that uses Priority Queue ADT in which the internal representation of the priority queue are double-linked series of dynamically allocated nodes. The priority queue stores text messages read from an input file by the main{} function. The message and priority info will be stored within an object of the type Message. The text message is stored as a string attribute, and the message priority is represented with an attribute with type Priorities, an enumerated type provided.
All that is needed is the Priorityq.cpp
********Priorityq.h********
#ifndef PRIORITYQ_H #define PRIORITYQ_H
#include
// // Exception classes for PriorityQ // class EmptyPQ { /* No additional code */ }; // Exception class for empty PriorityQ condition
class FullPQ { /* No additional code */ }; // Exception class for full PriorityQ condition
class InvalidPeekPQ { /* No additional code */ }; // Exception class for invalid PriorityQ peek condition
class InvalidUIDPQ { /* No additional code */ }; // Exception class for invalid PriorityQ UID search
// // Priority Queue Node Structure // struct Node // Linked priority queue node structure { Message data; // Field for storing data in the priority queue node Node* nextPtr; // Points to next priority queue node (points from front towards rear) Node* previousPtr; // Points to previous priority queue node (points from rear towards front) };
// // PriorityQ class declaration // class PriorityQ // Double linked queue of messages sorted by priority { private: Node* frontPtr; // Points to front node of priority queue Node* rearPtr; // Points to rear node of priority queue int count; // Number of values stored in priority queue public: /********** Start of functions you must implement for PriorityQ **************/ // Implement the following nine public functions in the file named priorityq.cpp PriorityQ(); // PriorityQ() // Initializes all private variables to indicate an empty priority queue ~PriorityQ(); //~PriorityQ() // Deallocates all priority queue nodes // No memory leak allowed void MakeEmpty(); // MakeEmpty() // Deallocates all priority queue nodes and returns priority queue to empty ready-to-use state // No memory leak allowed void Enqueue(Message msg); // Enqueue() // Adds value to priority queue in correct position and increments count. // Duplicates are allowed. // Highest priority messages must always be at front of queue // Lowest priority messages must always be at rear of queue // Add AFTER messages of similar priority // If queue is already full, throws FullPQ exception.
void Dequeue(); // Dequeue() // Removes highest priority message from front of priority queue and decrements count. // If queue is empty, throws EmptyPQ exception // No memory leak allowed
void Purge(Priorities p); // Purge() // Removes all messages of priority p from queue leaving all other messages in priority queue // If queue is empty, throws EmptyPQ exception // No memory leak allowed Message Front() const; // Front() // Returns message at front of priority queue. DOES NOT MODIFY THE PRIORITY QUEUE // If queue is empty, throws EmptyPQ
Message Rear() const; // Rear() // Returns message at rear of priority queue. DOES NOT MODIFY THE PRIORITY QUEUE // If queue is empty, throws EmptyPQ
Message Peek(int n) const; // Peek() // Returns message n positions from front of priority queue. DOES NOT MODIFY THE PRIORITY QUEUE // If position n does not exist, throws InvalidPeekPQ bool IsFull() const; // IsFull() // Returns true if queue is full. Returns false otherwise. DOES NOT MODIFY THE PRIORITY QUEUE bool IsEmpty() const; // IsEmpty() // Returns true if queue is empty. Returns false otherwise. DOES NOT MODIFY THE PRIORITY QUEUE int Size() const; // Size() // Returns number of items stored in priority queue. DOES NOT MODIFY THE PRIORITY QUEUE
Message IsPresent(int uid) const; // IsPresent() // Returns message with uid if it is present in PriorityQ. DOES NOT MODIFY THE PRIORITY QUEUE // If message with specified uid does not exist, throws InvalidUIDPQ
/*********** End of functions you must implement for PriorityQ ***************/ void Print() const // Print() -- DO NOT MODIFY OR RELOCATE THIS FUNCTION // Prints contents of priority queue without modifying its contents { Node* tempPtr = frontPtr;
// Prints queue nodes Front-to-Rear order cout << "Front { "; while (tempPtr != NULL) { //cout << '(' << tempPtr->data.GetMessage() << ')' << ' '; tempPtr->data.Print(); cout << ' '; tempPtr = tempPtr->nextPtr; } cout << "} Rear Rear { ";
// Prints queue nodes Rear-to-Front order tempPtr = rearPtr; while (tempPtr != NULL) { //cout << '(' << tempPtr->data.GetMessage() << ')' << ' '; tempPtr->data.Print(); cout << ' '; tempPtr = tempPtr->previousPtr; } cout << "} Front"; } // End Print() };
#endif
********Message.h********
#ifndef MESSAGE_H #define MESSAGE_H
#include
// // Define enumerated Priorities type // enum Priorities {UNKNOWN, LOW, MEDIUM, HIGH};
// // Message class declaration // class Message // Models a single message with priority { private: Priorities priority; // Stores message priority string message; // Stores message contents int uid; // Stores message unique identifier public: /********** Start of functions you must implement for Message **************/ // Implement the following five public functions in the file named message.cpp Message(); // Initializes message to empty string with UNKNOWN priority
void SetPriority(Priorities p); // Sets priority equal to p
void SetMessage(string msg); // Sets message equal to msg
void SetUID(int messageid); // Sets uid to equal messageid. Assumes uid will be unique. Priorities GetPriority() const; // Returns priority value without modification
string GetMessage() const; // Returns message contents without modification
int GetUID() const; // Returns uid value without modification /*********** End of functions you must implement for Message ***************/ void Print() const // DO NOT MODIFY OR RELOCATE THIS FUNCTION { cout << "[";
cout << uid << ", "; if (priority == HIGH) cout << "H"; else if (priority == MEDIUM) cout << "M"; else if (priority == LOW) cout << "L"; else cout << "U"; cout << ", " << message << "]"; } // End Print() const };
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
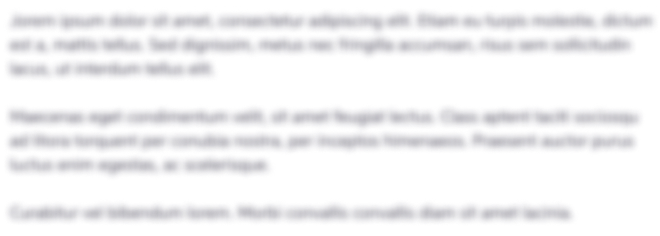
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started