Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Complete in C + + . 1 . Your focus in this assignment will on the implementation of the Polynomial class in files polynomial.h and
Complete in C Your focus in this assignment will on the implementation of the Polynomial class in files polynomial.h and polynomial.cpp This class stores the set of coefficients that define a polynomial egxxxx o You must not change the private data members in polynomial.hYou may, if you wish, add additional private function members if doing so would aid you in completing the implementation. o You may need to change some of the public functions in polynomial.h Your code will be evaluated both on its ability to function correctly within the polyfactor application the systems tests and on its ability to pass the various unit tests provided. Files that you may change: polynomial.h polynomial.cpp Attached is polynomial.h #ifndef POLYNOMIALH #define POLYNOMIALH #include #include #include "term.h class Polynomial public: Polynomial; Polynomial int b int a ; Polynomial Term term; Polynomial int nCoeff, int coeff; int getCoeffint power const; int getDegree const; Polynomial operatorconst Polynomial& p const; Polynomial operatorint scale const; Polynomial operatorTerm term const; void operatorint scale; Polynomial operatorconst Polynomial& p const; private: int degree; int coefficients; void normalize; friend std::ostream& operatorstd::ostream& const Polynomial&; ; std::ostream& operatorstd::ostream& const Polynomial&; inline Polynomial operatorint by const Polynomial& p return p by; #endif Attached is polynomial.cpp #include "polynomial.h #include using namespace std; Polynomial::Polynomial : degree coefficientsnullptr Polynomial::Polynomial int b int a : degree coefficientsnew int coefficients b; coefficients a; normalize; Polynomial::Polynomial Term term : degreetermpower coefficientsnew inttermpower for int i ; i degree; i coefficientsi; coefficientsdegree term.coefficient; normalize; Polynomial::Polynomial int nC int coeff : degreenC coefficientsnew intnC for int i ; i degree; i coefficientsi coeffi; normalize; void Polynomial::normalize while degree && coefficientsdegree degree; int Polynomial::getDegree const return degree; int Polynomial::getCoeffint power const if power && power degree return coefficientspower; else return ; Polynomial Polynomial::operatorconst Polynomial& p const if degree pdegree return Polynomial; int resultSize maxdegree pdegree; int resultCoefficients new intresultSize; int k ; while k getDegree && k pgetDegree resultCoefficientsk coefficientsk pcoefficientsk; k; for int i k; i getDegree; i resultCoefficientsi coefficientsi; for int i k; i pgetDegree; i resultCoefficientsi pcoefficientsi; Polynomial resultresultSize resultCoefficients; delete resultCoefficients; return result; Polynomial Polynomial::operatorint scale const if degree return Polynomial; Polynomial result this; for int i ; i degree; i result.coefficientsi scale coefficientsi; result.normalize; return result; Polynomial Polynomial::operatorTerm term const if degree return Polynomial; int results new intdegree term.power; for int i ; i term.power; i resultsi; for int i ; i degree ; i resultsiterm.power coefficientsi term.coefficient; Polynomial result degree term.power, results; delete results; return result; void Polynomial::operatorint scale if degree return; for int i ; i degree; i coefficientsi scale coefficientsi; normalize; Polynomial Polynomial::operatorconst Polynomial& denominator const if degree denominator.degree return Polynomial; if this Polynomial return this; if denominatorgetDegree getDegree return Polynomial; int resultSize degree denominator.degree ; int results new intresultSize; for int i ; i resultSize; i resultsi; Polynomial remainder this; for int i resultSize; i ; i int remainderstCoeff remainder.getCoeffidenominator.getDegree; int denominator.getDegree; int denominatorstCoeff denominator.getCoeffdenominatorgetDegree; if remainderstCoeff denominatorstCoeff resultsi remainderstCoeff denominatorstCoeff; Polynomial subtractor denominator Term resultsi i; remainder remainder subtractor; else break; if remainder Polynomial Polynomial result resultSize results; delete results; return result; else delete results; return Polynomial;
Complete in C
Your focus in this assignment will on the implementation of the Polynomial class in files polynomial.h and polynomial.cpp This class stores the set of coefficients that define a polynomial egxxxx
o You must not change the private data members in polynomial.hYou may, if you wish, add additional private function members if doing so would aid you in completing the implementation.
o You may need to change some of the public functions in polynomial.h
Your code will be evaluated both on its ability to function correctly within the polyfactor application the systems tests and on its ability to pass the various unit tests provided.
Files that you may change: polynomial.h polynomial.cpp
Attached is polynomial.h
#ifndef POLYNOMIALH
#define POLYNOMIALH
#include
#include
#include "term.h
class Polynomial
public:
Polynomial;
Polynomial int b int a ;
Polynomial Term term;
Polynomial int nCoeff, int coeff;
int getCoeffint power const;
int getDegree const;
Polynomial operatorconst Polynomial& p const;
Polynomial operatorint scale const;
Polynomial operatorTerm term const;
void operatorint scale;
Polynomial operatorconst Polynomial& p const;
private:
int degree;
int coefficients;
void normalize;
friend std::ostream& operatorstd::ostream& const Polynomial&;
;
std::ostream& operatorstd::ostream& const Polynomial&;
inline
Polynomial operatorint by const Polynomial& p
return p by;
#endif
Attached is polynomial.cpp
#include "polynomial.h
#include
using namespace std;
Polynomial::Polynomial
: degree coefficientsnullptr
Polynomial::Polynomial int b int a
: degree coefficientsnew int
coefficients b;
coefficients a;
normalize;
Polynomial::Polynomial Term term
: degreetermpower coefficientsnew inttermpower
for int i ; i degree; i
coefficientsi;
coefficientsdegree term.coefficient;
normalize;
Polynomial::Polynomial int nC int coeff
: degreenC coefficientsnew intnC
for int i ; i degree; i
coefficientsi coeffi;
normalize;
void Polynomial::normalize
while degree && coefficientsdegree
degree;
int Polynomial::getDegree const
return degree;
int Polynomial::getCoeffint power const
if power && power degree
return coefficientspower;
else
return ;
Polynomial Polynomial::operatorconst Polynomial& p const
if degree pdegree
return Polynomial;
int resultSize maxdegree pdegree;
int resultCoefficients new intresultSize;
int k ;
while k getDegree && k pgetDegree
resultCoefficientsk coefficientsk
pcoefficientsk;
k;
for int i k; i getDegree; i
resultCoefficientsi coefficientsi;
for int i k; i pgetDegree; i
resultCoefficientsi pcoefficientsi;
Polynomial resultresultSize resultCoefficients;
delete resultCoefficients;
return result;
Polynomial Polynomial::operatorint scale const
if degree
return Polynomial;
Polynomial result this;
for int i ; i degree; i
result.coefficientsi scale coefficientsi;
result.normalize;
return result;
Polynomial Polynomial::operatorTerm term const
if degree
return Polynomial;
int results new intdegree term.power;
for int i ; i term.power; i
resultsi;
for int i ; i degree ; i
resultsiterm.power coefficientsi term.coefficient;
Polynomial result degree term.power, results;
delete results;
return result;
void Polynomial::operatorint scale
if degree
return;
for int i ; i degree; i
coefficientsi scale coefficientsi;
normalize;
Polynomial Polynomial::operatorconst Polynomial& denominator const
if degree denominator.degree
return Polynomial;
if this Polynomial
return this;
if denominatorgetDegree getDegree
return Polynomial;
int resultSize degree denominator.degree ;
int results new intresultSize;
for int i ; i resultSize; i
resultsi;
Polynomial remainder this;
for int i resultSize; i ; i
int remainderstCoeff remainder.getCoeffidenominator.getDegree;
int denominator.getDegree;
int denominatorstCoeff denominator.getCoeffdenominatorgetDegree;
if remainderstCoeff denominatorstCoeff
resultsi remainderstCoeff denominatorstCoeff;
Polynomial subtractor denominator Term resultsi i;
remainder remainder subtractor;
else
break;
if remainder Polynomial
Polynomial result resultSize results;
delete results;
return result;
else
delete results;
return Polynomial;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
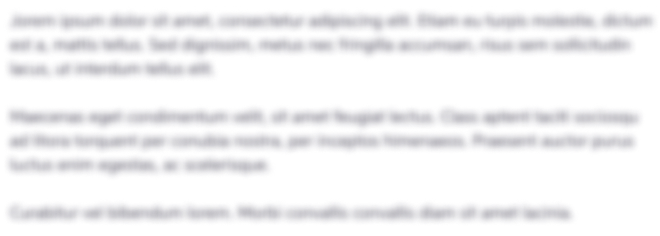
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started