Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Complete in Python. Attached are the two Python files given. Attached is parseTempsDemo.py: # ! / usr / bin / env python 3 import sys
Complete in Python.
Attached are the two Python files given.
Attached is parseTempsDemo.py:
#usrbinenv python
import sys
from parsetemps import parserawtemps
def main:
This main function serves as the driver for the demo. Such functions
are not required in Python. However, we want to prevent unnecessary module
level ie global variables.
inputtemps sysargv
with openinputtemps, r as tempsfile:
#
# Output raw structure
#
for tempsasfloats in parserawtempstempsfile:
printtempsasfloats
with openinputtemps, r as tempsfile:
#
# Split data
#
for tempsasfloats in parserawtempstempsfile:
time, coredata tempsasfloats
printftime coredata
with openinputtemps, r as tempsfile:
#
# Split Data
#
times
coredata
coredata
coredata
coredata
for time, coredata in parserawtempstempsfile:
times.appendtime
coredata.appendcoredata
coredata.appendcoredata
coredata.appendcoredata
coredata.appendcoredata
printftimes:
printfcoredata:
for time, temps in listziptimes coredata, coredata, coredata, coredata::
printftimetemps
with openinputtemps, r as tempsfile:
#
# Split Data, but Better!
#
times
coredata for in range
for time, rawcoredata in parserawtempstempsfile:
times.appendtime
for coreidx, reading in enumeraterawcoredata:
coredatacoreidxappendreading
for time, temps in listziptimescoredata::
printftimetemps
if namemain:
main
Attached is parsetemps.py:
#usrbinenv python
This module is a collection of input helpers for the CPU Temperatures Project.
All code may be used freely in the semester project, iff it is imported using
import parsetemps or from parsetemps import where
represents one or more functions.
import re
from typing import TextIO Iterator, List, Tuple
def parserawtempsoriginaltemps: TextIO,
stepsize: int IteratorTuplefloat Listfloat:
Take an input file and timestep size and parse all core temps.
Args:
originaltemps: an input file
stepsize: timestep in seconds
Yields:
A tuple containing the next time step and a List containing n core
temps as floating point values where n is the number of CPU cores
splitre recompilers$
for step, line in enumerateoriginaltemps:
yield step stepsize
floatentry for entry in splitresplitline if lenentry
Your program must accept an input filename as the first command line argument. Your program must NOT prompt the user for a filename.
All code must be properly and fully documented using a language appropriate comment style. All functions including parameters and return types must be documented.
Input Format:
Data takes the form of temperatures in a txt file. All data points are whitespace delimited.
Attached is the input file inputtemps:
Each line represents temperature readings from processor cores. Readings are taken every seconds. In this example:
line is sec
line is sec
line is sec
line is sec
line is sec
We need to look at each of the four cores independently. This means that each input file is really four sets of temperature data.
Output Format:
All output must be written to text files one file per core Each line must take the form:
xk x xk ; yi c cx ; type
where
xk and xk are the domain in which yk is applicable
yk is the kth function
type is interpolation
For the example data from the input file would generate output files.
basenamecoretxtbasenamecoretxtbasenamecoretxtbasenamecoretxt
where basename is the input file name without the extension eg without the txt or dat
Expected Output:
Given the fiveline input file inputtemps:
we would end up with four output files...
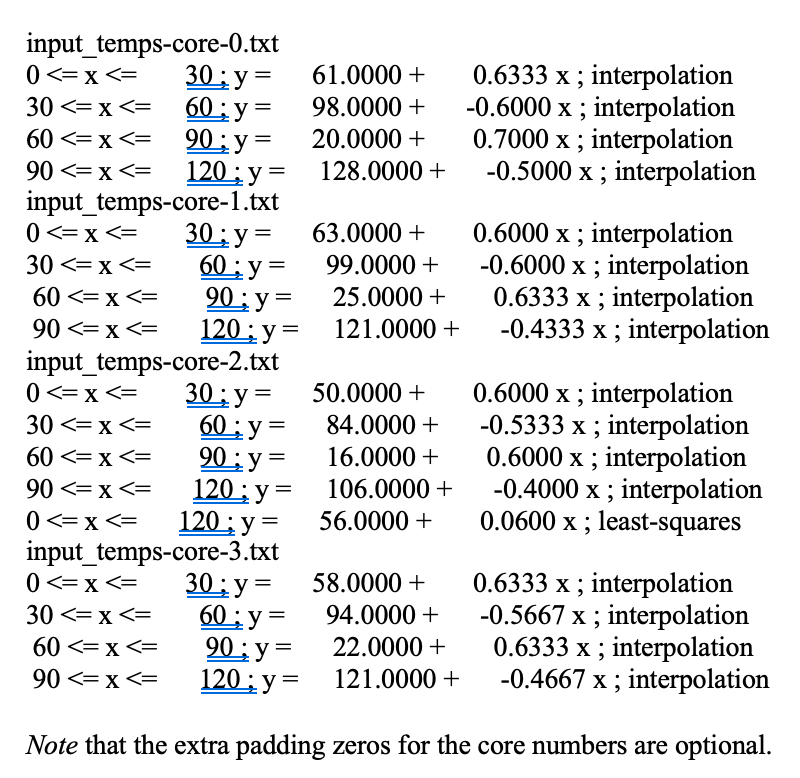
Step by Step Solution
There are 3 Steps involved in it
Step: 1
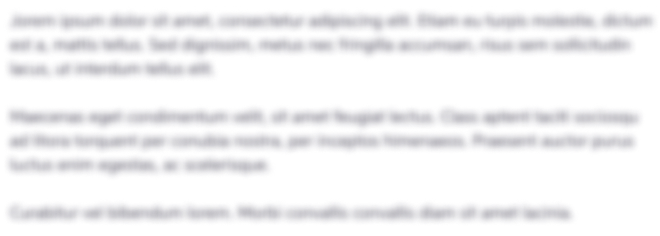
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started