Question
Complete the C++ skeleton program you have been given. The skeleton contains the function declarations, a driver, and empty function definitions. The program currently runs.
Complete the C++ skeleton program you have been given. The skeleton contains the function declarations, a driver, and empty function definitions. The program currently runs. However, there are several modification you should make for the program to be complete. You will need to implement the empty functions AND make calls to these functions in the main function.
Below is a list of the functions you will need to implement:
void RepeatDisplay(string st, int num);
int SumDigits(int num);
string RepeatItem (string st, int num);
void StringToFile (string filename, string stuff);
void PrintTriangle(int dimension);
int CountVowels (string st);
Further descriptions of these functions can be found in the in the provided source code file:
#include
#include
using namespace std;
//constants
const string STARS = "********************************";
//********************************************************************
//Function Prototypes
//********************************************************************
void RepeatDisplay(string st, int num);
int SumDigits(int num);
string RepeatItem (string st, int num);
void StringToFile (string filename, string stuff);
void PrintTriangle(int dimension);
int CountVowels (string st);
int GetChoice();
//********************************************************************
//Driver Program
//********************************************************************
int main(){
int choice = GetChoice();
while(choice !=0){
system("cls");
cout << STARS << endl;
if(choice == 1){
string str;
cout << "Enter a string to be printed" << endl;
cin >> str;
int num;
cout << "How many times to print the string?" << endl;
cin >> num;
cout << "REPLACE this entire statement with a call to RepeatDisplay" << endl;
}
else if (choice == 2){
int num;
cout << "Enter an integer to compute the sum of the digits" << endl;
cin >> num;
cout << "The sum of the digits of " << num << " is ";
cout << "REPLACE this quote with a call to SumDigits" << endl;
}
else if (choice == 3){
string str;
cout << "Enter a string to be printed" << endl;
cin >> str;
int num;
cout << "How many times to concatenate the string?" << endl;
cin >> num;
cout << "REPLACE this quote statement with a call to RepeatItem" << endl;
}
else if (choice == 4){
string filename;
cout << "Enter a filename" << endl;
cin >> filename;
string str;
cout << "Enter a string to be printed to the file" << endl;
cin >> str;
cout << "REPLACE this stmt with a call to StringToFile" << endl;
cout << str << " has been written to the file " << filename << endl;
}
else if (choice == 5){
int row, col;
cout << "Enter the dimension that will represent row and column" << endl;
cin >> row;
col = row;
cout << "REPLACE this entire stmt with a call to PrintTriangle" << endl;
}
else if (choice == 6){
string str;
cout << "Enter a string" << endl;
cin >> str;
cout << "The number of vowels in " << str << " is " ;
cout << "REPLACE this quote with a call to CountVowels" << endl;
}
else
cout << "INVALID CHOICE" << endl;
cout << STARS << endl;
choice = GetChoice(); //call to get next choice
system("cls");
} // end while loop
return 0;
}
//**********************************************************
//Function: RepeatDisplay
//Purpose: This function should print the given string
// num times
//Incoming: name contains the string to be printed
// the number of times the given string will be printed
//Outgoing: N/A
//Return: N/A
//**********************************************************
void RepeatDisplay(string st, int num){
//PUT YOUR CODE HERE
}
//**********************************************************
//Function: SumDigits
//Purpose: This function takes in an integer and returns
// the number sum of its digits
//Incoming: num contains the number
//Outgoing: N/A
//Return: Returns the sum of the digits
//**********************************************************
int SumDigits(int num){
int result = 0;
//PUT YOUR CODE HERE
return result;
}
//**********************************************************
//Function: RepeatItem
//Purpose: This function concatenates the 1st parameter to
// an empty string num times, where num is the
// 2nd parameter. Return the new string
//Incoming: str contains the original string
// num contains the number of times str should
// be concatenated to the temporary string
//Outgoing: N/A
//Return: The new string
//**********************************************************
string RepeatItem (string st, int num){
string result = "";
//PUT YOUR CODE HERE
return result;
}
//**********************************************************
//Function: StringToFile
//Purpose: This function will open an output file stream
// using the given string (1st parameter). If
// a successful open, the 2nd parameter will be
// printed to the file. If the file does fails
// to open, and error message should be printed
// to the screen.
//Incoming: filename will hold the name of the file to be
// opened for writing
// stuff will hold the string to be written to the
// file
//Outgoing: N/A
//Return: N/A
//**********************************************************
void StringToFile (string filename, string stuff){
//PUT YOUR CODE HERE
}
//**********************************************************
//Function: PrintTriangle
//Purpose: This function prints a triangle in the form
// X
// XX
// XXX
// XXXX
// based upon the dimension given
//Incoming: row and column dimension (will be same, so one parameter is sent
//Outgoing: N/A
//Return: N/A
//**********************************************************
void PrintTriangle(int dim){
//PUT YOUR CODE HERE
}
//**********************************************************
//Function: CountVowels
//Purpose: This function returns the number of vowels
// found in the given string parameter
//Incoming: given string
//Outgoing: N/A
//Return: number of vowels found in the string
//**********************************************************
int CountVowels (string st){
int count=0;
//PUT YOUR CODE HERE
return count;
}
//**********************************************************
//Function: GetChoice
//Purpose: This function prompts the user for their choice
//Incoming: N/A
//Outgoing: N/A
//Return: Menu choice
//**********************************************************
int GetChoice(){
cout << endl << STARS << endl;
int option;
cout << "1. Repeat Display" << endl;
cout << "2. Sum Digits" << endl;
cout << "3. Repeat Item" << endl;
cout << "4. Write to a File" << endl;
cout << "5. Print Triangle" << endl;
cout << "6. Count Vowels" << endl;
cout << "0. Quit" << endl;
cout << STARS<< endl << endl;
cout << "Enter your choice: ";
cin >> option;
return option;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
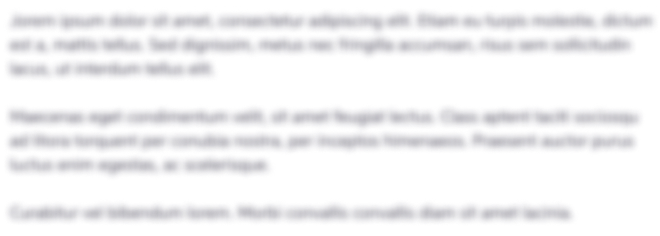
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started