Question
///// Complete the Deck, Hand and CrazyEights Classes. Complete the provided Deck class. The class has two constructors: public Deck() // purpose: creates a deck
///// Complete the Deck, Hand and CrazyEights Classes.
Complete the provided Deck class. The class has two constructors:
public Deck() // purpose: creates a deck of 52 standard cards // (one card for each rank/suit combination; no Jokers)
public Deck(int num_jokers) // purpose: creates a deck of (52 + num_jokers) cards
// consisting of the 52 standard cards
// plus num_joker Joker cards
You will also complete the following public methods:
public ListgetCards(int num_cards){return null;} // purpose: remove and return num_cards cards from this deck
// removes the first num_cards from the deck
public Card getCard(){return null;} // purpose: remove and return a single card from this deck // removes the first card from the deck
public void addCard(Card c){} // purpose: adds the card c to the back of the deck
Update: provided Deck class updated to match this specification.
Your must use encapsulation for this clas! You can add any private/protected attributes and helper methods that you need. You should not have any static attributes or methods (except possibly amain method that you use for testing the class).
Note: You can use any concrete List that Javas Collection Framework (JCF) provides.
Now complete the Hand class that is provided. You need to implement two methods (remove and add). You do not need to add very much code to this class.
Now finally,
consider the provided abstract Player class. You will create a new class called CrazyEightsPlayer that is a subclass the Player class.
The crazy eights player class simulates a valid player of the game crazy eights. The rules forour version of Crazy Eights is as follows:
-The game starts with each player being given some number of cards from the deck.
-A single card is taken from the deck and placed on top of the discard pile (and is visible to the player).
-A players turn consists of taking zero or more cards from the deck (adding them to their hand) and then playing a card on the top of the discard pile (removing it from their hand).
-A player can always play a card from their hand that has the same rank as the top card in
the discard pile.
-A player can always play a card from their hand that has the same suit as the top card in the discard pile.
-A player can always play a card with rank 8. When a player plays an 8, they are allowed to change the suit of the card to any of the four suits of their choosing. (You will do this by removing the eight from your hand and then returning a new Card object with the desired suit.)
-A player is allowed to (repeatedly) take a card from the deck before playing a card to the discard pile. You must play a card if you are able to. If the deck runs out of cards, the game ends.
-The player that discards all their cards first is the winner. If the deck is exhausted before any player can play all their cards then there is no winner.
Note: You are NOT implementing a crazy eights game. You are building the classes that would be needed for such a game. You are building the model for the game.
ALL JAVA CLASSES YOU NEED:
CARD
public abstract class Card implements Comparable{ /* handy arrays for ranks and suits */ /* do NOT change these */ public final static String[] RANKS = { "None", "Joker", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King", "Ace"}; public final static String[] SUITS = { "Diamonds", "Clubs", "Hearts", "Spades", "NONE"}; protected int rank; protected int suit; /** creates a card with specified suit and rank * * @param suit is the suit of the card (must be a string from Card.SUITS) * @param rank is the rank of the card (must be a string from Card.RANKS) */ public Card(String suit, String rank){ // add code here if needed boolean st = true; for(int i = 0;i RANKS.length;i++){ if(rank.equals(Card.RANKS[i])){ if(i == 0 || i == 1){ this.rank = i; this.suit = 4; st = false; break; }else{ this.rank = i; break; } } } if(st){ for(int i = 0;i SUITS.length;i++){ if(suit.equals(Card.SUITS[i])){ if(i == 4){ this.rank = 0; this.suit = 4; break; }else{ this.suit = i; break; } } } } } /** the numerical representation of the rank of the current card * * ranks have the numerical values * Joker = 1, * 2 = 2, 3 = 3, ..., 10 = 10 * Jack = 11, Queen = 12, King = 13, Ace = 14 * * @return the numerical rank of this card */ public abstract int getRank(); /** the string representation of the rank of the current card * * @return the string representation of the rank of this card (must be a string from Card.RANKS) */ public abstract String getRankString(); /** the suit of the current card * * @return the suit of this card (must be a string from Card.SUITS) */ public abstract String getSuit(); @Override public final String toString(){ // outputs a string representation of a card object int r = getRank(); if( r >= 2 && r <= 14 ){ return r + getSuit().substring(0,1); } if (r == 1){ return "J"; } return "invalid card"; } }
STANDARD CARD
public class StandardCard extends Card{ public StandardCard(String rank,String suit){ super(suit,rank); }
public StandardCard(int rank,String suit){ super(suit,Card.RANKS[rank]); }
public int getRank(){ return rank; }
public String getRankString(){ return Card.RANKS[rank]; }
public String getSuit(){ return Card.SUITS[suit]; }
public int compareTo(Card card){ if(card instanceof StandardCard){ StandardCard c = (StandardCard)card; if(c.getRank() == 1 && getRank() == 1){ return 0; } if(c.getRank() == 1 && getRank() != 1){ return -1; } if(getRank() == 1 && c.getRank() != 1){ return 1; } int s = 0; for(int i = 0;i
DECK
import java.util.List;
/* finish all of the constructors and methods given here */ /* do NOT add any public attributes or methods */ /* add any protected/private attributes you need */ public class Deck{ public Deck(){} public Deck(int nun_jokers){} public List
HAND
import java.util.List;
public class Hand{ protected List
PLAYER
public abstract class Player{ protected Hand hand; public Player(Hand hand){ this.hand = hand; } /* play a card from the player's hand */ /* returns null if they cannot play a card and the deck becomes empty */ /* do NOT return null unless you cannot play a card and the deck is empty */ public abstract Card play(Card top_of_discard_pile, Deck deck); public final int cardsLeft(){ return this.hand.numberOfCards(); } }
Thanks :)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
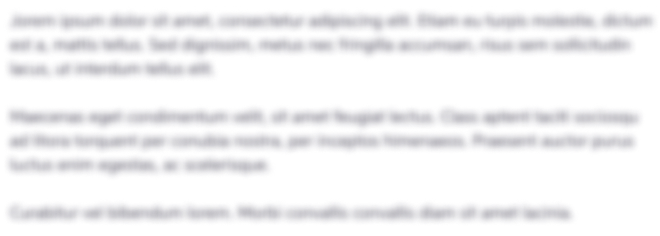
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started