Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Complete the Java code in Devoir2.java to get the following output: --------------------------Rectangle----------------------- --- I am the constructor of RegularGeometricShape without parameters I am the constructor
Complete the Java code in Devoir2.java to get the following output: --------------------------Rectangle----------------------- --- I am the constructor of RegularGeometricShape without parameters I am the constructor of Quadrilateral with five parameters Rectangle dimension number: 4 Rectangle Length = 2.0 Rectangle Width = 1.0 The center of the Rectangle = (1.00,0.50) Area of Rectangle = 2.0 Perimeter of Rectangle = 6.0 The points p1(0.00,1.00), p2(2.00,1.00), p3(2.00,0.00), p4(0.00,0.00) form a rectangle ------------------ -------------------------------------------- ------- -------------------Square------------------------------ I am the constructor of RegularGeometricShape without parameters I am the constructor of Quadrilateral with five parameters Number of square odds: 4 Square Length = 1.0 The center of the Square = (0.50,0.50) The points p1, p2, p3, p4 form a square -------------------------------------------------- ----------- --------------------------Circle------------ ----------------- I am the constructor of RegularGeometricShape without parameters Circle rating number: 0 The center of the Circle = (1.00,2.00) The area of the Circle = 3.14 The perimeter of the Circle = 6.28 -------------------------------------------------- ----------- --------------------------Triangle------------ --------------- I am the constructor of RegularGeometricShape without parameters Triangle rating number: 3 The center of the Triangle = (0.33,0.33) The perimeter of the Triangle = 3.414213562373095 The area of the Triangle = 0.5 Isosceles triangle The triangle is not equilateral Rectangle triangle
Here is devoir2.java:
import java.lang.Math; class Point { Point() { this.x = 0; this.y = 0; } Dot(double x, double y) { } public double distance(Dot p){ } public double getX() { } public double getY() { } public void setX(double x) { } public void setY(double y) { } public String toString() { return "(" + String.format("%.2f",this.x) + "," + String.format("%.2f",this.y) + ")"; } }
interface Form { public Point getCenter(); public int getNumberOdds(); public Point[] getVertices(); public double perimeter(); public double surface(); public String formName(); public void draw(); //optional } abstract class RegularGeometricShape implements Shape{ RegularGeometricShape() { System.out.println("I am the constructor of RegularGeometricShape with no parameters"); } public Point getCenter(){ } public int getNumberOdds() { } public Point[] getVertices() { } public static void displayFormName() { } public String shapeName(){ } }
abstract class Quadrilateral extends RegularGeometricShape{ Quadrilateral() { System.out.println("I'm the constructor of Quadrilateral with no parameters"); center = new Point(0,0); } Quadrilateral(Point p1, Point p2, Point p3, Point p4, Center point) { System.out.println("I am the constructor of Quadrilateral with five parameters"); } public String shapeName(){ //Overriding method } public int getNumberOdds() { } public boolean isRectangle() { //Just check that if P1P2 == P3P4 and P1P4 == P2P3 } public boolean isSquare() { //If rectangle and P1P2 == P2P3 } } class Rectangle extends Quadrilateral { public static void displayFormName() { //Overriding method } public String shapeName(){ } private static Point calculateCenter(Point p1, Point p2, Point p3, Point p4) { } Rectangle(Point p1, Point p2, Point p3, Point p4) { } public double scope() { } public double surface() { } public double getLength() { } public double getWidth() { } public void draw(){ //optional } } class Square extends Rectangle{ public String shapeName(){ //Overriding method } Square (Dot p1, Dot p2, Dot p3, Dot p4) { } Square (Dot p1, Dot p3) { //optional } } class Circle extends RegularGeometricShape{ public String shapeName(){ //Overriding method } Circle (Center point, double radius) { } public int getNumberOdds() { } public double scope() { } public double surface() { } public void draw() { //Optional } } class Triangle extends RegularGeometricShape{ public static void displayFormName() { //Overriding method } public String shapeName(){ } private static Point calculateCenter(Point p1, Point p2, Point p3) { } Triangle (Point p1, Point p2, Point p3) { } public int getNumberOdds() { } public double scope() { } public double surface() { } public void draw(){ //Optional } public boolean isRectangular() { } public boolean isIsocele() { } public boolean isEquilateral() { } }
public class Devoir2 { public static void main(String[] args) { //Rectangle System.out.println("-------------------------Rectangle---------------- ----------"); Rectangle rect1 = new Rectangle(new Point(0,1),new Point(2,1), new Point(2,0),new Point(0,0)); System.out.println("Number of dimension of " + rect1.NameOfTheForm() + ": " + rect1.getNumberDimensions()); System.out.println("Length of " + rect1.shapeName() + " = " + rect1.getLength()); System.out.println("Width of " + rect1.shapeName() + " = " + rect1.getWidth()); System.out.println("The center of " + rect1.shapeName() + " = " + rect1.getCenter()); System.out.println("The surface of the " + rect1.shapeName() + " = " + rect1.surface()); System.out.println("The perimeter of the " + rect1.shapeName() + " = " + rect1.perimeter()); Point[] vertices = rect1.getVertices(); if (rect1.isRectangle()) { System.out.println("The points p1" + vertices[0] + ", p2" + vertices[1] + ", p3" + vertices[2] + ", p4" + vertices[3] + " form a rectangle"); } else { System.out.println("The points p1" + vertices[0] + ", p2" + vertices[1] + ", p3" + vertices[2] + ", p4" + vertices[3] + " do not form not a rectangle"); } System.out.println("---------------------------------------------------------- ------------------"); System.out.println("--------------------------Square---------------- --------------");
//Square Square c1 = new Square(new Point(0,1), new Point(1,1), new Point(1,0), new Point(0,0)); System.out.println("Number of dimension of the " + c1.nameOfTheForm() + ": " + c1.nber_dimensions); System.out.println("Length of " + c1.shapeName() + " = " + c1.getLength()); System.out.println("The center of " + c1.shapeName() + " = " + c1.getCenter()); if (c1.isSquare()) { System.out.println("The points p1, p2, p3, p4 form a square"); } else { System.out.println("Points p1, p2, p3, p4 do not form a square"); } System.out.println("---------------------------------------------------------- ------------------"); System.out.println("-------------------------Circle---------------- -------------"); //Circle Circle circle1 = new Circle(new Point(1,2) , 1); System.out.println("Number of dimension of " + circle1.shapeName() + ": " + circle1.getNumberDimensions()); System.out.println("The center of " + circle1.shapeName() + " = " + circle1.getCenter()); System.out.println("The surface of " + circle1.shapeName() + " = " + String.format("%.2f",circle1.surface())); System.out.println("The perimeter of " + circle1.shapeName() + " = " + String.format("%.2f",circle1.perimeter())); System.out.println("---------------------------------------------------------- ------------------"); System.out.println("-------------------------Triangle---------------- -----------"); //Triangle Triangle triangle1 = new Triangle(new Point(0,0),new Point(1,0), new Point(0,1)); System.out.println("Number of dimension of " + triangle1.NameOfTheShape() + ": " + triangle1.getNumberDimensions()); System.out.println("The center of " + triangle1.shapeName() + " = " + triangle1.getCenter()); System.out.println("The perimeter of the " + triangle1.shapeName() + " = " + triangle1.perimeter()); System.out.println("The surface of " + triangle1.shapeName() + " = " + triangle1.surface()); if (triangle1.isIsocele()) { System.out.println("Isosceles Triangle"); } else { System.out.println("The triangle is not Isoscele"); } if (triangle1.isEquilateral()) { System.out.println("Equilateral Triangle"); } else { System.out.println("The triangle is not Equilateral"); } if (triangle1.isRectangle()) { System.out.println("Triangle Rectangle"); } else { System.out.println("Triangle is not Rectangle"); } } }
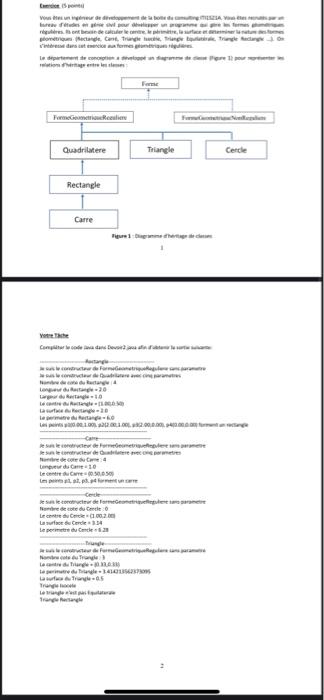
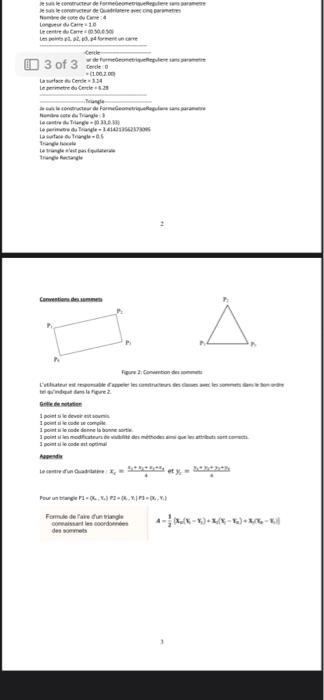
Translation:
You are a development engineer from the ITI1521A consulting box. You are recruited by a civil engineering design office to develop a program that manages regular geometric shapes. They need to calculate the center, perimeter, area and determine the nature of geometric shapes (Rectangle, Square, Isosceles Triangle, Equilateral Triangle, Rectangle Triangle...). In this exercise, we are interested in regular geometric shapes.
The design department developed a class diagram (Figure 1) to represent the inheritance relationships between classes:
Form
RegularGeometricShape
NonRegularGeometricShape
Quadrilateral
Triangle
Rectangle
Edge
Figure 1: Class inheritance diagram 1
Circle
Your Task
Complete the Java code in Devoir2.java to get the following output:
--------------------------Rectangle----------------------- ---
I am the constructor of RegularGeometricShape without parameters
I am the constructor of Quadrilateral with five parameters
Rectangle dimension number: 4
Rectangle Length = 2.0
Rectangle Width = 1.0
The center of the Rectangle = (1.00,0.50)
Area of Rectangle = 2.0
Perimeter of Rectangle = 6.0
The points p1(0.00,1.00), p2(2.00,1.00), p3(2.00,0.00), p4(0.00,0.00) form a rectangle ------------------ -------------------------------------------- ------- -------------------Edge------------------------------
I am the constructor of RegularGeometricShape without parameters
I am the constructor of Quadrilateral with five parameters
Number of square odds: 4
Edge Length = 1.0
The center of the Square = (0.50,0.50)
The points p1, p2, p3, p4 form a square
-------------------------------------------------- ----------- --------------------------Circle------------ -----------------
I am the constructor of RegularGeometricShape without parameters
Circle rating number: 0
The center of the Circle = (1.00,2.00)
The area of the Circle = 3.14
The perimeter of the Circle = 6.28
-------------------------------------------------- ----------- --------------------------Triangle------------ ---------------
I am the constructor of RegularGeometricShape without parameters
Triangle rating number: 3
The center of the Triangle = (0.33,0.33)
The perimeter of the Triangle = 3.414213562373095
The area of the Triangle = 0.5
Isosceles triangle
The triangle is not equilateral
Rectangle triangle
2
Summit conventions
P1
P4
P2
P2
P3 P1 Figure 2: Vertex convention
P3
The user is responsible for calling the class constructors with the vertices in the correct order as shown in Figure 2.
Scoring grid
1 point if the assignment is submitted.
1 point if the code compiles.
1 point if the code gives the correct output.
1 point if the visibility modifiers of the methods as well as the attributes are correct. 1 point if the code is optimal
appendix
The Center of a Quadrilateral: = 1+ 2+ 3+4 and = 1+ 2+ 3+4
import java.lang.Math;
class Point {
Point() {
this.x = 0;
this.y = 0;
}
Point(double x, double y) {
}
public double distance(Point p){
}
public double getX() {
}
public double getY() {
}
public void setX (double x) {
}
public void setY (double y) {
}
public String toString() {
return "(" + String.format("%.2f",this.x) + "," + String.format("%.2f",this.y) + ")";
}
}
interface Forme {
public Point getCentre();
public int getNombreCotes();
public Point [] getSommets();
public double perimetre();
public double surface();
public String nomDeLaForme();
public void dessiner(); //optionnel
}
abstract class FormeGeometriqueReguliere implements Forme{
FormeGeometriqueReguliere() {
System.out.println("Je suis le constructeur de FormeGeometriqueReguliere sans parametre");
}
public Point getCentre(){
}
public int getNombreCotes() {
}
public Point [] getSommets() {
}
public static void afficherNomDeLaForme() {
}
public String nomDeLaForme(){
}
}
abstract class Quadrilatere extends FormeGeometriqueReguliere{
Quadrilatere() {
System.out.println("Je suis le constructeur de Quadrilatere sans parametre");
centre = new Point(0,0);
}
Quadrilatere(Point p1, Point p2, Point p3, Point p4, Point centre) {
System.out.println("Je suis le constructeur de Quadrilatere avec cinq parametres");
}
public String nomDeLaForme(){ //Overriding method
}
public int getNombreCotes() {
}
public boolean isRectangle() {
//Il suffit de verifier que si P1P2 == P3P4 et P1P4 == P2P3
}
public boolean isCarre() {
//Si rectangle et P1P2 == P2P3
}
}
class Rectangle extends Quadrilatere{
public static void afficherNomDeLaForme() { //Overriding method
}
public String nomDeLaForme(){
}
private static Point calculerCentre(Point p1, Point p2, Point p3, Point p4) {
}
Rectangle (Point p1, Point p2, Point p3, Point p4) {
}
public double perimetre() {
}
public double surface() {
}
public double getLongueur() {
}
public double getLargeur() {
}
public void dessiner(){
//optionnel
}
}
class Carre extends Rectangle{
public String nomDeLaForme(){ //Overriding method
}
Carre (Point p1, Point p2, Point p3, Point p4) {
}
Carre (Point p1, Point p3) {
//optionnel
}
}
class Cercle extends FormeGeometriqueReguliere{
public String nomDeLaForme(){ //Overriding method
}
Cercle (Point centre, double rayon) {
}
public int getNombreCotes() {
}
public double perimetre() {
}
public double surface() {
}
public void dessiner() {
//Optionnel
}
}
class Triangle extends FormeGeometriqueReguliere{
public static void afficherNomDeLaForme() { //Overriding method
}
public String nomDeLaForme(){
}
private static Point calculerCentre(Point p1, Point p2, Point p3) {
}
Triangle (Point p1, Point p2, Point p3) {
}
public int getNombreCotes() {
}
public double perimetre() {
}
public double surface() {
}
public void dessiner(){
//Optionnel
}
public boolean isRectanle() {
}
public boolean isIsocele() {
}
public boolean isEquilaterale() {
}
}
public class Devoir2
{
public static void main(String[] args) {
//Rectangle
System.out.println("--------------------------Rectangle--------------------------");
Rectangle rect1 = new Rectangle(new Point(0,1),new Point(2,1), new Point(2,0),new Point(0,0));
System.out.println("Nombre de cote du " + rect1.nomDeLaForme() + " : " + rect1.getNombreCotes());
System.out.println("Longueur du " + rect1.nomDeLaForme() + " = " + rect1.getLongueur());
System.out.println("Largeur du " + rect1.nomDeLaForme() + " = " + rect1.getLargeur());
System.out.println("Le centre du " + rect1.nomDeLaForme() + " = " + rect1.getCentre());
System.out.println("La surface du " + rect1.nomDeLaForme() + " = " + rect1.surface());
System.out.println("Le perimetre du " + rect1.nomDeLaForme() + " = " + rect1.perimetre());
Point[] sommets = rect1.getSommets();
if (rect1.isRectangle()) {
System.out.println("Les points p1" + sommets[0] + ", p2" + sommets[1] + ", p3" + sommets[2] + ", p4" + sommets[3] + " forment un rectangle");
} else {
System.out.println("Les points p1" + sommets[0] + ", p2" + sommets[1] + ", p3" + sommets[2] + ", p4" + sommets[3] + " ne forment pas un rectangle");
}
System.out.println("-------------------------------------------------------------");
System.out.println("--------------------------Carre------------------------------");
//Carre
Carre c1 = new Carre(new Point(0,1), new Point(1,1), new Point(1,0), new Point(0,0));
System.out.println("Nombre de cote du " + c1.nomDeLaForme() + " : " + c1.nbre_cotes);
System.out.println("Longueur du " + c1.nomDeLaForme() + " = " + c1.getLongueur());
System.out.println("Le centre du " + c1.nomDeLaForme() + " = " + c1.getCentre());
if (c1.isCarre()) {
System.out.println("Les points p1, p2, p3, p4 forment un carre");
} else {
System.out.println("Les points p1, p2, p3, p4 ne forment pas un carre");
}
System.out.println("-------------------------------------------------------------");
System.out.println("--------------------------Cercle-----------------------------");
//Cercle
Cercle cercle1 = new Cercle(new Point(1,2) , 1);
System.out.println("Nombre de cote du " + cercle1.nomDeLaForme() + " : " + cercle1.getNombreCotes());
System.out.println("Le centre du " + cercle1.nomDeLaForme() + " = " + cercle1.getCentre());
System.out.println("La surface du " + cercle1.nomDeLaForme() + " = " + String.format("%.2f",cercle1.surface()));
System.out.println("Le perimetre du " + cercle1.nomDeLaForme() + " = " + String.format("%.2f",cercle1.perimetre()));
System.out.println("-------------------------------------------------------------");
System.out.println("--------------------------Triangle---------------------------");
//Triangle
Triangle triangle1 = new Triangle(new Point(0,0),new Point(1,0), new Point(0,1));
System.out.println("Nombre cote du " + triangle1.nomDeLaForme() + " : " + triangle1.getNombreCotes());
System.out.println("Le centre du " + triangle1.nomDeLaForme() + " = " + triangle1.getCentre());
System.out.println("Le perimetre du " + triangle1.nomDeLaForme() + " = " + triangle1.perimetre());
System.out.println("La surface du " + triangle1.nomDeLaForme() + " = " + triangle1.surface());
if (triangle1.isIsocele()) {
System.out.println("Triangle Isocele");
} else {
System.out.println("Le triangle n'est pas Isocele");
}
if (triangle1.isEquilaterale()) {
System.out.println("Triangle Equilaterale");
} else {
System.out.println("Le triangle n'est pas Equilaterale");
}
if (triangle1.isRectangle()) {
System.out.println("Triangle Rectangle");
} else {
System.out.println("Le triangle n'est pas Rectangle");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
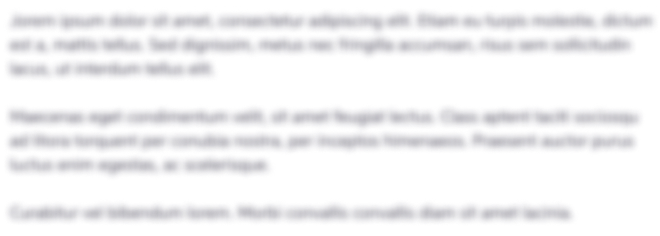
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started