Question
Complete the merge(B1DoubleLL obj) method Modify the betterInsert(E val, int index) method Modify the betterDelete(int index) method Modify the betterGet(int index) method Implement opCounts across
Complete the merge(B1DoubleLL
Grading: Implement OpCounts across all methods Note: Variable already exists, as does the getter and reset methods public class B1DoubleLL
private Node start, end; private int count; private long opCount = 0; public B1DoubleLL() { start = end = null; count = 0; } public long getOpCount() { return opCount; } public void resetOpCount() { opCount = 0; } public String printList() { String output = ""; Node current = start; while(current != null) { output += current.value + ","; current = current.next; } return output; } public String printListRev() { String output = ""; Node current = end; while(current != null) { output += current.value + ","; current = current.prev; } return output; } public void add(E val) { Node newItem = new Node(val); if(start == null) { start = newItem; end = start; count = 1; } else { end.next = newItem; newItem.prev = end; end = newItem; count++; } } public void insert(E val, int index) { if(index < 0) {//fix invalid index index = 0; } if(index >= count) {//goes in last position this.add(val); } else { Node newItem = new Node(val); if(index == 0) {//goes in first position newItem.next = start; start.prev = newItem; start = newItem; } else {//goes in middle Node current = start; for(int i = 1; i < index; i++) { current = current.next; } newItem.next = current.next; newItem.prev = current; current.next.prev = newItem; current.next = newItem; } count++; } } public void delete(int index) { if(index >= 0 && index < count) {//valid index if(index == 0) {//remove first start = start.next; if(start != null) {//as long as there was an item next in list start.prev = null; } else {//if only item was removed end = null; } } else if(index == count-1) {//remove last item end = end.prev; end.next = null; } else {//remove middle item Node current = start; for(int i = 1; i < index; i++) { current = current.next; } current.next = current.next.next; current.next.prev = current; } count--; } } public E get(int index) { if(index >= 0 && index < count) {//valid index Node current = start; for(int i = 0; i < index; i++) { current = current.next; } return current.value; } return null; } public int size() { return count; } public String toString() { return this.printList(); } private class Node { E value; Node next, prev; public Node(E v) { value = v; next = prev = null; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
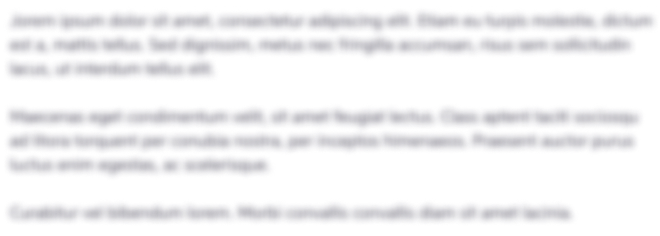
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started